Meritshot Tutorials
- Home
- »
- Understanding Variables in Python
Python Tutorial
-
Understanding Variables in PythonUnderstanding Variables in Python
-
Types Of Operators in PythonTypes Of Operators in Python
-
How to create string ?How to create string ?
-
Data Structure in PythonData Structure in Python
-
What is a Function in PythonWhat is a Function in Python
-
Parameters and Arguments in PythonParameters and Arguments in Python
-
What is a Lambda FunctionWhat is a Lambda Function
-
What is a Regular Expression?What is a Regular Expression?
-
Introduction to Loops in PythonIntroduction to Loops in Python
-
If-else Statements in PythonIf-else Statements in Python
-
Break Statement in PythonBreak Statement in Python
-
OOPS in PythonOOPS in Python
-
Space and Time in PythonSpace and Time in Python
-
Data Type in PythonData Type in Python
-
Understanding Variables in PythonUnderstanding Variables in Python
-
Types Of Operators in PythonTypes Of Operators in Python
-
How to create string ?How to create string ?
-
Data Structure in PythonData Structure in Python
-
What is a Function in PythonWhat is a Function in Python
-
Parameters and Arguments in PythonParameters and Arguments in Python
-
What is a Lambda FunctionWhat is a Lambda Function
-
What is a Regular Expression?What is a Regular Expression?
-
Introduction to Loops in PythonIntroduction to Loops in Python
-
If-else Statements in PythonIf-else Statements in Python
-
Break Statement in PythonBreak Statement in Python
-
OOPS in PythonOOPS in Python
-
Space and Time in PythonSpace and Time in Python
-
Data Type in PythonData Type in Python
Understanding Variables in Python
Variables are one of the very basic concepts when one is getting started with programming. You can basically think of a variable as a container that holds data that you can use and manipulate within your program. Variables in Python are very flexible to work with and really easy, therefore, making them is very good for any beginner.
What is a Variable?
Basically, a variable in Python is nothing but a name given to a value. This can eventually be utilized within the code snippet to reference that value. Variables help in storing and manipulating data; therefore, they enable your code to perform different types of operations.
For example, if you would like to store the number of toys you have, you may want to create a variable called toys and you might give it a value:
toys = 6
Here, toys is the variable name, and 6 is the value stored in that variable.
3 How to Declare a Variable in Python
Declaring a Variable in Python is very simple: a variable in Python is declared by writing the name
of this variable, using the assignment operator – ‘=’, and then assigning a value.
* variable_name = value Here’s an example:
Example:
# A variable to store a name
name = “Bhuvan Bam”
# A variable to store integer number
age = 30
# A variable to store decimal number
height = 5.8
# A variable to save the current status of the person
is_student = False
In the example above:
‘name’ is a variable that holds the string “Bhuvan Bam”.
‘age’ is a variable that holds the integer 30.
‘height’ is a variable that holds the float 5.8
Each variable name is unique and refers to the data you’ve assigned to it.
4 Changing the Value of a Variable in Python
You can easily change the value of a variable. It totally depends on you what you want to store in
a variable. Just how you decide which toy to put in which box
company_name = “Meritshot”
print(company_name)
# Assigning a new value to company name
company_name = “Meritshot Zetta”
print(company_name)
Meritshot
Meritshot Zetta
5 Assigning multiple values to multiple variables
Now if you want to assign values to multiple variables, assigning then again and again seems a tiring job, so we have a shortcut here.
# we declare the variable again and again, note we are
a = 5
b = 6
c = 10
d = 15
# we are assigning all the variables together
a,b,c,d = 5,6,10,15
The task of assigning multiple values becomes so easier now right?
6 Assigning one value to multiple variables
We can also assign one value to multiple variables at once instead of repeatedly assigning them
again and again
a = b = c = “Dyuti”
print(a)
print(b)
print(c)
Dyuti
Dyuti
Dyuti
6.0.1 Variables do not need to be declared with any particular type and can even
change the type after they have been set.
now let’s understand this statement. Suppose you declare a variable ‘a’ and then assign ‘6’ to the variable. Later on you decide to change the value assigned to ‘a’. You can do that easily by reassigning the value to ‘a’. Look at the example below.
a = b = c = “Dyuti”
print(a)
print(b)
print(c)
6
Shweta
a = Shweta
print(a)
————————————————————————— NameError Traceback (most recent call last) Input In [9], in () —-> 1 a = Shweta 2 print(a) NameError: name ‘Shweta’ is not defined
We encounter NameError because Shweta is string and strings should be enclosed in single or double
quotes
a = ‘Shweta’
print(a)
a = “Shweta”
print(a)
Shweta
Shweta
Enclosing strings in Single quote ” or double quote ”” makes no difference. They give you same result
6.0.2 NOTE:
- The = symbol is known as the assignment This is not the same as an equals symbol.
- The value on the right of the = gets stored in the variable on the So ‘Bhuvan’ = name will not work. The error that will appear is “SyntaxError: can’t assign to literal”
- We cannot use spaces in a variable For example, player name = ‘Bhuvan’ will not work. The error that will appear is “SyntaxError: invalid syntax”
- Python doesn’t have For constants, simply make a variable and don’t change it!
7 Variable Naming Conventions.
Naming conventions are a set of guidelines for choosing names for variables, functions, classes, and other identifiers in your code. Consistent naming is crucial which makes your code more readable, maintainable, and easier to understand for both you and others. Now imagine if you name a variable as “fruits” but you wanted to store a chocolate’s name, For you it is easy to understand that whenever you are referring to “fruits”, it is actually chocolate you’re refering to, but some other person can’t understand that in your code “fruits” refer to chocolate. This will create confusion, That is why all over the world people decided on some rules to abide by while using variables. There are some common styles that you can use while naming the variables. ## Common Styles:
CamelCase: Lowercase first word, then capitalize the first letter of each subsequent word (e.g., firstName, totalAmount).
myVariableName = “Dyuti” print(myVariableName)
Dyuti
PascalCase: Capitalize the first letter of each word (e.g., FirstName, TotalAmount).
MyVariableName = “Dyuti” print(MyVariableName)
Dyuti
Snake Case: All lowercase, words separated by underscores (e.g., first_name, total_amount).
my_variable_name = “Dyuti” print(my_variable_name)
Dyuti
- Kebab Case: All lowercase, words separated by Often used in file and directory names.
(e.g., first-name, total-price)
Input In [14]
my-variable-name = “Dyuti”
^
SyntaxError: cannot assign to operator
We get syntax error because Python does not allow kebab case
8 Rules for variables:
- Start with a letter or underscore: Just like your parents wouldn’t name you “123”, don’t start a variable name with a
- Use letters, numbers, and underscores: You can use these characters to create your variable
- Case matters: “age” and “Age” are different
- Avoid special words: Don’t use words like “if”, “else”, or “for” as variable (These special words are called keywords)
- A variable name cannot start with a number
- A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and __)
1man = “superman” print(1man)
Input In [15]
1man = “superman”
^
SyntaxError: invalid syntax
We got SyntaxError because variables cannot start with 1
age = 19
Age = 20
print(age)
print(Age)
19
20
As you can see “age” and “Age” are different
for = “Cat”
print(for)
Input In [17] for = “Cat”
^
SyntaxError: invalid syntax
We get an error because “for” is a reserved keyword in python
wow! = “Interjection
print(wow!)
Input In [18]
wow! = “Interjection”
^
SyntaxError: invalid syntax
9 Best Practices:
- Descriptive names: Clearly indicate the variable’s purpose but you can also use short names (like x and y) (e.g., customer_age instead of age).
- Consistency: Use the same naming style throughout your
- Avoid reserved keywords: Don’t use keywords like if, else, for, as variable names.
- Be concise: While descriptive, avoid overly long
- Use meaningful prefixes or suffixes: For example, str_ for strings, int_ for integers, or obj_ for
10 How to delete variable:
Now since we are declaring and assigning variable, we should also be able to delete the variables
fruit = “pomegranate” print(fruit)
del fruit
# The variable gets deleted. Now if you try to print fruit again you will get␣
↪an error because it does not exist.
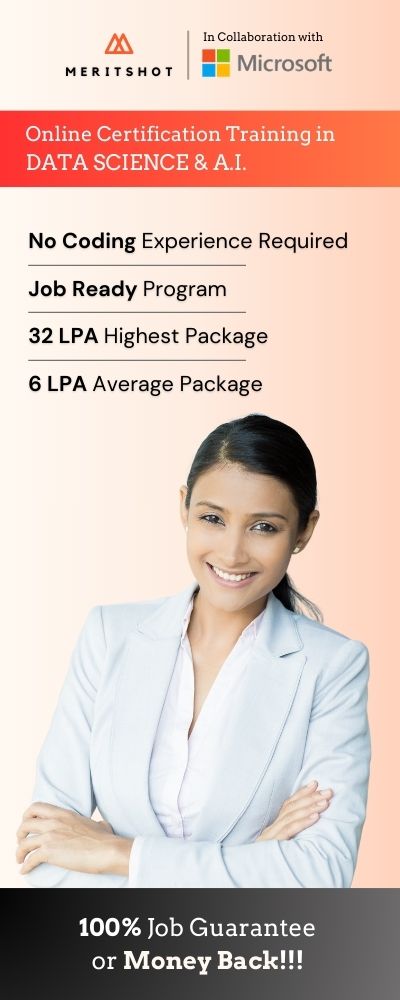