Meritshot Tutorials
- Home
- »
- If-else Statements in Python
Python Tutorial
-
Understanding Variables in PythonUnderstanding Variables in Python
-
Types Of Operators in PythonTypes Of Operators in Python
-
How to create string ?How to create string ?
-
Data Structure in PythonData Structure in Python
-
What is a Function in PythonWhat is a Function in Python
-
Parameters and Arguments in PythonParameters and Arguments in Python
-
What is a Lambda FunctionWhat is a Lambda Function
-
What is a Regular Expression?What is a Regular Expression?
-
Introduction to Loops in PythonIntroduction to Loops in Python
-
If-else Statements in PythonIf-else Statements in Python
-
Break Statement in PythonBreak Statement in Python
-
OOPS in PythonOOPS in Python
-
Space and Time in PythonSpace and Time in Python
-
Data Type in PythonData Type in Python
Conditionals
if-else Statements
Sometimes the programmer needs to check the evaluation of certain expression(s), whether the expression(s) evaluate to True or False. If the expression evaluates to False, then the program execution follows a different path than it would have if the expression had evaluated to True.
Based on this, the conditional statements are further classified into following types:
if
if-else
if-else-elif
nested if-else-elif.
## An if……else statement evaluates like this: ### 1. if the expression evaluates True: Execute the block of code inside if statement.
After execution return to the code out of the if……else block.\ ### 2. if the expression evaluates False:
Execute the block of code inside else statement.
After execution return to the code out of the if……else block.
applePrice = 210
budget = 200
if (applePrice <= budget):
print(“Alexa, add 1 kg Apples to the cart.”) else:
print(“Alexa, do not add Apples to the cart.”)
Alexa, do not add Apples to the cart.
elif Statements
Sometimes, the programmer may want to evaluate more than one condition, this can be done using an elif statement.
Working of an elif statement
Execute the block of code inside if statement if the initial expression evaluates to True. After execution return to the code out of the if block.
Execute the block of code inside the first elif statement if the expression inside it evaluates True. After execution return to the code out of the if block.
Execute the block of code inside the second elif statement if the expression inside it evaluates True. After execution return to the code out of the if block.
Execute the block of code inside the nth elif statement if the expression inside it evaluates True. After execution return to the code out of the if block.
Execute the block of code inside else statement if none of the expression evaluates to True. After execution return to the code out of the if block.
EXAMPLE:
num = 0
if (num < 0):
print(“Number is negative.”) elif (num == 0):
print(“Number is Zero.”) else:
print(“Number is positive.”)
Number is Zero.
Nested if statements
We can use if, if-else, elif statements inside other if statements as well.
Example:
num = 18
if (num < 0):
print(“Number is negative.”) elif (num > 0):
if (num <= 10):
print(“Number is between 1-10”) elif (num > 10 and num <= 20):
print(“Number is between 11-20”) else:
print(“Number is greater than 20”)
else:
print(“Number is zero”)
Number is between 11-20
If ... Else in One Line
There is also a shorthand syntax for the if-else statement that can be used when the condition being tested is simple and the code blocks to be executed are short. Here’s an
Example:
a = 2
b = 330
print(“A”) if a > b else print(“B”)
B
You can also have multiple else statements on the same line:
Example:
One line if else statement, with 3 conditions:
a = 330
b = 330
print(“A”) if a > b else print(“=”) if a == b else print(“B”)
Another Example:
result = value_if_true if condition else value_if_false
This syntax is equivalent to the following if-else statement:
if condition:
result = value_if_true else:
result = value_if_false
Conclusion
The shorthand syntax can be a convenient way to write simple if-else statements, especially when you want to assign a value to a variable based on a condition. However, it’s not suitable for more complex situations where you need to execute multiple statements or perform more complex logic. In those cases, it’s best to use the full if-else syntax.
Enumerate function in python
The enumerate function is a built-in function in Python that allows you to loop over a sequence (such as a list, tuple, or string) and get the index and value of each element in the sequence at the same time. Here’s a basic example of how it works:
# Loop over a list and print the index and value of each element
fruits = [‘apple’, ‘banana’, ‘mango’] for index, fruit in enumerate(fruits):
print(index, fruit)
- apple
- banana
- mango
As you can see, the enumerate function returns a tuple containing the index and value of each element in the sequence. You can use the for loop to unpack these tuples and assign them to variables, as shown in the example above.
Changing the start index
By default, the enumerate function starts the index at 0, but you can specify a different starting index by passing it as an argument to the enumerate function:
# Loop over a list and print the index (starting at 1) and value of each element
fruits = [‘apple’, ‘banana’, ‘mango’]
for index, fruit in enumerate(fruits, start=1):
print(index, fruit)
- apple
- banana
- mango
The enumerate function is often used when you need to loop over a sequence and perform some action with both the index and value of each element. For example, you might use it to loop over a list of strings and print the index and value of each string in a formatted way:
fruits = [‘apple’, ‘banana’, ‘mango’]
for index, fruit in enumerate(fruits):
print(f'{index+1}: {fruit}’)
1: apple
2: banana
3: mango
In addition to lists, you can use the enumerate function with any other sequence type in Python, such as tuples and strings. Here’s an example with a tuple:
# Loop over a tuple and print the index and value of each element
colors = (‘red’, ‘green’, ‘blue’)
for index, color in enumerate(colors): print(index, color)
- red
- green
- blue
And here’s an example with a string:
# Loop over a string and print the index and value of each character
s = ‘hello’
for index, c in enumerate(s): print(index, c)
- h
- e
- l
- l
- o
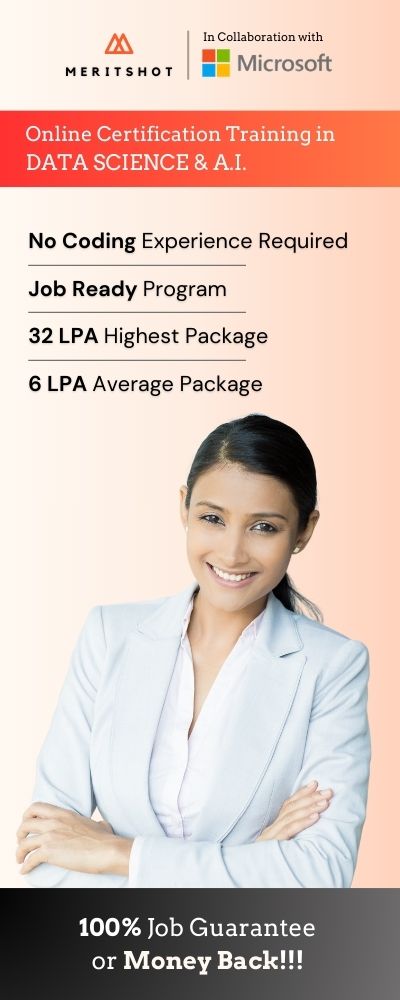