Meritshot Tutorials
- Home
- »
- Data Type in Python
Python Tutorial
-
Understanding Variables in PythonUnderstanding Variables in Python
-
Types Of Operators in PythonTypes Of Operators in Python
-
How to create string ?How to create string ?
-
Data Structure in PythonData Structure in Python
-
What is a Function in PythonWhat is a Function in Python
-
Parameters and Arguments in PythonParameters and Arguments in Python
-
What is a Lambda FunctionWhat is a Lambda Function
-
What is a Regular Expression?What is a Regular Expression?
-
Introduction to Loops in PythonIntroduction to Loops in Python
-
If-else Statements in PythonIf-else Statements in Python
-
Break Statement in PythonBreak Statement in Python
-
OOPS in PythonOOPS in Python
-
Space and Time in PythonSpace and Time in Python
-
Data Type in PythonData Type in Python
1 What is Data Type ?
A data type is like a label that tells the computer what kind of information you’re working with. When you create a variable in programming, you’re essentially telling the computer, “Hey, this is going to hold a certain kind of data.”
Data types define what kind of values the variable can hold (like numbers, text, etc.), what you can do with that data (like adding, subtracting, or joining), and how the computer should store it. Think of it like sorting things into categories: numbers go in one box, words go in another, and so on. This way, the computer knows exactly how to handle and work with the data you’re giving it.
In simpler terms, data types help the computer understand and process the information you’re dealing with, ensuring everything runs smoothly and without errors.
2 Get the type:
We can also print the type of variable used:
a = 6
b = “superman” c = False
print(type(a)) #integer print(type(b)) #string print(type(c)) #boolean
<class ‘int’>
<class ‘str’>
<class ‘bool’>
We can see that when we mention the variable in the type() we get the data type of the variable. For now we saw three data type
Integer – Number datatype String – categorical datatype boolean – True/False(0/1)
Python is dynamically typed, the data type of a variable is determined at runtime based on the assigned value.
For example – In any other programming language suppose java we mention the data type before the variable.
string name – “Shweta”
3 Types of data in data types:
For now we will just know the types of data that are available, Don’t worry if you don’t understand some things. We will dive deep into each one of them in detail later. ## 1. Numeric Data Types Integers and floating points are separated by the presence or absence of a decimal point. For instance,
- is an integer
- is a floating-point * Int – for Integer Number Example :-
#Integer Variable
num1 = 5
Float – for Decimal Number
Example:
#Float Variable
num2 = 5.5
Complex – for Complex Number
Example:
num3 = 3 + 4j
3.1 2. String Data Types
In Python, a string is simply a collection of characters that represent text. You can think of it as a piece of text that can include anything from letters and numbers to symbols and spaces. Strings are one of the most commonly used data types in programming because they let you work with text in all sorts of ways. We will learn more about string later in detail.
Example :-
company_name =”Meritshot”
3.2 3. Sequence Data Types
In Python, a sequence is a way to store an ordered collection of elements, where the order really matters. You can access each element by its position, or index, in the sequence. Python provides several built-in sequence types that help you organize and work with collections of data easily and efficiently. * List
Example :-
fruits = [‘apple’,’banana’,’pomegranate’]
Tuple
Example :-
roll_no = (121,122,123,124,125,126,127,128,129,130)
Range
Example :-
for i in range(5):
print(i)
0
1
2
3
4
3.3 4. Binary Data Types
In Python, a binary data type represents information using a series of 0s and 1s—just like how computers handle data at a fundamental level. Think of it as the special “language” that computers use to efficiently store and process information. This binary data format is particularly useful when working with files, images, or any data that can be broken down into just two possible values. Instead of using regular numbers or letters, binary data uses combinations of 0s and 1s to represent everything from simple text to complex images. * bytes
Example :-
b1 = bytes([65, 66, 67, 68, 69])
print(b1)
b’ABCDE’
bytearray
Example :-
value = bytearray([72, 101, 108, 108, 111]) print(value)
bytearray(b’Hello’)
Now you might be wondering that bytes and bytearray have same example then where is the difference. Well you’re right they both are almost same except one thing that bytearray is mutable whereas bytes is not !!!
memoryview
Example :-
data = bytearray(b’Hello, world!’)
view = memoryview(data)
print(view)
<memory at 0x000001D9B6204880>
3.4 5. Dictionary Data Type
Python dictionaries are like special storage containers that use a hash table to organize data. You can think of them as a collection of key-value pairs.Python dictionaries are like special storage containers that use a hash table to organize data. You can think of them as a collection of key- value pairs
Example :-
dict = {121:’Moon’, 122:’Sia’, 123:’Diya’} print(dict)
{121: ‘Moon’, 122: ‘Sia’, 123: ‘Diya’}
3.5 6. Set Data Type
Set is a Python implementation of set as defined in Mathematics. A set in Python is a collection, but is not an indexed or ordered collection as string, list or tuple. An object cannot appear more than once in a set, whereas in List and Tuple, same object can appear more than once. Comma separated items in a set are put inside curly brackets or braces {}. Items in the set collection can be of different data types. * Set
Example :-
set1 = {‘pink’,’blue’,’red’,’yellow’,’green’}
set2 = {1,2,3,4,5}
print(set1)
print(set2)
{‘yellow’, ‘pink’, ‘red’, ‘green’, ‘blue’}
{1, 2, 3, 4, 5}
3.6 7. Boolean Data Type
The Boolean type in Python is a built-in data type that represents one of two values: True or False. It’s like a simple on/off switch in programming. The bool() function in Python lets you check the truth value of any expression, returning True or False based on the outcome. A Boolean value can only be True or False, which are actually just special versions of the numbers 1 and 0 in the background.
Example :-
a = True
print(a)
True
3.7 8. None Data Type
In Python, the None type is represented by the special type NoneType. It’s a unique object that signifies the absence of a value or a null value. When you see None in your code, it’s like Python’s way of saying, “There’s nothing here.”
Example :-
x = None
print(x)
None
4 Implicit Conversion (Coercion):
When Python automatically changes the type of your data during an operation, that’s called implicit conversion. It happens without you telling it to, making sure everything works smoothly without losing important information. For example, if you’re adding an integer to a float, Python will automatically turn the integer into a float so the math adds up correctly.
x = 5 # This is an integer variable
y = 5.5 # This is a float variable
result = x + y # Adding integer and float values
print(result) # Final Value converted to Float
10.5
So the above example is Implicit conversion, here Python converts the Integer variable to Float variable on its own without doing it explicitly.
x = 5 # Integer variable
y = “Ashish” # String Variable
result = x+y # Adding Integer and String Variable
print(result) # error generated
TypeError
Input In [17], in <cell line: 3>()
1 x = 5 # Integer variable
2 y = “Ashish” # String Variable
—-> 3 result = x+y # Adding Integer and String Variable
4 print(result)
TypeError: unsupported operand type(s) for +: ‘int’ and ‘str’
We got a TypeError in the above example because it was not implicit conversion. The above example comes under Explicit conversion which we will learn next.
Some of the coomon Implicit conversions are :
* Integer to Float * Integer to complex * Float to complex * Boolean to Integer
result = True + 1 #Adding boolean value and Integer Value
print(result)
As discussed above there are two types of boolean values, True/False, where ‘True’ stands for ‘1’ and ‘False’ standa for ‘0’
5 Type Casting (Type Conversion in Python)
We can easily convert from one type to another type as int(), float(), and complex() methods. Type casting is simply the process of converting one type of data into another. It’s like changing the form of a value so it can be used in a different way. For example, turning a number into a string so you can combine it with text.
num1 = 10
num_str = str(num1) num2 = 20
res = num_str + num2 print(res)
TypeError
Input In [19], in <cell line: 4>()
2 num_str = str(num1) 3 num2 = 20
—-> 4 res = num_str + num2
5 print(res)
TypeError: can only concatenate str (not “int”) to str
6 Obtaining User Input
6.1 input() function:
Takes a prompt as an optional argument and returns the user’s input as a string.
name = input(“Enter your name: “) print(“Hello, ” + name + “!”)
Enter your name: Aisha Hello, Aisha!
6.2 Converting Input Data Types:
int() function:
Converts a string to an integer.
age = int(input(“Enter your age: “))
print(“You will be”, age + 1, “next year.”)
Enter your age: 17
You will be 18 next year.
- float() function:
Converts a string to a floating-point number.
height = float(input(“Enter your height in centimeters: “))
print(“Your height in meters is:”, height / 100)
Enter your height in centimeters: 152.5 Your height in meters is: 1.525
Combining the three examples above we get:
name = input(“Enter your name: “) age = int(input(“Enter your age: “))
height = float(input(“Enter your height in centimeters: “))
print(f”Hello, {name}!”)
print(f”You will be {age + 1} next year.”) print(f”Your height in meters is: {height / 100}“)
Enter your name: Aisha
Enter your age: 17
Enter your height in centimeters:
152.5 Hello, Aisha!
You will be 18 next year.
Your height in meters is: 1.525
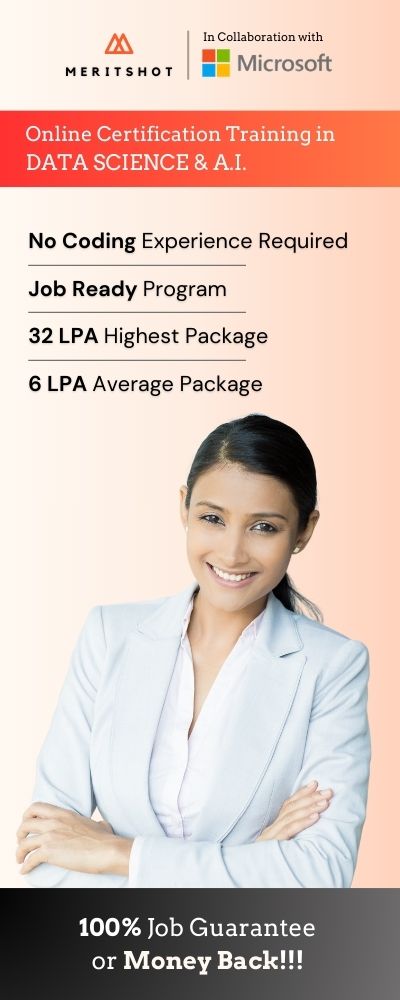