Meritshot Tutorials
- Home
- »
- Data structure in Python
Python Tutorial
-
Understanding Variables in PythonUnderstanding Variables in Python
-
Types Of Operators in PythonTypes Of Operators in Python
-
How to create string ?How to create string ?
-
Data Structure in PythonData Structure in Python
-
What is a Function in PythonWhat is a Function in Python
-
Parameters and Arguments in PythonParameters and Arguments in Python
-
What is a Lambda FunctionWhat is a Lambda Function
-
What is a Regular Expression?What is a Regular Expression?
-
Introduction to Loops in PythonIntroduction to Loops in Python
-
If-else Statements in PythonIf-else Statements in Python
-
Break Statement in PythonBreak Statement in Python
-
OOPS in PythonOOPS in Python
-
Space and Time in PythonSpace and Time in Python
-
Data Type in PythonData Type in Python
1 What is Data structure ?
Data structures in Python are ways to organize and store data. They provide a structure for data, making it easier to access, manipulate, and manage. Basically They help to keep data tidy and easy to find.
Think of Data structure as different ways to organize your toys. Let’s look into some Data Structures:
2 Python List :
2.1 What is a List?————-> The Ordered Box
A list is an ordered collection of items. Think of it as a shopping list where each item has a specific place. Lists are flexible, meaning you can change them after creation by adding, removing, or modifying items, where the order matters.
2.2 How to Create a List?
Creating a list is simple. Use square brackets [] to define your list and separate items with commas. Suppose Sneha want to keep the track of her daily tasks that she does after waking up in order, then she can use a list to do this task.
# List of daily tasks
tasks = [“Wake up”, “Brush teeth”, “Go for a jog”, “Breakfast”]
print(tasks)
[‘Wake up’, ‘Brush teeth’, ‘Go for a jog’, ‘Breakfast’]
2.3 Accessing List Items
You can access individual items in a list using an index, starting from 0.
Suppose Aryan wants to know what is the third task Sneha does on daily basis, so then instead of manually going through the list he can just directly access the third item on the list.
For now the list has only 4 tasks but imagine the list has 100 tasks and you want to know what is the 90th task a person does?
It will be very hectic for you to manually do that so we use access list item function
#Accessing the third item
print(tasks[2])
#Accessing the last item
print(tasks[-1])
Go for a jog
Breakfast
Negative indexing in Python allows you to access elements of a sequence, such as a list, tuple, or string, from the end rather than the beginning. It is very helpful when we don’t know how many elements are there in a list.
NOTE : Negative Indexing starts at -1 not 0
2.4 Modifying a List
Lists are mutable, meaning you can change their content.
Now suppose rainy season comes and it becomes very difficult for Sneha to go out for a job, so she decides to change her daily tasks. she decides to replace jogging with yoga. Now in this situation creating a whole new list seems like a pretty dumb idea. So here we use modify list function and replace jogging with yoga. We mention the index where we want to do modification and boom the work is done. So simple !!!!
tasks[2] = “Yoga”
print(tasks)
tasks[2] = “Yoga” print(tasks)
2.5 Appending a list
It allows to add elements to the end of an existing list.
Python provides the append() method specifically for this purpose.
Again let’s imagine that Sneha wants to add in her tasks that after breakfast “she starts her work”. Making the whole list again doesn’t seem like a practical idea. So we use append function
tasks.append(“Starts work”)
print(tasks)
[‘Wake up’, ‘Brush teeth’, ‘Yoga’, ‘Breakfast’, ‘Starts work’]
NOTE : Lists are mutable and String are Immutable.
As you can see everytime we made changes according to Sneha’s will the original list was changed.
3 Inserting in a list
Inserts an element at a specified position in the list.
|
Prevoiusly we saw the modification function that replaces value then we saw appending function that adds a value to the end of the list but what if Sneha wants to add a task after Breakfast. Imagine Sneha wants to add a task which requires for her to call her parents. We will use insert function for this task.
tasks.insert(4,”Call parents”)
print(tasks)
[‘Wake up’, ‘Brush teeth’, ‘Yoga’, ‘Breakfast’, ‘Call parents’, ‘Starts work’]
‘4’ refers to the index where you want to add the new task and ‘call parents’ is the task
3.1 Extending a list
adds all the elements of an iterable. Suppose you have two lists, L1 and L2 when we use extend function then all the elements of L2 will go in L1. Imagine Sneha wants to make a new list of her to_do tasks and then she decides to merge that to_do list with her taks list because all the work in her to_do list is laready completed.
#This is Sneha’s to_do list
to_do = [‘Dancing’,’Bath’,’Dinner’,’Read books’, ‘Sleep’]
#Sneha completed her to_do list now she wants to merge her to_do list with her␣
↪task list.
tasks.extend(to_do) print(tasks)
[‘Wake up’, ‘Brush teeth’, ‘Yoga’, ‘Breakfast’, ‘Call parents’, ‘Starts work’, ‘Dancing’, ‘Bath’, ‘Dinner’, ‘Read books’, ‘Sleep’]
3.2 Removing from a list
Removes the first occurrence of a specified element from the list.
Sneha by mistake appended “sleep” twice so she by using remove function deletes the first occurence of “sleep”.
Lets see how she does it.
# adding extra sleep in tasks tasks.append(“sleep”) print(tasks)
#removing the extra sleep tasks.remove(“sleep”) print(tasks)
[‘Wake up’, ‘Brush teeth’, ‘Yoga’, ‘Breakfast’, ‘Call parents’, ‘Starts work’, ‘Dancing’, ‘Bath’, ‘Dinner’, ‘Read books’, ‘Sleep’, ‘sleep’]
[‘Wake up’, ‘Brush teeth’, ‘Yoga’, ‘Breakfast’, ‘Call parents’, ‘Starts work’, ‘Dancing’, ‘Bath’, ‘Dinner’, ‘Read books’, ‘Sleep’]
See in first list there are “sleep” mentioned twice but in the next list sleep is only mentioned once.
4 Sorting a list
Sorts the list in ascending order (by default). We can also sort in descending order by using the reverse=True parameter.
my_list = [3, 1, 4, 2]
my_list.sort()
my_list = [3, 1, 4, 2] my_list.sort()
[1, 2, 3, 4]
Now Let’s arrange the list in descending order
my_list.sort(reverse = True)
print(my_list)
[4, 3, 2, 1]
5 Find Length of List
magine you guys have many elements, now you can’t count all the element manually right. If there are 999 elements then you need to count them 999 times. So we need a function for that work to be done.
length = len(tasks) print(length)
lengt = len(my_list) print(lengt)
11
4
6 What is Tuples? —————> The Locked Box
A tuple is like a list, but it’s immutable, meaning once you create it, you can’t change it. Tuples are great for storing data that shouldn’t be altered. For example: Student’s Roll number or tracking historical events.
6.1 How to Create a Tuple?
Use parentheses () to define a tuple.
Lets imagine that Ritu is given the task of recording all the historical events, so she decides to use a tuple to store all the details, as Tuples are immutable i.e. cannot be changed. She doesn’t want anyone to tamper with historical events.
Before we start solving Ritu’s problem let’s first learn how to create a simple tuple.
colors = (“red”,”white”,”yellow”,”pink”)
print(colors)
colors = (“red”,”white”,”yellow”,”pink”) print(colors)
6.2 Accessing Tuple Items
You can access individual items in a tuple using an index, starting from 0. We will also help Ritu in accessing historic events after a while.
print(colors[0])
print(colors[-1])
print(colors[-3]) #accessing colors[1]
red pink white
7 Differet Types of Tuple
7.1 Empty Tuple
Empty tuple is really useful. Suppose you don’t know now the values that you need to enter or suppose you want to take user input then reating empty tuple initially is very helpful
# create an empty tuple
empty_tuple = ()
print(empty_tuple)
()
7.2 Tuple of different data types
# tuple of string types
names = (‘Jeetu’, ‘Tingu’, ‘Chotu’) print (names)
# tuple of float types float_values = (1.2, 3.4, 2.1)
print(float_values)
(‘Jeetu’, ‘Tingu’, ‘Chotu’)
(1.2, 3.4, 2.1)
7.3 Tuple of mixed data types
# tuple including string and integer mixed_tuple = (2, ‘Hello’, ‘Chingu’) print(mixed_tuple)
#Chingu means friend in korean
(2, ‘Hello’, ‘Chingu’)
Now let’s solve Ritu’s problem: let us first make a tuple for her
#Here we have a tuple inside another tuple
historical_events = (
(“India’s Independence”, 1947),
(“India’s Nuclear Test”, 1998), (“COVID-19 Pandemic”, 2020),
(“Chandrayaan 3”, 2023)
)
print(historical_events)
(“India’s Nuclear Test”, 1998), (“COVID-19 Pandemic”, 2020),
(“Chandrayaan 3”, 2023)
)
print(historical_events)
#Here we have a tuple inside another tuple
historical_events = (
(“India’s Independence”, 1947),
(“India’s Nuclear Test”, 1998), (“COVID-19 Pandemic”, 2020),
(“Chandrayaan 3”, 2023)
)
print(historical_events)
7.4 Accessing recent historic event for Ritu:
recent_event = historical_events[-1] print(recent_event)
(‘Chandrayaan 3’, 2023)
7.5 Modifying Tuple values
Now imagine Ritu made a mistake and wants to change the date of Covid-19 to 2021 and tried to change it
historical_events[2][1] = 2021
print(historical_events)
TypeError
Input In [20], in <cell line: 1>()
—-> 1 historical_events[2][1] = 2021
2 print(historical_events)
Traceback (most recent call last)
TypeError: ‘tuple’ object does not support item assignment
Now you can see Ritu faced an TypeError while modifying the value, This proves that tuple values cannot be changed. They are immutable.
7.6 Length Function:
Ritu wants to find how many historical events she saved. She forgot the count. Let’s help her!!!
num_events = len(historical_events) print(num_events)
4
Ritu found her answer there are 4 historical events
7.7 Iterate through a Tuple
We can also iterate through a Tuple using loops. we will learn about loops a bit later in details for now let’s just undertsand how to use it. We can use the same method to iterate through a list.
for event in historical_events: print(event)
(“India’s Independence”, 1947) (“India’s Nuclear Test”, 1998) (‘COVID-19 Pandemic’, 2020)
(‘Chandrayaan 3’, 2023)
7.8 Delete a Tuple
Ritu wants to delete the file of historical_events
NameError
Input In [24], in <cell line: 1>()
—-> 1 print(historical_events)
Traceback (most recent call last)
NameError: name ‘historical_events’ is not defined
See historical_events has already been deleted so we get a NameError when we try to print it.
7.9 Addition of tuples:
We can add two tuples. Tuples in Python are immutable, meaning that once they are created, their content cannot be changed. However, we can “add” tuples together by concatenating them, which creates a new tuple that combines the elements of the original tuples.
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6) result = tuple1 + tuple2
print(tuple1)
print(tuple2)
print(result)
(1, 2, 3)
(4, 5, 6)
(1, 2, 3, 4, 5, 6)
See there were no changes made in tuple1 and tuple2 rather a new tuple was created “result”.
7.10 Modifying Tuple Values part 2
Now we all saw above that tuple values are not changeable but suppose we made a mistake while making the tuple. Being a human, making errors is just a part of life. So in that case if we are not able to modify the tuple value then we either need to delete the tuple or create a new one.
#This is a tuple
my_tuple = (1,2,9,4,5) #converting the tuple to a list temp_tuple = list(my_tuple) #changing value
temp_tuple[2] = 3
# again converting the list to a tuple
my_tuple = tuple(temp_tuple) #Tuple is modified print(my_tuple)
(1, 2, 3, 4, 5)
See we were able to modify tuple. Exciting isn’t it? We simply convert the tuple into a list.
make changes in the list
then we convert the list back into a tuple In the end just print the tuple
Hurray!!! all the changes are made
7.11 Tuple Characteristics
Tuples are:
- Ordered – They maintain the order of
- Immutable – They cannot be changed after
- Allow duplicates – They can contain duplicate
8 Python Dictionaries
8.1 What is a Dictionary? —————–> The Labelled Box
A dictionary is an unordered collection of key-value pairs. It’s like a real dictionary where you look up a word (key) and get its meaning (value). ## How to Create a Dictionary? We create a dictionary by placing key: value pairs inside curly brackets {}, separated by commas.
Suppose we want to store and manage inventory in a store. We can store apple and quantity of apple side by side
inventory = {
“apple”: 50,
“banana”: 100,
“orange”: 75
}
print(inventory)
{‘apple’: 50, ‘banana’: 100, ‘orange’: 75}
8.2 Accessing Inventory Items (Dictionary)
Access dictionary values using their keys.
print(inventory[“apple”])
50
8.3 Modifying an Inventory (Dictionary)
Dictionaries are mutable, so you can add or change key-value pairs.
inventory[“apple”] = 60
inventory[“orange”] = 80 print(inventory)
{‘apple’: 60, ‘banana’: 100, ‘orange’: 80}
NOTE: Keys of a dictionary are immutable
8.4 Add Items to the inventory (Dictionary)
We can add an item to a dictionary by assigning a value to a new key.
inventory[“pear”] = 40
inventory[“mango”] = 25
inventory[“pineapple”] = 20 print(inventory)
{‘apple’: 60, ‘banana’: 100, ‘orange’: 80, ‘pear’: 40, ‘mango’: 25, ‘pineapple’:
20}
8.5 Iterate Through the items in inventory (Dictionary)
# print dictionary keys one by one
for item in inventory: print(item)
apple
banana
orange
pear
mango
pineapple
# print dictionary values one by one
for item in inventory: items = inventory[item] print(items)
60
100
80
40
25
20
8.6 Dictionary Length
We can find the length of a dictionary by using the len() function.
print(len(inventory))
6
8.7 There are some more Dictionary methods such as :
- pop() – Removes the item with the specified
- update() – Adds or changes dictionary items.
- clear() – Remove all the items from the
- keys() – Returns all the dictionary’s
- values() – Returns all the dictionary’s
- get() – Returns the value of the specified
- popitem() – Returns the last inserted key and value as a
- copy() – Returns a copy of the dictionary. Let’s explore some of them :
#POP
marks = { ‘Physics’: 67, ‘Chemistry’: 72, ‘Math’: 89 } element = marks.pop(‘Chemistry’)
print(‘Popped Marks:’, element)
Popped Marks: 72
The line “element = marks.pop(‘Chemistry’)” removes the entry for ‘Chemistry’ from the dictionary and stores its value (72) in the variable element. The print() function then outputs this value, showing what was removed. The pop() method is useful when you need to remove an item from a dictionary and work with its value.
#GET
scores = {
‘Physics’: 67,
‘Maths’: 87,
‘History’: 75
}
result = scores.get(‘Physics’)
print(result)
67
The dictionary scores contains key-value pairs representing subject names and their corresponding scores.
result = scores.get(‘Physics’) retrieves the value associated with the key ‘Physics’ from the scores dictionary. The get() method returns the value 67, which is the score for Physics.
#UPDATE
marks = {‘Physics’:67, ‘Maths’:87} internal_marks = {‘Practical’:48}
marks.update(internal_marks)
print(marks)
{‘Physics’: 67, ‘Maths’: 87, ‘Practical’: 48}
The marks dictionary initially contains scores for Physics and Maths. The internal_marks dictio- nary holds the Practical score. By calling marks.update(internal_marks), the contents of inter- nal_marks are added to the marks dictionary.
#COPY
original_marks = {‘Physics’:67, ‘Maths’:87} copied_marks = original_marks.copy()
print(‘Original Marks:’, original_marks) print(‘Copied Marks:’, copied_marks)
Original Marks: {‘Physics’: 67, ‘Maths’: 87}
Copied Marks: {‘Physics’: 67, ‘Maths’: 87}
It uses the copy() method to create copied_marks from original_marks. The print() statements display both dictionaries, illustrating that copied_marks is a separate copy of original_marks.
8.8 Removing Dictionary Items
We can remove items using the del statement, the pop() method, or the popitem() method
student_info = { “name”: “Ajay”, “age”: 32,
“major”: “Computer Science”, “graduation_year”: 2012
}
# Removing an item using the del statement
del student_info[“major”]
# Removing an item using the pop() method
graduation_year = student_info.pop(“graduation_year”)
print(student_info)
{‘name’: ‘Ajay’, ‘age’: 32}
9 Python Sets
9.1 What is a Set? ——————> The Unique Box
A set is an unordered collection of unique items. It’s like a list, but it automatically removes duplicates. ## How to Create a Set? Use curly braces {} to define a set, with items separated by commas.
Imagine tracking participants who have signed up for two different workshops:
workshop_a = {“Chintu”, “Chinki”, “Coco”} workshop_b = {“Bindu”, “Darsh”, “Emli”} print(workshop_a)
print(workshop_b)
{‘Chinki’, ‘Chintu’, ‘Coco’}
{‘Bindu’, ‘Emli’, ‘Darsh’}
9.2 Accessing Set Items
We can’t access set items using an index because sets are unordered, but you can loop through the set or check if an item exists.
print(workshop_a[0])
TypeError
Input In [40], in <cell line: 1>()
—-> 1 print(workshop_a[0])
Traceback (most recent call last)
TypeError: ‘set’ object is not subscriptable
We encounter TypeError
9.3 Modifying a Set
Sets are mutable, allowing us to add or remove items.
workshop_a.add(“Saloni”)
workshop_b.remove(“Darsh”)
print(workshop_a)
print(workshop_b)
{‘Chinki’, ‘Chintu’, ‘Saloni’, ‘Coco’}
{‘Bindu’, ‘Emli’}
9.4 create an empty set
Creating an empty set is a bit tricky. Empty curly braces {} will make an empty dictionary in Python.
To make a set without any elements, we use the set() function without any argument.
empty_set = set()
9.5 Set Operations
sets support various set operations, which is used to manipulate and compare sets. These oper- ations include union, intersection, difference, symmetric difference, and subset testing. Sets are particularly useful when dealing with collections of unique elements and performing operations based on set theory.
- Union − It combine elements from both sets using the union() function or the |
- Intersection − It is used to get common elements using the intersection() function or the &
- Difference − It is used to get elements that are in one set but not the other using the difference() function or the –
- Symmetric Difference − It is used to get elements that are in either of the sets but not in both using the symmetric_difference() method or the ^
Imagine you have two different lists related to gardening:
- Gardening Tools: These are the items you use to help with For example, you might have a shovel, a rake, and some gloves.
- Plants: These are the things you’re growing in your For instance, you might have roses, tulips, and sunflowers.
Now, let’s use these two lists to see how sets in Python can help us organize and compare them using four main operations: Union, Intersection, Difference, and Symmetric Difference.
9.5.1 Creating Sets
gardening_tools = {“shovel”, “rake”, “pruning shears”, “gloves”} plants = {“rose”, “tulip”, “shovel”, “gloves”, “sunflower”}
9.5.2 Union - Combine elements from both sets
all_items = gardening_tools.union(plants)
print(“Union of gardening tools and plants:”, all_items)
Union of gardening tools and plants: {‘sunflower’, ‘gloves’, ‘shovel’, ‘pruning shears’, ‘rake’, ‘rose’, ‘tulip’}
This gives us a list of everything in either the gardening tools or the plants list. It’s like combining all our tools and plants into one big list.
9.5.3 Intersection - Get common elements
Finds items that are in both lists
common_items = gardening_tools.intersection(plants)
print(“Common items in both sets:”, common_items)
Common items in both sets: {‘gloves’, ‘shovel’}
This tells us what items appear in both lists. For instance, if “shovel” and “gloves” are in both your tools and your plants list, it shows them here.
9.5.4 Difference - Get elements in gardening tools but not in plants
Shows items that are in one list but not in the other.
unique_tools = gardening_tools.difference(plants)
print(“Gardening tools not in plants:”, unique_tools)
Gardening tools not in plants: {‘rake’, ‘pruning shears’}
This shows which tools you have that are not plants. It’s like finding out which gardening tools you have that aren’t listed as plants in your garden.
9.5.5 Symmetric Difference - Get elements in either set but not in both
Finds items that are in one list or the other, but not in both.
unique_items = gardening_tools.symmetric_difference(plants)
print(“Unique items in either set but not in both:”, unique_items)
Unique items in either set but not in both: {‘rake’, ‘sunflower’, ‘rose’, ‘tulip’, ‘pruning shears’}
This helps you find items that are unique to either the gardening tools or the plants list, but not in both. It’s like finding out which items you have that don’t overlap between the two lists.
There are some more functions on sets which you can learn more about by referring to official Python doumentation 🙂
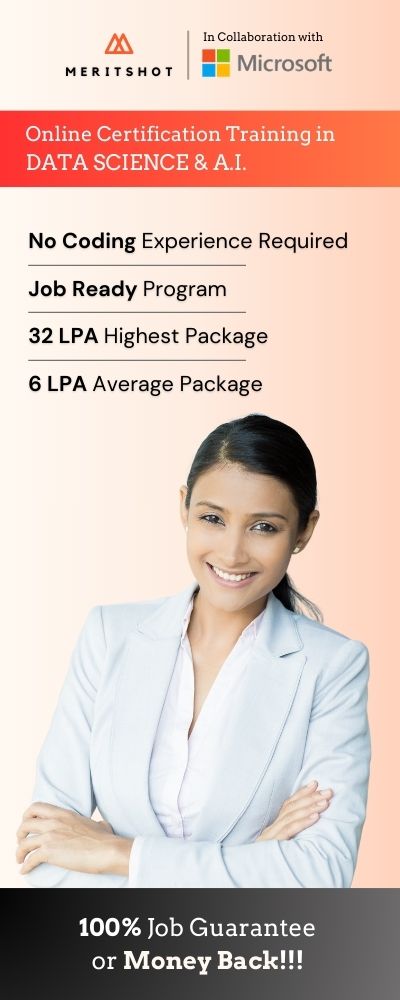