Meritshot Tutorials
- Home
- »
- What is a Lambda Function
SQL Tutorial
-
Understanding Variables in PythonUnderstanding Variables in Python
-
Types Of Operators in PythonTypes Of Operators in Python
-
How to create string ?How to create string ?
-
Data Structure in PythonData Structure in Python
-
What is a Function in PythonWhat is a Function in Python
-
Parameters and Arguments in PythonParameters and Arguments in Python
-
What is a Lambda FunctionWhat is a Lambda Function
-
What is a Regular Expression?What is a Regular Expression?
-
Introduction to Loops in PythonIntroduction to Loops in Python
-
If-else Statements in PythonIf-else Statements in Python
-
Break Statement in PythonBreak Statement in Python
-
OOPS in PythonOOPS in Python
-
Space and Time in PythonSpace and Time in Python
-
Data Type in PythonData Type in Python
Anonymous Functions (Lambda Functions)
1.1 What is a Lambda Function?
A lambda function is a small, one-line function that doesn’t need a name. It’s useful for quick,
simple operations.
* syntax lambda arguments: expression
Suppose you want to sort a list of tuples based on the second item in each tuple:
# List of tuples
data = [(1, ‘apple’), (2, ‘banana’), (3, ‘cherry’), (4, ‘date’)]
# Sort the list of tuples by the second item in each tuple using a lambda␣
↪function
sorted_data = sorted(data, key=lambda x: x[1])
print(sorted_data)
[(1, ‘apple’), (2, ‘banana’), (3, ‘cherry’), (4, ‘date’)]
• sorted() is used to sort the list.
• key=lambda x: x[1] specifies that the sorting should be based on the second item in each
tuple (the fruit name).
• lambda x: x[1] is a lambda function that takes a tuple x and returns the second element of
that tuple (x[1]).
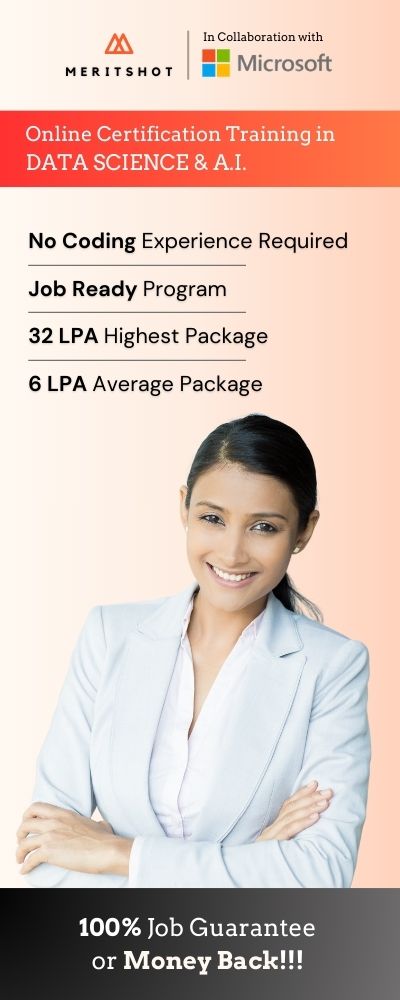