Meritshot Tutorials
- Home
- »
- What is a Function in Python
Python Tutorial
-
Understanding Variables in PythonUnderstanding Variables in Python
-
Types Of Operators in PythonTypes Of Operators in Python
-
How to create string ?How to create string ?
-
Data Structure in PythonData Structure in Python
-
What is a Function in PythonWhat is a Function in Python
-
Parameters and Arguments in PythonParameters and Arguments in Python
-
What is a Lambda FunctionWhat is a Lambda Function
-
What is a Regular Expression?What is a Regular Expression?
-
Introduction to Loops in PythonIntroduction to Loops in Python
-
If-else Statements in PythonIf-else Statements in Python
-
Break Statement in PythonBreak Statement in Python
-
OOPS in PythonOOPS in Python
-
Space and Time in PythonSpace and Time in Python
-
Data Type in PythonData Type in Python
1 Functions
1.1 What is a Function?
A function is like a machine in programming. You give it some input, it does a specific task, and then it gives you an output. Functions help you organize your code into reusable pieces.
1.2 How to Define a Function ?
You define a function using the def keyword
We write a code that automatically greets if any new user comes to our website whenever we call it.
def greet(name): print(f”Namaste, {name}!”)
If we run the above code, we won’t get an output.
It’s because creating a function doesn’t mean we are executing the code inside it. It means the code is there for us to use if we want to.
- def: This keyword starts the function
- greet: This is the name of the
- name: This is a Think of it as a placeholder for the information you will provide.
- print(f“Namaste, {name}!”): This line is the body of the function, where it does its work (printing a greeting).
1.3 How to Call a Function ?
Once you have defined a function, you can use it by calling it. You do this by writing the function’s name followed by parentheses.
greet(“Roshan”)
Namaste, Roshan!
Function Components: * Function Name: The name you give to the function, like greet. * Parameters: Inputs to the function, like name. * Return Value: What the function gives back after doing its job.
1.4 The return Statement
We return a value from the function using the return statement
def calculate_area(length, width): area = length * width
return area
area = calculate_area(5, 10) print(‘Area of the rectangle:’, area)
Area of the rectangle: 50
NOTE : Indentation is important.
1.5 The pass Statement
The pass statement serves as a placeholder for future code, preventing errors from empty code blocks.
def future_function():
pass
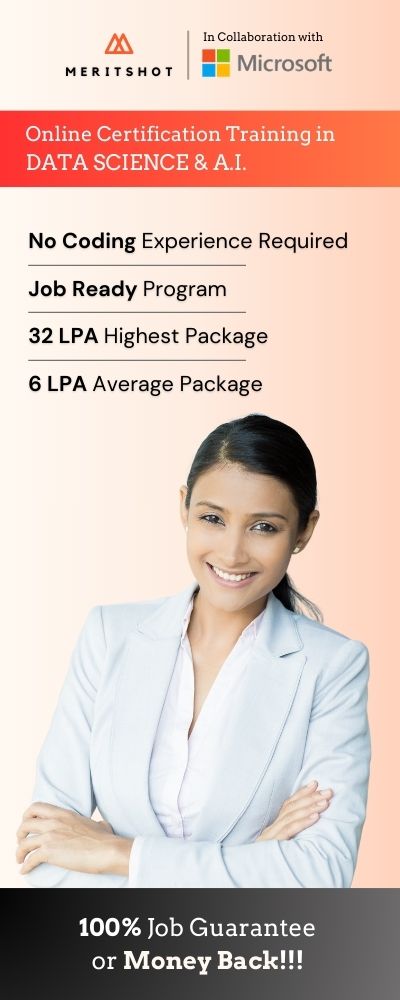