Meritshot Tutorials
- Home
- »
- How to create string
SQL Tutorial
-
Understanding Variables in PythonUnderstanding Variables in Python
-
Types Of Operators in PythonTypes Of Operators in Python
-
How to create string ?How to create string ?
-
Data Structure in PythonData Structure in Python
-
What is a Function in PythonWhat is a Function in Python
-
Parameters and Arguments in PythonParameters and Arguments in Python
-
What is a Lambda FunctionWhat is a Lambda Function
-
What is a Regular Expression?What is a Regular Expression?
-
Introduction to Loops in PythonIntroduction to Loops in Python
-
If-else Statements in PythonIf-else Statements in Python
-
Break Statement in PythonBreak Statement in Python
-
OOPS in PythonOOPS in Python
-
Space and Time in PythonSpace and Time in Python
-
Data Type in PythonData Type in Python
1 Strings
a string is a sequence of characters enclosed within quotes. Strings are one of the most commonly used data types in Python and are versatile for various operations such as manipulation, formatting, and searching. Let’s understand some functions of strings.
1.1 How to create string ?
To create strings we can either enclose the words in single or double quotes. for example: ‘Hello World’
“Hello World”
Both are correct
print(‘Hello World’)
print(“Hello World”)
Hello World
Hello World
See there is no diffrence between the two sentences the result is same
1.2 Now let’s see some string operations
1.3 1. Concatenation
You can concatenate (join) two or more strings using the + operator.
W1 = “Good”
W2 = “Byeee”
W3 = W1+W2
print(W3)
GoodByeee
1.4 2.Repetition
You can repeat a string multiple times using the * operator.
name = “Dyuti! ” * 3
print(name)
Dyuti! Dyuti! Dyuti!
1.5 3.Slicing
You can extract a portion of a string using slicing
text = “Hello, Kitty!”
slice = text[7:12] # 7 is starting index and 12 is ending index, 7th value is␣
↪not included
print(slice)
Kitty
1.6 4.Indexing
You can access individual characters in a string using indexing
text = “Hello, Kitty!”
first_char = text[0]
print(first_char)
H
1.6.1 NOTE : Indexing starts at 0
1.7 5. Length
ou can find the length of a string using the len() function.
text = “Hello, Kitty!”
length = len(text)
print(length)
13
1.8 6. lower() and upper()
Convert a string to lowercase or uppercase
text = “Hello, Kitty!”
print(text.lower())
print(text.upper())
hello, kitty!
HELLO, KITTY!
1.9 7.strip()
Remove whitespace from the beginning and end of a string.
text = ” Hello, Kitty!
” print(text.strip())
Hello, Kitty!
1.10 8. replace()
Replace occurrences of a substring with another substring. Part of a string is known as substring
text = “Hello, Kitty!”
result = text.replace(‘Hello’,’Byee’)
print(result)
Byee, Kitty!
1.11 9.split()
Split a string into a list of substrings based on a delimiter
text = “apple,banana,cherry”
fruits = text.split(“,”)
print(fruits)
[‘apple’, ‘banana’, ‘cherry’]
10. join()
Join a list of strings into a single string with a specified separator
fruits = [‘apple’, ‘banana’, ‘cherry’]
text = “, “.join(fruits)
print(text)
apple, banana, cherry
1.13 11.f-Strings
Use f-strings for more readable and concise string formatting.
This is a newly added function in the latest module of Python.(Python 3.6+).
name = “Bhuvan Bam”
age = 30
message = f”Name: {name}, Age: {age}“
print(message)
Name: Bhuvan Bam, Age: 30
1.13.1 NOTE: Strings in Python are immutable, which means that once a string is created, it cannot be changed. Any modification creates a new string.
text = “Hello, Kitty!”
result = text.replace(‘Hello’,’Byee’)
print(text)
print(result)
Hello, Kitty!
Byee, Kitty!
See the original string which is “text” even after modifying when we print “text” again, we get the same answer. This makes it clear that Python does not modify the existing string but it rather makes a new string. Hence, Strings are immutable i.e. cannot be changed
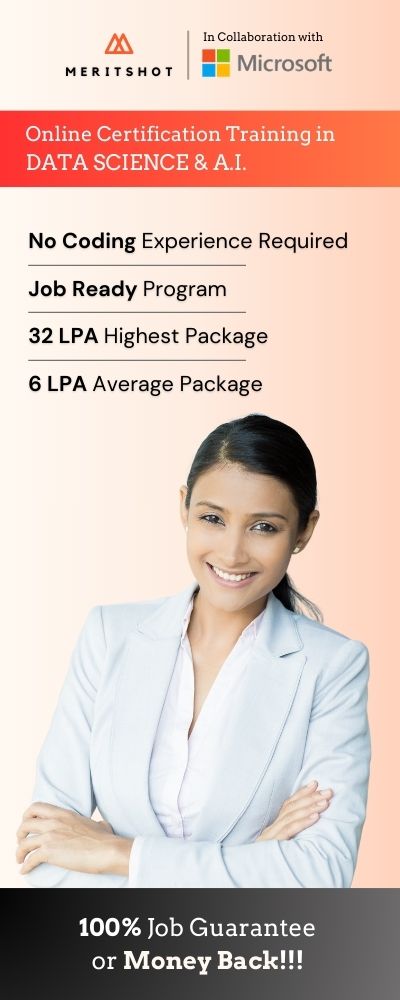