Meritshot Tutorials
- Home
- »
- Understanding Routes and Endpoints
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Serving JSON ResponsesServing JSON Responses
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Understanding Routes and Endpoints
In Flask, routes and endpoints are the key components that define how your application handles requests and sends responses. When deploying machine learning models, these routes allow you to serve predictions via HTTP requests.
What Are Routes and Endpoints?
- Route: A route is a URL pattern associated with a specific function in your Flask application. It tells Flask how to respond to a particular request type (GET, POST, etc.) for a given URL.
- Endpoint: An endpoint is a function that is called when a particular route is accessed. It handles the logic for that route, such as processing input data, making predictions, and sending responses.
How Routes Work in Flask
When a user or application makes an HTTP request (for example, a POST request with input data), Flask maps that request to a route based on the URL pattern and HTTP method. The corresponding endpoint function is then executed to handle the request and return a response.
Basic Example of a Route
Let’s take a look at a basic Flask route that receives a request and returns a response:
from flask import Flask
app = Flask(__name__)
# Define a route that listens for GET requests at the URL “/”
@app.route(“/”, methods=[“GET”])
def home():
return “Hello, World!”
if __name__ == “__main__”:
app.run(debug=True)
- Route: / (the root of the application)
- HTTP Method: GET
- Function: home() – this function simply returns a string “Hello, World!” when the route is accessed.
Routing for Machine Learning Models
When deploying machine learning models, routes are typically set up to receive data, process it, make predictions using the trained model, and return the results as a response.
Example: Creating a Route for Predictions
Let’s consider a route where a user can send data (e.g., house features) to get a house price prediction from a trained machine learning model.
from flask import Flask, request, jsonify
import pickle
import numpy as np
# Load the trained model (assuming it’s saved as ‘house_price_model.pkl’)
with open(“house_price_model.pkl”, “rb”) as file:
model = pickle.load(file)
app = Flask(__name__)
# Define a route for predictions
@app.route(“/predict”, methods=[“POST”])
def predict():
# Extract the features from the incoming JSON request
data = request.get_json()
features = np.array(data[“features”]).reshape(1, -1)
# Make a prediction using the model
prediction = model.predict(features)
# Return the prediction as a JSON response
return jsonify({“predicted_price”: prediction[0] * 1000})
if __name__ == “__main__”:
app.run(debug=True)
Explanation of the Code:
- Route Definition: The route /predict listens for POST requests. This is common for API endpoints that receive input data.
- Request Handling:
- request.get_json() extracts the JSON data sent by the client.
- The features from the JSON data are extracted and reshaped to match the model’s expected input format.
- Prediction: The model makes a prediction using the provided features.
- Response: The prediction is returned in JSON format, which makes it easy for clients (e.g., front-end applications or other APIs) to parse and use.
Common HTTP Methods Used in Flask Routing
- GET: Typically used for retrieving data (e.g., viewing the results of a query or getting a prediction form).
- POST: Used to send data to the server for processing (e.g., submitting input data for predictions).
- PUT: Used for updating existing resources (not commonly used in ML model deployment).
- DELETE: Used for removing resources (rarely used for ML model deployment).
Best Practices for Flask Routing in ML Deployments
- Use RESTful Routes: Organize your routes based on REST principles. For example:
- /predict for predictions
- /train for training a model
- Input Validation: Always validate the incoming data to ensure it’s in the correct format and type before passing it to the model for predictions.
- Error Handling: Implement proper error handling in case of incorrect requests, such as invalid data formats or missing values.
Frequently Asked Questions
- Q: How can I handle multiple routes for different models?
A: You can define separate routes for different models, such as /predict_house_price and /predict_iris. Each route would load and use a different model to make predictions. - Q: Can I pass multiple input features for a prediction?
A: Yes, you can pass multiple input features in the request data, and Flask can process them accordingly to make a prediction using the machine learning model. - Q: How do I deploy multiple versions of the same model?
A: You can define different routes or endpoints for each version of the model, such as /v1/predict and /v2/predict, or use versioning in the URL path like /predict/v1. - Q: Can I use Flask for both training and serving models?
A: Yes, Flask can be used for both tasks, but typically, model training is done offline and the trained model is loaded for serving predictions in a production environment. - Q: How do I secure my Flask routes?
A: You can secure routes by implementing authentication methods, such as token-based authentication (JWT) or OAuth, to restrict access to the endpoints.
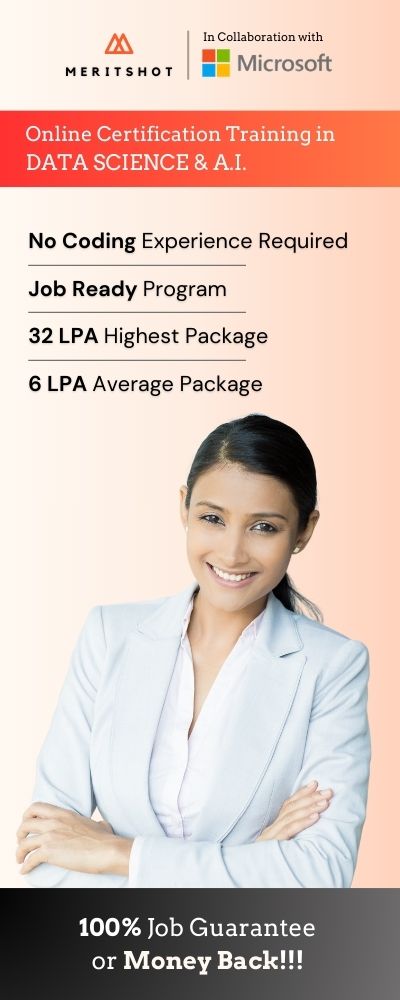