Meritshot Tutorials
- Home
- »
- Testing API Endpoints with Postman
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Serving JSON ResponsesServing JSON Responses
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Testing API Endpoints with Postman
Postman is a popular API development and testing tool that allows you to test your API endpoints without writing any code. It is an essential tool for ensuring that your Flask application is serving machine learning predictions correctly and that your API behaves as expected. You can send different types of HTTP requests (GET, POST, PUT, DELETE, etc.) to your Flask API and inspect the responses.
In this section, we will cover how to use Postman to test the Flask API endpoints we created earlier, including submitting requests and checking the JSON responses.
Steps to Test API Endpoints with Postman
Step 1: Install Postman
- Download and install Postman from the official website: https://www.postman.com/downloads/.
- Once installed, open Postman to start testing your API.
Step 2: Set Up Your Flask Application Ensure your Flask application is running locally on your machine. If you have created an API for serving machine learning predictions, make sure the Flask server is running, and your application is accessible at http://127.0.0.1:5000/ or the address you specified.
Step 3: Create a New Request in Postman
- Open Postman and click on the “New” button in the top left corner.
- Choose “Request” to create a new API request.
- Name your request (e.g., “Predict Image”) and save it to a collection.
- Select the HTTP method, such as POST, which is typically used for sending data to the server (like submitting an image file).
Step 4: Sending a Request to Your Flask API
In this example, we are going to test the /predict endpoint of the Flask application. The /predict endpoint accepts a POST request and returns a JSON response with the model’s prediction.
- Set Request Type to POST
- In Postman, change the request type from the default GET to POST by selecting POST from the dropdown next to the URL field.
- Enter the URL for Your Flask Endpoint
- In the URL field, enter the URL of the endpoint you want to test. If your Flask app is running locally, use:
http://127.0.0.1:5000/predict
- Add Image to the Request
- Since our Flask API expects an image file as part of the request, go to the Body tab in Postman.
- Select form-data as the type of request body.
- Add a key named image (or the key used in your Flask code) and select the File option.
- Choose an image file from your system to upload.
- Send the Request
- Once the image is added, click Send to submit the request to the Flask API.
Step 5: Inspecting the Response
After sending the request, you will see the response in the lower section of the Postman interface.
- If the request is successful, the Flask API will return a JSON response with the predicted class and the probability (if implemented):
{
“prediction”: 5,
“probability”: 0.8765,
“message”: “Prediction successful”
}
- If there’s an error, you might receive a different JSON response with error details:
{
“error”: “Invalid image format”,
“message”: “The provided image is not in a valid format”
}
Step 6: Testing with Different Data
You can repeat the process by sending different types of input data to the /predict endpoint:
- Try different image files to see how the model performs with various inputs.
- Test with missing or invalid files to see how your Flask API handles errors and whether appropriate error messages are returned.
Step 7: Using Postman for More Complex API Tests
Postman is not just limited to simple requests. It also supports:
- Adding Headers: For example, you can add an Authorization header if your API requires token-based authentication.
- Pre-request Scripts: You can write scripts that run before sending the request, such as adding dynamic authentication tokens.
- Tests: Postman allows you to write tests to automate checks on the response. For instance, you can check if the response contains the right JSON format:
pm.test(“Response should be JSON”, function () { pm.response.to.have.jsonBody();
});
Testing Different HTTP Methods
While the /predict endpoint uses the POST method, Postman can also test other HTTP methods:
- GET: Fetch data from the API.
- PUT/PATCH: Update existing data.
- DELETE: Remove data from the server.
Make sure to test all the necessary API methods for your use case.
Frequently Asked Questions
- What should I do if Postman is not able to connect to my Flask app?
- Answer: Ensure your Flask application is running, and check if it is accessible at http://127.0.0.1:5000/. You can also try using http://localhost:5000/. Make sure there are no firewall or network issues.
- How do I send a JSON payload with a POST request in Postman?
- Answer: To send a JSON payload, select the Body tab in Postman and choose the raw option. Then, set the content type to JSON and enter your JSON data, for example:
{
“data”: [1.0, 2.5, 3.0, 4.5]
}
- How can I add authentication tokens when testing an API in Postman?
- Answer: In the Authorization tab, select the authentication type (e.g., Bearer Token). Enter the token you want to use for the request.
- Can I test the Flask API without Postman?
- Answer: Yes, you can use tools like cURL or build a frontend that makes requests to the Flask API. These methods are particularly useful for automated testing or for integrating with other systems.
- How do I view the request and response details in Postman?
- Answer: After sending a request, Postman will show the response in the lower section. You can view the status code, headers, body, and even the raw request and response logs.
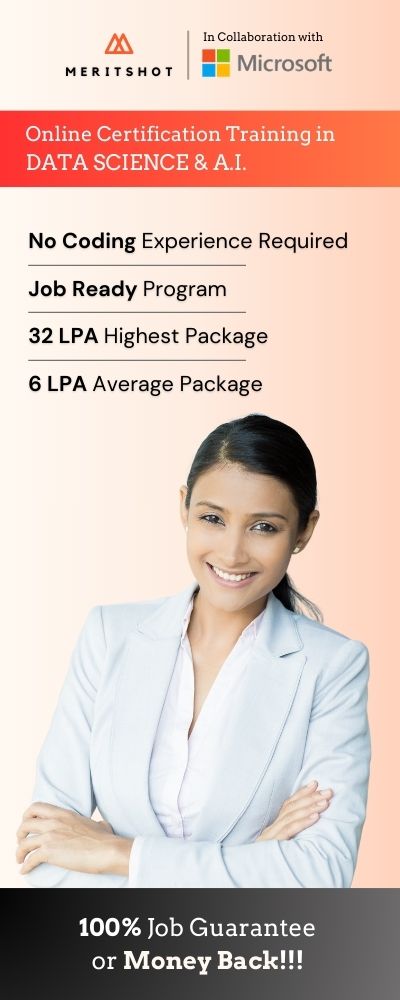