Meritshot Tutorials
- Home
- »
- SQL Views
SQL Tutorial
-
SQL SyntaxSQL Syntax
-
SQL ORDER BY ClauseSQL ORDER BY Clause
-
Introduction to SQLIntroduction to SQL
-
SQL SELECT DISTINCTSQL SELECT DISTINCT
-
SQL Logical OperatorsSQL Logical Operators
-
SQL WHERE ClauseSQL WHERE Clause
-
SQL SELECT StatementSQL SELECT Statement
-
SQL DELETE StatementSQL DELETE Statement
-
SQL INSERT INTOSQL INSERT INTO
-
SQL Null ValuesSQL Null Values
-
SQL Update StatementSQL Update Statement
-
SQL Select TopSQL Select Top
-
SQL Aggregate FunctionsSQL Aggregate Functions
-
SQL LIKE and WildcardsSQL LIKE and Wildcards
-
SQL IN and SQL BETWEENSQL IN and SQL BETWEEN
-
SQL JOINSSQL JOINS
-
SQL Group BySQL Group By
-
SQL HavingSQL Having
-
SQL EXISTSSQL EXISTS
-
SQL SELECT INTOSQL SELECT INTO
-
SQL INSERT INTO SELECTSQL INSERT INTO SELECT
-
SQL CASE STATEMENTSQL CASE STATEMENT
-
SQL NULL FunctionsSQL NULL Functions
-
SQL Stored ProceduresSQL Stored Procedures
-
SQL User-Defined FunctionsSQL User-Defined Functions
-
SQL CommentsSQL Comments
-
SQL OperatorsSQL Operators
-
SQL Database Creation and ManagementSQL Database Creation and Management
-
SQL CREATE DATABASE StatementSQL CREATE DATABASE Statement
-
SQL CREATE TABLE StatementSQL CREATE TABLE Statement
-
SQL DROP DATABASE StatementSQL DROP DATABASE Statement
-
SQL DROP TABLE StatementSQL DROP TABLE Statement
-
SQL ALTER TABLE StatementSQL ALTER TABLE Statement
-
SQL NOT NULL ConstraintSQL NOT NULL Constraint
-
SQL UNIQUE ConstraintSQL UNIQUE Constraint
-
SQL PRIMARY KEY ConstraintSQL PRIMARY KEY Constraint
-
SQL FOREIGN KEY ConstraintSQL FOREIGN KEY Constraint
-
SQL CHECK ConstraintSQL CHECK Constraint
-
SQL DEFAULT ConstraintSQL DEFAULT Constraint
-
SQL IndexesSQL Indexes
-
SQL Date FunctionsSQL Date Functions
-
SQL ViewsSQL Views
-
SQL InjectionSQL Injection
-
SQL Data Types OverviewSQL Data Types Overview
-
SQL AUTO_INCREMENTSQL AUTO_INCREMENT
-
SQL Keywords ReferenceSQL Keywords Reference
SQL Views
A VIEW in SQL is a virtual table that provides a way to present data from one or more tables in a specific format or structure. Views do not store data themselves but are defined by a query that retrieves data from underlying tables. They can be used to simplify complex queries, encapsulate data access, and provide a consistent interface to data.
Syntax
— Creating a view
CREATE VIEW view_name AS
SELECT column1, column2, …
FROM table_name
WHERE condition;
— Updating a view
CREATE OR REPLACE VIEW view_name AS
SELECT column1, column2, …
FROM table_name
WHERE condition;
— Dropping a view
DROP VIEW view_name;
- view_name: The name of the view to be created, updated, or dropped.
- column1, column2, …: The columns to be included in the view.
- table_name: The underlying table(s) from which the view retrieves data.
- condition: The criteria for filtering data in the view.
Example:
1 Creating a Basic View
Suppose you have a table Employees and you want to create a view that shows only employees from the Sales department:
CREATE VIEW SalesEmployees AS
SELECT EmployeeID, FullName, Position
FROM Employees
WHERE Department = ‘Sales’;
This view, SalesEmployees, will display only employees who work in the Sales department.
2 Updating an Existing View
If you want to update the SalesEmployees view to include the HireDate column:
CREATE OR REPLACE VIEW SalesEmployees AS
SELECT EmployeeID, FullName, Position, HireDate
FROM Employees
WHERE Department = ‘Sales’;
3 Updating an Existing View
If you want to update the SalesEmployees view to include the HireDate column:
CREATE OR REPLACE VIEW SalesEmployees AS
SELECT EmployeeID, FullName, Position, HireDate
FROM Employees
WHERE Department = ‘Sales’;
4 Creating a View with Joins
Suppose you have two tables, Orders and Customers, and you want to create a view that combines order details with customer information:
CREATE VIEW CustomerOrders AS
SELECT o.OrderID, o.OrderDate, c.CustomerName, c.ContactNumber
FROM Orders o
JOIN Customers c ON o.CustomerID = c.CustomerID;
This view, CustomerOrders, will show order details along with the customer names and contact numbers.
Tips to Remember
- Views Are Read-Only: In many database systems, views are read-only. To update data, you may need to perform updates on the underlying tables directly.
- Use for Security: Views can help in restricting access to sensitive data by exposing only specific columns or rows.
- Performance Considerations: While views simplify query writing, be aware that complex views or views based on other views can affect query performance.
Frequently Asked Questions
Q1: Can a view be based on another view?
A1: Yes, views can be nested. A view can be created based on another view, but be cautious of performance impacts and complexity.
Q2: Can I use views to enforce data integrity?
A2: Views themselves do not enforce data integrity, but they can provide a simplified and controlled way to access data, reducing the risk of erroneous data manipulation.
Q3: How do I refresh a view if the underlying data changes?
A3: Views are updated automatically with the latest data from the underlying tables. No action is needed to refresh the view.
Q4: Can I include aggregated data in a view?
A4: Yes, you can include aggregated data using functions like SUM(), COUNT(), AVG(), etc., in a view.
Q5: Are there any limitations to using views?
A5: Limitations may vary by database system. Common limitations include restrictions on updating views directly and potential performance impacts for complex views.
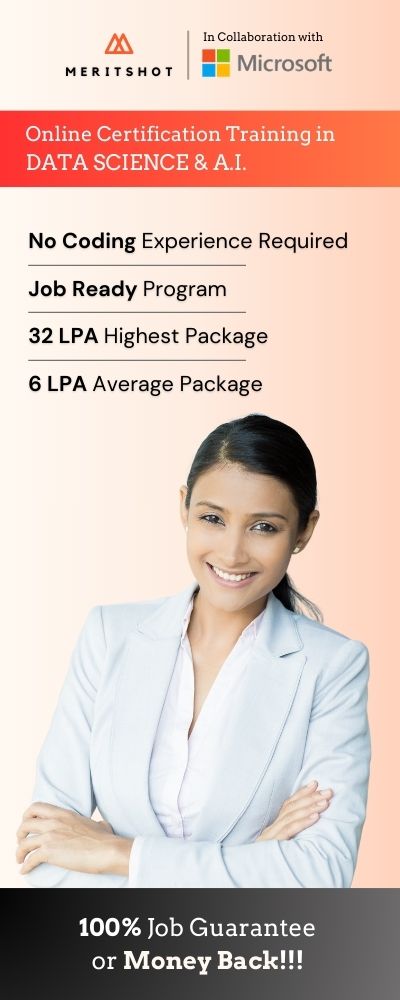