Meritshot Tutorials
- Home
- »
- SQL Syntax
SQL Tutorial
-
SQL SyntaxSQL Syntax
-
SQL ORDER BY ClauseSQL ORDER BY Clause
-
Introduction to SQLIntroduction to SQL
-
SQL SELECT DISTINCTSQL SELECT DISTINCT
-
SQL Logical OperatorsSQL Logical Operators
-
SQL WHERE ClauseSQL WHERE Clause
-
SQL SELECT StatementSQL SELECT Statement
-
SQL DELETE StatementSQL DELETE Statement
-
SQL INSERT INTOSQL INSERT INTO
-
SQL Null ValuesSQL Null Values
-
SQL Update StatementSQL Update Statement
-
SQL Select TopSQL Select Top
-
SQL Aggregate FunctionsSQL Aggregate Functions
-
SQL LIKE and WildcardsSQL LIKE and Wildcards
-
SQL IN and SQL BETWEENSQL IN and SQL BETWEEN
-
SQL JOINSSQL JOINS
-
SQL Group BySQL Group By
-
SQL HavingSQL Having
-
SQL EXISTSSQL EXISTS
-
SQL SELECT INTOSQL SELECT INTO
-
SQL INSERT INTO SELECTSQL INSERT INTO SELECT
-
SQL CASE STATEMENTSQL CASE STATEMENT
-
SQL NULL FunctionsSQL NULL Functions
-
SQL Stored ProceduresSQL Stored Procedures
-
SQL User-Defined FunctionsSQL User-Defined Functions
-
SQL CommentsSQL Comments
-
SQL OperatorsSQL Operators
-
SQL Database Creation and ManagementSQL Database Creation and Management
-
SQL CREATE DATABASE StatementSQL CREATE DATABASE Statement
-
SQL CREATE TABLE StatementSQL CREATE TABLE Statement
-
SQL DROP DATABASE StatementSQL DROP DATABASE Statement
-
SQL DROP TABLE StatementSQL DROP TABLE Statement
-
SQL ALTER TABLE StatementSQL ALTER TABLE Statement
-
SQL NOT NULL ConstraintSQL NOT NULL Constraint
-
SQL UNIQUE ConstraintSQL UNIQUE Constraint
-
SQL PRIMARY KEY ConstraintSQL PRIMARY KEY Constraint
-
SQL FOREIGN KEY ConstraintSQL FOREIGN KEY Constraint
-
SQL CHECK ConstraintSQL CHECK Constraint
-
SQL DEFAULT ConstraintSQL DEFAULT Constraint
-
SQL IndexesSQL Indexes
-
SQL Date FunctionsSQL Date Functions
-
SQL ViewsSQL Views
-
SQL InjectionSQL Injection
-
SQL Data Types OverviewSQL Data Types Overview
-
SQL AUTO_INCREMENTSQL AUTO_INCREMENT
-
SQL Keywords ReferenceSQL Keywords Reference
Understanding SQL Syntax
SQL syntax refers to the set of rules that define how SQL statements are structured and written. Just like any other programming language, SQL has its own syntax that dictates how you should write commands to interact with the database.
Basic Structure of an SQL Query
An SQL query typically follows a simple structure that includes the following components:
- Keywords: These are predefined words in SQL that perform specific actions. Examples include SELECT, FROM, WHERE, INSERT, UPDATE, and DELETE.
- Clauses: Clauses are components of SQL statements that perform specific operations. Common clauses include SELECT, FROM, WHERE, ORDER BY, and GROUP BY.
- Expressions: These include combinations of columns, constants, and operators that evaluate to a single value. For example, price * quantity is an expression.
- Predicates: These are used to specify conditions in SQL statements. For example, WHERE age > 30 is a predicate that filters data based on the condition age > 30.
- Statements: A complete SQL instruction that the database can execute, such as SELECT * FROM customers.
- Semicolon (;): In many SQL implementations, a semicolon is used to terminate a statement, especially when executing multiple statements in a script.
Example:
Of a Basic SQL Query
Here’s an example of a simple SQL query:
SELECT first_name, last_name
FROM employees
WHERE department = ‘Sales’
ORDER BY last_name;
- SELECT: This keyword specifies the columns to retrieve.
- FROM: This clause specifies the table from which to retrieve the data.
- WHERE: This clause filters the results to include only those rows where the department is ‘Sales’.
- ORDER BY: This clause sorts the result set by the last_name column.
Common SQL Commands and Their Syntax
- SELECT
- Purpose: Retrieve data from a database.
- Syntax:
SELECT column1, column2, …
FROM table_name
WHERE condition;
- INSERT
- Purpose: Add new data to a table.
- Syntax:
INSERT INTO table_name (column1, column2, …)
VALUES (value1, value2, …);
- UPDATE
- Purpose: Modify existing data in a table.
- Syntax:
UPDATE table_name
SET column1 = value1, column2 = value2, …
WHERE condition;
- DELETE
- Purpose: Remove data from a table.
- Syntax:
DELETE FROM table_name
WHERE condition;
- CREATE TABLE
- Purpose: Create a new table in the database.
- Syntax:
CREATE TABLE table_name (
column1 datatype,
column2 datatype,
);
- ALTER TABLE
- Purpose: Modify an existing table (e.g., add, delete, or modify columns).
- Syntax:
ALTER TABLE table_name
ADD column_name datatype;
- DROP TABLE
- Purpose: Delete an existing table from the database.
- Syntax:
DROP TABLE table_name;
SQL Syntax Best Practices
- Use Uppercase for SQL Keywords: Although SQL is not case-sensitive, it’s a common practice to write SQL keywords in uppercase for readability.
- Indentation and Line Breaks: Use indentation and line breaks to organize your code and make it more readable, especially for complex queries.
- Consistent Naming Conventions: Use consistent naming conventions for tables, columns, and other database objects. For example, snake_case or camelCase.
- Comment Your Code: Use comments to explain complex SQL queries. This is especially useful for queries that will be revisited or maintained by others.
— This query selects all employees in the Sales department
SELECT first_name, last_name
FROM employees
WHERE department = ‘Sales’;
What Can SQL Do?
SQL’s syntax allows you to perform a wide range of database operations, such as:
- Selecting Data: Retrieve specific data based on various conditions.
- Inserting Data: Add new records to a database.
- Updating Data: Modify existing records.
- Deleting Data: Remove records based on specific criteria.
- Creating Structures: Define new tables, views, and other database objects.
- Altering Structures: Modify the existing structure of database objects.
- Dropping Structures: Remove database objects like tables or views permanently.
Frequently Asked Questions
Q1: Do all SQL databases use the same syntax?
A1: Most SQL commands are standardized, but there can be slight variations and additional proprietary extensions depending on the database system (e.g., MySQL, SQL Server, Oracle).
Q2: Is it necessary to use a semicolon at the end of an SQL statement?
A2: While many SQL environments require a semicolon to end a statement, it depends on the system you’re using. It’s good practice to include it, especially when writing multiple statements in a script.
Q3: Are SQL keywords case-sensitive?
A3: No, SQL keywords are not case-sensitive. SELECT and select are treated the same by the database. However, it’s standard practice to write keywords in uppercase for better readability.
Q4: Can SQL queries be written on a single line?
A4: Yes, SQL queries can be written on a single line. However, breaking them into multiple lines with proper indentation is recommended for readability, especially for complex queries.
Q5: What happens if there’s a syntax error in my SQL query?
A5: If there’s a syntax error, the database will typically return an error message indicating the issue. The query will not execute until the syntax is corrected.
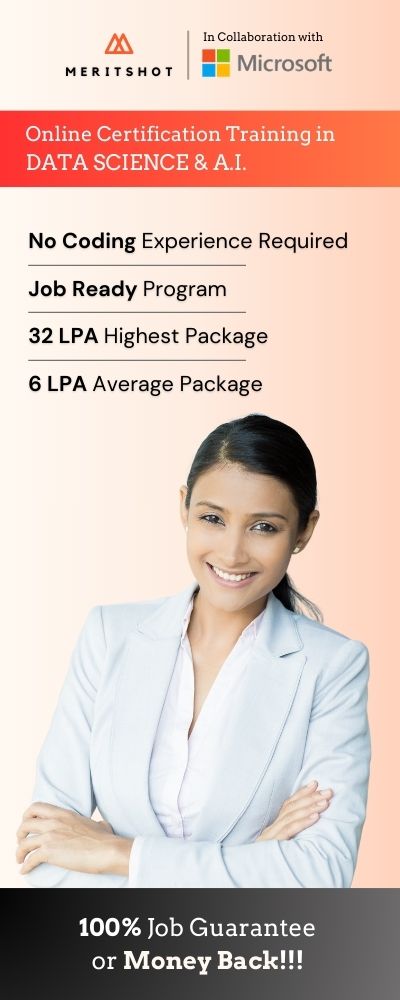