Meritshot Tutorials
- Home
- »
- SQL Keywords Reference
SQL Tutorial
-
SQL SyntaxSQL Syntax
-
SQL ORDER BY ClauseSQL ORDER BY Clause
-
Introduction to SQLIntroduction to SQL
-
SQL SELECT DISTINCTSQL SELECT DISTINCT
-
SQL Logical OperatorsSQL Logical Operators
-
SQL WHERE ClauseSQL WHERE Clause
-
SQL SELECT StatementSQL SELECT Statement
-
SQL DELETE StatementSQL DELETE Statement
-
SQL INSERT INTOSQL INSERT INTO
-
SQL Null ValuesSQL Null Values
-
SQL Update StatementSQL Update Statement
-
SQL Select TopSQL Select Top
-
SQL Aggregate FunctionsSQL Aggregate Functions
-
SQL LIKE and WildcardsSQL LIKE and Wildcards
-
SQL IN and SQL BETWEENSQL IN and SQL BETWEEN
-
SQL JOINSSQL JOINS
-
SQL Group BySQL Group By
-
SQL HavingSQL Having
-
SQL EXISTSSQL EXISTS
-
SQL SELECT INTOSQL SELECT INTO
-
SQL INSERT INTO SELECTSQL INSERT INTO SELECT
-
SQL CASE STATEMENTSQL CASE STATEMENT
-
SQL NULL FunctionsSQL NULL Functions
-
SQL Stored ProceduresSQL Stored Procedures
-
SQL User-Defined FunctionsSQL User-Defined Functions
-
SQL CommentsSQL Comments
-
SQL OperatorsSQL Operators
-
SQL Database Creation and ManagementSQL Database Creation and Management
-
SQL CREATE DATABASE StatementSQL CREATE DATABASE Statement
-
SQL CREATE TABLE StatementSQL CREATE TABLE Statement
-
SQL DROP DATABASE StatementSQL DROP DATABASE Statement
-
SQL DROP TABLE StatementSQL DROP TABLE Statement
-
SQL ALTER TABLE StatementSQL ALTER TABLE Statement
-
SQL NOT NULL ConstraintSQL NOT NULL Constraint
-
SQL UNIQUE ConstraintSQL UNIQUE Constraint
-
SQL PRIMARY KEY ConstraintSQL PRIMARY KEY Constraint
-
SQL FOREIGN KEY ConstraintSQL FOREIGN KEY Constraint
-
SQL CHECK ConstraintSQL CHECK Constraint
-
SQL DEFAULT ConstraintSQL DEFAULT Constraint
-
SQL IndexesSQL Indexes
-
SQL Date FunctionsSQL Date Functions
-
SQL ViewsSQL Views
-
SQL InjectionSQL Injection
-
SQL Data Types OverviewSQL Data Types Overview
-
SQL AUTO_INCREMENTSQL AUTO_INCREMENT
-
SQL Keywords ReferenceSQL Keywords Reference
SQL Keywords Reference
SQL keywords are reserved words in SQL that have special meanings and functions. These keywords are used to perform various operations and queries on the database. Understanding and using SQL keywords correctly is essential for writing effective SQL queries and managing databases.
Common SQL Keywords
1 SELECT: Retrieves data from one or more tables.
Example:
SELECT column1, column2 FROM table_name;
2 FROM: Specifies the table from which to retrieve data.
Example:
FROM: Specifies the table from which to retrieve data.
3 WHERE: Filters records based on specified conditions.
Example:
SELECT column1 FROM table_name WHERE condition;
4 INSERT INTO: Adds new rows to a table.
Example:
INSERT INTO table_name (column1, column2) VALUES (value1, value2);
5 UPDATE: Modifies existing rows in a table.
Example:
UPDATE table_name SET column1 = value1 WHERE condition;
6 DELETE: Removes rows from a table.
Example:
DELETE FROM table_name WHERE condition;
7 JOIN: Combines rows from two or more tables based on a related column.
Example:
SELECT columns FROM table1 JOIN table2 ON table1.column = table2.column;
8 GROUP BY: Groups rows that have the same values in specified columns into summary rows.
Example:
SELECT column1, COUNT(*) FROM table_name GROUP BY column1;
9 ORDER BY: Sorts the result set by one or more columns.
Example:
SELECT column1 FROM table_name ORDER BY column1 ASC;
10 HAVING: Filters groups based on a specified condition, used with GROUP BY.
Example:
SELECT column1, COUNT(*) FROM table_name GROUP BY column1 HAVING COUNT(*) > 1;
11 DISTINCT: Removes duplicate rows from the result set.
Example:
SELECT DISTINCT column1 FROM table_name;
12 LIMIT: Specifies the number of rows to return.
Example:
SELECT column1 FROM table_name LIMIT 10;
13 OFFSET: Skips a specified number of rows before starting to return rows.
Example:
SELECT column1 FROM table_name LIMIT 10 OFFSET 20;
14 BETWEEN: Filters the result set within a range.
Example:
SELECT column1 FROM table_name WHERE column1 BETWEEN value1 AND value2;
15 IN: Filters the result set to include only rows with values in a specified list.
Example:
SELECT column1 FROM table_name WHERE column1 IN (value1, value2);
16 LIKE: Searches for a specified pattern in a column.
Example:
SELECT column1 FROM table_name WHERE column1 LIKE ‘pattern%’;
17 NULL: Represents a missing or undefined value.
Example:
SELECT column1 FROM table_name WHERE column1 IS NULL;
18 CREATE: Defines a new table, view, index, or other database object.
Example:
CREATE TABLE table_name (column1 datatype, column2 datatype);
19 ALTER: Modifies an existing database object, such as a table.
Example:
ALTER TABLE table_name ADD column_name datatype;
20 DROP: Deletes an existing database object.
Example:
DROP TABLE table_name;
21 TRUNCATE: Removes all rows from a table, but retains the structure.
Example:
TRUNCATE TABLE table_name;
22 EXECUTE: Executes a stored procedure.
Example:
EXECUTE procedure_name;
23 CALL: Calls a stored procedure or function.
Example:
CALL procedure_name(parameters);
24 BEGIN / END: Defines the start and end of a block of statements, often used in stored procedures and functions.
Example:
BEGIN
— SQL statements
END;
25 COMMIT: Saves all changes made during the current transaction.
Example:
COMMIT;
26 ROLLBACK: Reverts all changes made during the current transaction.
Example:
ROLLBACK;
Tips to Remember
- Familiarize Yourself with Keywords: Understanding the purpose and usage of SQL keywords will help you write accurate and efficient queries.
- Avoid Reserved Keywords: Be cautious when using keywords as identifiers (e.g., column or table names) to avoid conflicts and errors.
- Use Keywords Consistently: Follow consistent formatting and capitalization practices for readability and maintainability of SQL code.
Frequently Asked Questions
Q1: Are SQL keywords case-sensitive?
A1: SQL keywords are generally case-insensitive, meaning SELECT, select, and SeLeCt are treated the same. However, it’s a good practice to use consistent capitalization for readability.
Q2: Can SQL keywords be used as column or table names?
A2: While it is possible to use SQL keywords as column or table names by enclosing them in quotes, it is not recommended as it can lead to confusion and errors.
Q3: How do I find out which keywords are supported by my SQL database?
A3: Consult the documentation for your specific SQL database system to find a list of supported keywords and reserved words.
Q4: What is the purpose of the TRUNCATE keyword?
A4: The TRUNCATE keyword removes all rows from a table but retains the table structure, unlike DELETE, which also logs individual row deletions.
Q5: Can I use COMMIT and ROLLBACK outside of transactions?
A5: COMMIT and ROLLBACK are used within transactions to manage changes. They are not applicable if no transaction has been started.
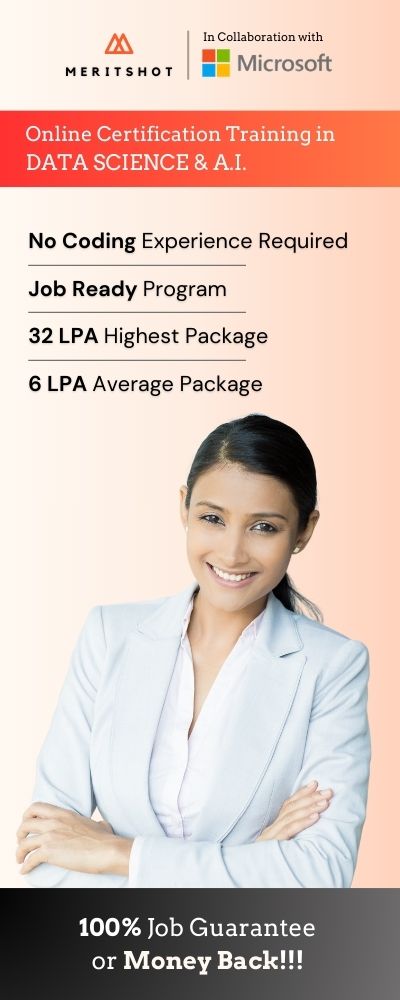