Meritshot Tutorials
- Home
- »
- SQL Injection
SQL Tutorial
-
SQL SyntaxSQL Syntax
-
SQL ORDER BY ClauseSQL ORDER BY Clause
-
Introduction to SQLIntroduction to SQL
-
SQL SELECT DISTINCTSQL SELECT DISTINCT
-
SQL Logical OperatorsSQL Logical Operators
-
SQL WHERE ClauseSQL WHERE Clause
-
SQL SELECT StatementSQL SELECT Statement
-
SQL DELETE StatementSQL DELETE Statement
-
SQL INSERT INTOSQL INSERT INTO
-
SQL Null ValuesSQL Null Values
-
SQL Update StatementSQL Update Statement
-
SQL Select TopSQL Select Top
-
SQL Aggregate FunctionsSQL Aggregate Functions
-
SQL LIKE and WildcardsSQL LIKE and Wildcards
-
SQL IN and SQL BETWEENSQL IN and SQL BETWEEN
-
SQL JOINSSQL JOINS
-
SQL Group BySQL Group By
-
SQL HavingSQL Having
-
SQL EXISTSSQL EXISTS
-
SQL SELECT INTOSQL SELECT INTO
-
SQL INSERT INTO SELECTSQL INSERT INTO SELECT
-
SQL CASE STATEMENTSQL CASE STATEMENT
-
SQL NULL FunctionsSQL NULL Functions
-
SQL Stored ProceduresSQL Stored Procedures
-
SQL User-Defined FunctionsSQL User-Defined Functions
-
SQL CommentsSQL Comments
-
SQL OperatorsSQL Operators
-
SQL Database Creation and ManagementSQL Database Creation and Management
-
SQL CREATE DATABASE StatementSQL CREATE DATABASE Statement
-
SQL CREATE TABLE StatementSQL CREATE TABLE Statement
-
SQL DROP DATABASE StatementSQL DROP DATABASE Statement
-
SQL DROP TABLE StatementSQL DROP TABLE Statement
-
SQL ALTER TABLE StatementSQL ALTER TABLE Statement
-
SQL NOT NULL ConstraintSQL NOT NULL Constraint
-
SQL UNIQUE ConstraintSQL UNIQUE Constraint
-
SQL PRIMARY KEY ConstraintSQL PRIMARY KEY Constraint
-
SQL FOREIGN KEY ConstraintSQL FOREIGN KEY Constraint
-
SQL CHECK ConstraintSQL CHECK Constraint
-
SQL DEFAULT ConstraintSQL DEFAULT Constraint
-
SQL IndexesSQL Indexes
-
SQL Date FunctionsSQL Date Functions
-
SQL ViewsSQL Views
-
SQL InjectionSQL Injection
-
SQL Data Types OverviewSQL Data Types Overview
-
SQL AUTO_INCREMENTSQL AUTO_INCREMENT
-
SQL Keywords ReferenceSQL Keywords Reference
SQL Injection
SQL Injection is a security vulnerability that occurs when an attacker is able to execute arbitrary SQL commands within a database through unsanitized input. This can lead to unauthorized access, data manipulation, and even database destruction. Understanding and mitigating SQL Injection is crucial for protecting the integrity and security of your database applications.
What is SQL Injection?
SQL Injection happens when user inputs are directly included in SQL queries without proper validation or escaping. Attackers can exploit this by injecting malicious SQL code into the query, which can be executed by the database.
Common SQL Injection Techniques
- Error-Based Injection: Exploits database error messages to gain information about the database structure.
- Union-Based Injection: Uses the UNION SQL operator to combine the results of the original query with the results of an injected query.
- Blind Injection: Extracts data from the database based on responses to true/false conditions, often when error messages are not provided.
- Boolean-Based Injection: Alters the SQL query to return different results based on true/false conditions.
Example:
1 Basic SQL Injection Example
Consider a vulnerable login query:
SELECT * FROM Users WHERE username = ‘user_input’ AND password = ‘pass_input’;
If an attacker inputs ‘ OR ‘1’=’1 as both the username and password, the query becomes:
SELECT * FROM Users WHERE username = ” OR ‘1’=’1′ AND password = ” OR ‘1’=’1′;
This query always returns true, potentially granting unauthorized access.
2 Union-Based Injection Example
Given a vulnerable query that retrieves user details:
SELECT name, email FROM Users WHERE user_id = ‘user_input’;
An attacker might inject:
‘ UNION SELECT username, password FROM Admins; —
The query becomes:
SELECT name, email FROM Users WHERE user_id = ” UNION SELECT username, password FROM Admins; –‘;
This injection can expose sensitive data from the Admins table.
3 Error-Based Injection Example
An attacker might input:
‘ AND 1=CONVERT(int, (SELECT @@version))–‘
This injection forces the database to return its version information through an error message.
Preventing SQL Injection
- Use Prepared Statements: Prepared statements with parameterized queries ensure that user inputs are treated as data, not executable code.
- MySQL:
PREPARE stmt FROM ‘SELECT * FROM Users WHERE username = ? AND password = ?’;
EXECUTE stmt USING @username, @password;
- SQL Server:
DECLARE @username NVARCHAR(50) = ‘user_input’;
DECLARE @password NVARCHAR(50) = ‘pass_input’;
EXEC sp_executesql N’SELECT * FROM Users WHERE username = @username AND password = @password’, N’@username NVARCHAR(50), @password NVARCHAR(50)’, @username, @password;
- Python (with sqlite3):
cursor.execute(‘SELECT * FROM Users WHERE username = ? AND password = ?’, (username, password))
- Use ORM Frameworks: Object-Relational Mapping (ORM) frameworks abstract database interactions and help prevent SQL injection by using safe query construction methods.
- Validate and Sanitize Input: Ensure all user inputs are validated and sanitized to prevent malicious data from entering your system. Use whitelists and proper data validation techniques.
- Escape Special Characters: If you must include user input directly in SQL queries, escape special characters to prevent them from being interpreted as SQL commands.
- Limit Database Permissions: Follow the principle of least privilege by granting only the necessary permissions to the database user accounts. This minimizes the impact of any potential SQL injection attacks.
- Regular Security Audits: Conduct regular security audits and code reviews to identify and address potential vulnerabilities.
Tips to Remember
- Always Use Parameterized Queries: This is the most effective way to prevent SQL injection.
- Educate Developers: Ensure that developers are aware of SQL injection risks and best practices for secure coding.
- Test for Vulnerabilities: Regularly test your applications for SQL injection vulnerabilities using automated tools and manual testing.
Frequently Asked Questions
Q1: Can SQL Injection be used to delete data from a database?
A1: Yes, SQL injection can be used to execute malicious SQL commands, including DELETE statements, potentially leading to data loss.
Q2: How can I identify SQL Injection vulnerabilities in my application?
A2: Use security scanning tools to test for SQL injection vulnerabilities, and conduct code reviews focusing on database query construction.
Q3: Are there any database systems less vulnerable to SQL Injection?
A3: All major database systems are vulnerable to SQL injection if proper security practices are not followed. The key is in securing the application and using best practices.
Q4: Can SQL Injection be mitigated with firewall rules?
A4: While web application firewalls (WAFs) can help detect and block SQL injection attacks, they should not be relied upon solely. Proper coding practices and parameterized queries are essential.
Q5: How often should I review my code for SQL Injection vulnerabilities?
A5: Regularly review your code, especially when adding new features or making changes that involve database queries. Continuous security practices are important for maintaining application security.
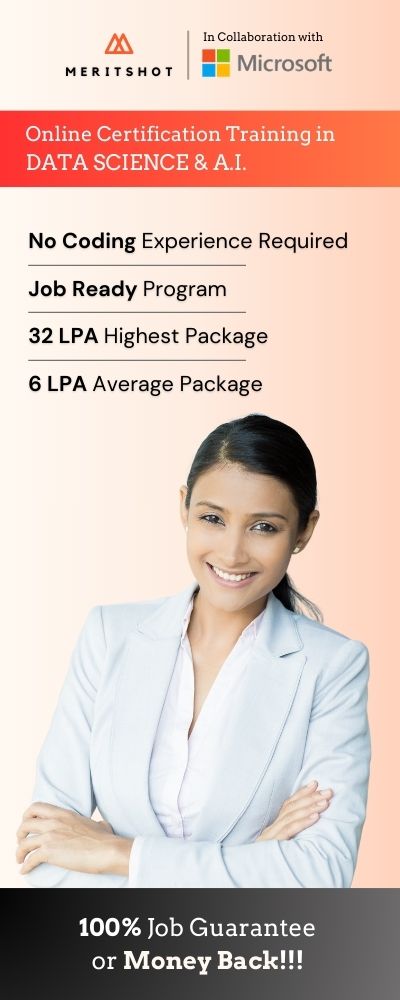