Meritshot Tutorials
- Home
- »
- SQL ANY and ALL
SQL Tutorial
-
SQL SyntaxSQL Syntax
-
SQL ORDER BY ClauseSQL ORDER BY Clause
-
Introduction to SQLIntroduction to SQL
-
SQL SELECT DISTINCTSQL SELECT DISTINCT
-
SQL Logical OperatorsSQL Logical Operators
-
SQL WHERE ClauseSQL WHERE Clause
-
SQL SELECT StatementSQL SELECT Statement
-
SQL DELETE StatementSQL DELETE Statement
-
SQL INSERT INTOSQL INSERT INTO
-
SQL Null ValuesSQL Null Values
-
SQL Update StatementSQL Update Statement
-
SQL Select TopSQL Select Top
-
SQL Aggregate FunctionsSQL Aggregate Functions
-
SQL LIKE and WildcardsSQL LIKE and Wildcards
-
SQL IN and SQL BETWEENSQL IN and SQL BETWEEN
-
SQL JOINSSQL JOINS
-
SQL Group BySQL Group By
-
SQL HavingSQL Having
-
SQL EXISTSSQL EXISTS
-
SQL SELECT INTOSQL SELECT INTO
-
SQL INSERT INTO SELECTSQL INSERT INTO SELECT
SQL ANY and ALL
The ANY and ALL operators in SQL are used to compare a value to a set of values returned by a subquery. They allow for more flexible comparisons and are often used in conjunction with subqueries to filter data based on conditions applied to multiple rows.
Purpose
- ANY: Compares a value to any value in a set returned by a subquery. It returns TRUE if the condition is met for at least one value in the set.
- ALL: Compares a value to all values in a set returned by a subquery. It returns TRUE only if the condition is met for every value in the set.
Syntax
ANY Syntax:
SELECT column_name(s)
FROM table_name
WHERE column_name operator ANY (subquery);
ALL Syntax:
SELECT column_name(s)
FROM table_name
WHERE column_name operator ALL (subquery);
Operators
- Comparison Operators: =, !=, >, <, >=, <=
Example:
1. Using ANY to Compare Values
Find employees with a salary greater than any salary in the HR department.
SELECT employee_id, first_name, salary
FROM employees
WHERE salary > ANY (
SELECT salary
FROM employees
WHERE department_id = (SELECT department_id FROM departments WHERE department_name = ‘HR’)
);
Output:
employee_id | first_name | salary |
1 | Rajesh | 55000 |
2 | Priya | 48000 |
2. Using ALL to Compare Values
Find departments where the maximum salary is greater than all salaries in the HR department.
SELECT department_id, MAX(salary) AS max_salary
FROM employees
GROUP BY department_id
HAVING MAX(salary) > ALL (
SELECT salary
FROM employees
WHERE department_id = (SELECT department_id FROM departments WHERE department_name = ‘HR’)
);
Output:
department_id | max_salary |
1 | 60000 |
3 | 70000 |
3. Using ANY with Subqueries
Retrieve products where the price is lower than at least one product in the Electronics category.
SELECT product_id, product_name, price
FROM products
WHERE price < ANY (
SELECT price
FROM products
WHERE category = ‘Electronics’
);
Output:
product_id | product_name | price |
101 | T-Shirt | 250 |
103 | Mug | 150 |
4. Using ALL with Subqueries
Retrieve customers who have placed orders with amounts greater than all orders placed by customer ID 1.
SELECT customer_id, customer_name
FROM customers
WHERE customer_id NOT IN (
SELECT customer_id
FROM orders
WHERE order_amount < ALL (
SELECT order_amount
FROM orders
WHERE customer_id = 1
)
);
Output:
customer_id | customer_name |
2 | Priya |
3 | Ravi |
Tips to Remember
- Subquery Results: ANY returns TRUE if at least one row in the subquery satisfies the condition, while ALL returns TRUE only if all rows satisfy the condition.
- Usage with Operators: Both ANY and ALL work with comparison operators such as =, !=, >, <, >=, and <=.
- Handling NULL Values: Be cautious with NULL values in subqueries, as comparisons involving NULL can affect the results. For accurate results, ensure subquery data does not contain NULLs or handle them appropriately.
Power of ANY and ALL
- Flexible Comparisons: Provides powerful comparison capabilities for data filtering based on conditions applied to sets of values.
- Enhanced Query Logic: Enables more complex and nuanced data retrieval strategies, enhancing the analytical power of SQL queries.
Frequently Asked Questions
Q1: Can I use ANY with multiple subqueries?
A1: Yes, ANY can be used with multiple subqueries as long as the outer query’s condition is appropriately formulated.
Example:
SELECT product_id, product_name
FROM products
WHERE price < ANY (
SELECT price
FROM products
WHERE category IN (‘Electronics’, ‘Clothing’)
);
Q2: How does ALL handle multiple subqueries?
A2: ALL can handle multiple subqueries if they return a set of values for comparison. Ensure the comparison is valid for all values returned.
Example:
SELECT department_id, MAX(salary) AS max_salary
FROM employees
GROUP BY department_id
HAVING MAX(salary) > ALL (
SELECT AVG(salary)
FROM employees
GROUP BY department_id
);
Q3: Can ANY and ALL be used with JOIN operations?
A3: Yes, both ANY and ALL can be used with JOIN operations to filter data based on the results of joined tables.
Example with ANY:
SELECT e.employee_id, e.first_name
FROM employees e
WHERE e.salary > ANY (
SELECT s.salary
FROM salaries s
WHERE s.department_id = e.department_id
);
Q4: What happens if the subquery returns no rows?
A4: If the subquery returns no rows, ANY will return FALSE and ALL will also return FALSE (as no rows meet the condition).
Q5: Can ANY and ALL be used with NOT?
A5: Yes, NOT ANY and NOT ALL can be used for inverse comparisons, where NOT ANY returns TRUE if no rows satisfy the condition, and NOT ALL returns TRUE if not all rows satisfy the condition.
Example with NOT ANY:
SELECT product_id, product_name
FROM products
WHERE price NOT < ANY (
SELECT price
FROM products
WHERE category = ‘Electronics’
);
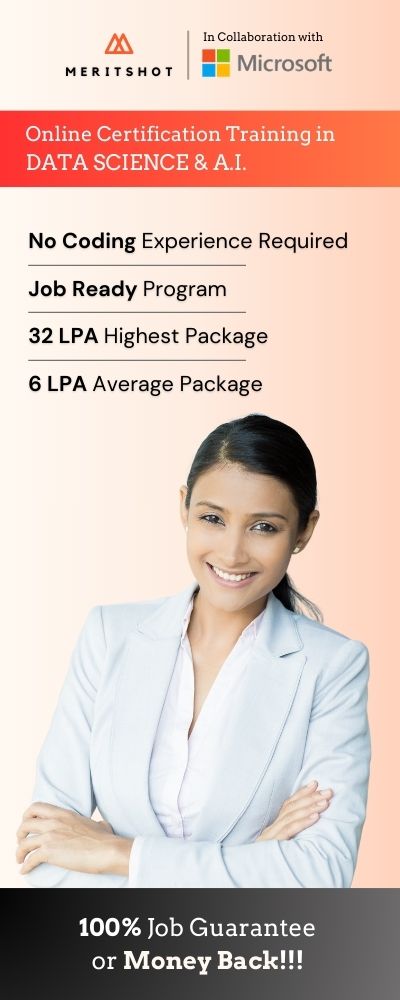