Meritshot Tutorials
- Home
- »
- Setting Up the Environment
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
Setting Up the Environment
Before building and deploying machine learning (ML) models using Flask, it’s essential to set up your development environment properly. This section provides a step-by-step guide for installing Python, Flask, and other necessary libraries for ML projects.
1.4.1 Installing Python
Python is the core programming language for building ML models and deploying them with Flask. Follow these steps to install Python:
- Download Python:
- Go to the official Python website: https://www.python.org/downloads/.
- Download the latest version of Python for your operating system.
- Install Python:
- Run the downloaded installer.
- Important: On the first screen of the installation wizard, check the box that says “Add Python to PATH”. This ensures that Python can be accessed globally from the command line.
- Click on “Install Now” and follow the installation wizard steps.
- Verify Installation:
- Open a terminal or command prompt and type:
python –version
or
python3 –version
- You should see the installed Python version.
1.4.2 Installing Flask
Flask can be installed using Python’s package manager, pip.
- Open Terminal/Command Prompt:
Use the terminal or command prompt to install Flask. - Install Flask:
Type the following command:
pip install flask
- Verify Installation:
After the installation is complete, check if Flask is installed by typing:
python -m flask –version
1.4.3 Installing Necessary Libraries
To work with machine learning models and datasets, you need additional libraries like scikit-learn, pandas, and NumPy. These can be installed using pip.
- scikit-learn: For building and evaluating ML models.
pip install scikit-learn
- pandas: For data manipulation and analysis.
pip install pandas
- NumPy: For numerical computations.
pip install numpy
- Other Libraries: Depending on your project, you may also need libraries like matplotlib, seaborn (for visualization), or joblib (for model serialization). Install them as needed:
pip install matplotlib seaborn joblib
- Install Everything Together (Optional): You can install multiple libraries at once by using:
pip install flask scikit-learn pandas numpy matplotlib seaborn joblib
1.4.4 Creating a Virtual Environment (Optional but Recommended)
Using a virtual environment ensures that your project dependencies are isolated and won’t interfere with other Python projects on your system.
- Create a Virtual Environment:
python -m venv myenv
- Activate the Virtual Environment:
- On Windows:
myenv\Scripts\activate
- On macOS/Linux:
source myenv/bin/activate
- Install Libraries in the Virtual Environment:
After activating the virtual environment, run the pip commands mentioned above to install Flask and other necessary libraries. - Deactivate the Virtual Environment:
When you’re done, deactivate the virtual environment by typing:
deactivate
1.4.5 Testing the Setup
- Create a Simple Flask App:
Save the following code in a file named app.py:
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Flask Environment is Ready!”
if __name__ == ‘__main__’:
app.run(debug=True)
- Run the App:
- Open the terminal, navigate to the directory containing app.py, and run:
python app.py
- Open your browser and go to http://127.0.0.1:5000/. You should see the message “Flask Environment is Ready!”.
Key Tips
- Always use the latest versions of Python and libraries for better performance and compatibility.
- If you encounter issues during installation, ensure that pip is up-to-date:
pip install –upgrade pip
By completing these steps, your environment will be fully set up for deploying ML models with Flask. In the next section, we’ll create our first Flask API for an ML model.
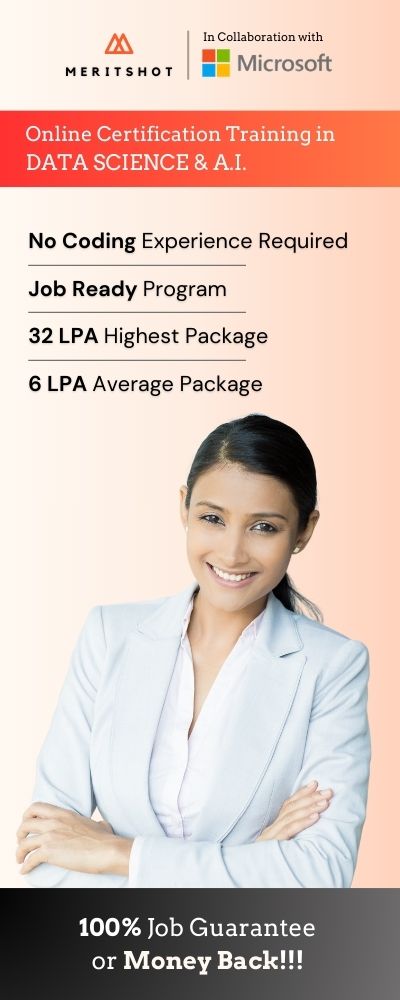