Meritshot Tutorials
- Home
- »
- Scaling ML Model Predictions for Large Inputs
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Scaling ML Model Predictions for Large Inputs
When deploying machine learning models in real-world applications, you might encounter scenarios where the model needs to handle large volumes of input data. Scaling your Flask application to process these inputs efficiently is critical to ensure high performance, low latency, and a seamless user experience.
Challenges of Handling Large Inputs
- Memory Constraints: Large datasets or high-dimensional input data can exceed the memory limits of your server.
- Processing Time: Predictions for large inputs can be computationally expensive, leading to higher response times.
- Concurrency Issues: When multiple users send large inputs simultaneously, it can overwhelm your application.
- Timeout Errors: Large inputs may cause your application to exceed response time limits, leading to client-side timeouts.
Strategies for Scaling Predictions
- Batch Processing:
- Instead of processing one input at a time, group multiple inputs into batches and process them together. This reduces overhead and improves efficiency.
- Example: Instead of predicting for one row of data at a time, process 100 rows in a single request.
- Asynchronous Processing:
- Use asynchronous APIs to handle predictions. For large inputs, the client can send a request, and your application can process it in the background and return the result once it’s ready.
- Tools like Celery or Redis Queue can help implement asynchronous workflows in Flask.
- Pagination:
- For datasets too large to process at once, paginate the input and process it in smaller chunks. For instance, handle 1,000 rows at a time.
- Preprocessing on the Client Side:
- Encourage users to preprocess and filter their data on the client side before sending it to your API. For example, users can send only the features required for the model rather than the entire dataset.
- Using a High-Performance Server:
- Deploy your Flask application on a high-performance server with sufficient CPU, GPU (if needed), and memory resources to handle large data inputs efficiently.
- Optimize the Model:
- Use lightweight models (e.g., a distilled version of a deep learning model) to reduce computational requirements for large inputs.
- Quantization and pruning techniques can make models faster without significant loss of accuracy.
- Parallel Processing:
- Utilize Python libraries like joblib or multiprocessing to process data in parallel. This approach leverages multiple cores of your server for faster computation.
Implementing Scalable APIs in Flask
- Input Size Validation:
- Set a limit on the size of input data that your API can handle to avoid overwhelming the server. Return an appropriate error if the input exceeds the limit.
- Stream Data:
- For very large files or datasets, use data streaming techniques to process the input in smaller parts rather than loading the entire dataset into memory.
- Efficient Data Formats:
- Encourage users to send data in efficient formats like CSV, JSON, or Parquet, depending on the use case. Avoid bulky or unstructured data formats.
Practical Example: Batch Processing with Flask
For instance, consider a machine learning model that predicts the house prices for multiple inputs:
- Accept a JSON array of input features from the user.
- Process the inputs in batches (e.g., 100 rows at a time).
- Return predictions for the entire dataset after processing all batches.
Performance Monitoring and Optimization
- Monitor Resource Usage:
- Use tools like Prometheus or Grafana to track CPU, memory, and latency metrics. Identify bottlenecks and scale resources accordingly.
- Enable Load Balancing:
- Distribute incoming requests across multiple server instances using load balancers like NGINX or AWS Elastic Load Balancer.
- Caching Predictions:
For frequently requested inputs, implement caching mechanisms (e.g., Redis) to return results instantly without recomputing.
Frequently Asked Questions
- How do I process large datasets without overloading the server?
- Use batch processing or paginate the data. Consider asynchronous processing for very large datasets.
- Can Flask handle large inputs efficiently?
- Flask can handle large inputs if combined with techniques like batching, streaming, and scaling infrastructure.
- What happens if a user sends too much data?
- Implement input size validation to reject excessively large requests and notify users with appropriate error messages.
- How do I ensure predictions remain fast for large inputs?
- Optimize your model, use parallel processing, and consider deploying the application on a server with sufficient resources.
- Should I preprocess data on the client or server side?
- If possible, encourage clients to preprocess data before sending it to reduce server-side load.
- What tools can help scale Flask applications?
- Use tools like Celery for asynchronous tasks, NGINX for load balancing, and Redis for caching to improve scalability.
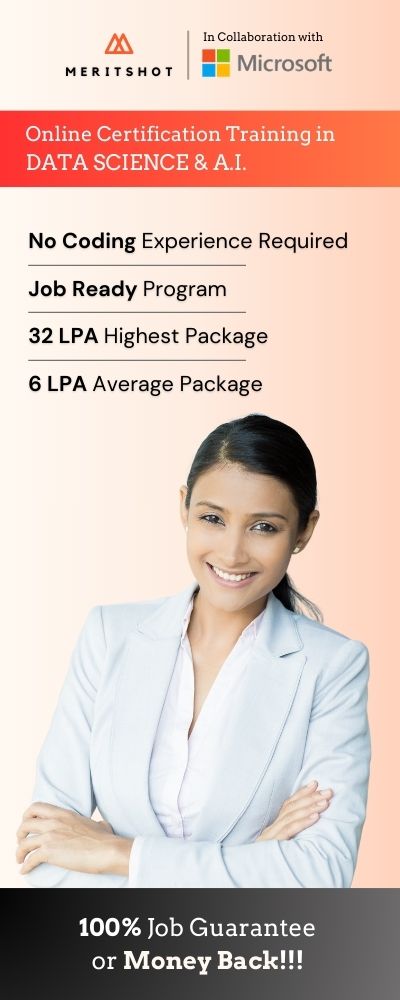