Meritshot Tutorials
- Home
- »
- Saving the Trained Model
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
Saving the Trained Model
After training a machine learning model, the next step is to save it so it can be loaded and used in different environments, such as a Flask application for deployment. Python provides libraries like pickle and joblib to save and load models efficiently.
Why Save a Model?
- Reusability: Saves time by avoiding retraining the model every time it’s needed.
- Deployment: Makes the model portable and deployable across different environments.
- Consistency: Ensures the same trained model is used in production, preventing variations caused by retraining.
Tools for Saving Models
- Using pickle
pickle is a Python library for serializing (saving) and deserializing (loading) objects, including machine learning models.
- Using joblib
joblib is optimized for handling large objects like NumPy arrays, making it faster and more memory-efficient than pickle for saving models.
Steps to Save the Model
Step 1: Using pickle
To save the trained model using pickle:
import pickle
# Save the model
with open(“house_price_model.pkl”, “wb”) as file:
pickle.dump(model, file)
print(“Model saved as ‘house_price_model.pkl'”)
Step 2: Using joblib
To save the model using joblib:
from joblib import dump
# Save the model
dump(model, “house_price_model.joblib”)
print(“Model saved as ‘house_price_model.joblib'”)
Steps to Verify the Saved Model
Reload the Model
To ensure the model is saved correctly, load it back into Python and test it with sample data:
Using pickle:
# Load the model
with open(“house_price_model.pkl”, “rb”) as file:
loaded_model = pickle.load(file)
# Test the loaded model
sample_input = np.array([[3.87, 29.0, 6.9841, 1.0238, 3.1400, 37.88, -121.23]])
sample_prediction = loaded_model.predict(sample_input)
print(f”Predicted House Price (loaded model): ${sample_prediction[0] * 1000:.2f}”)
Using joblib:
from joblib import load
# Load the model
loaded_model = load(“house_price_model.joblib”)
# Test the loaded model
sample_prediction = loaded_model.predict(sample_input)
print(f”Predicted House Price (loaded model): ${sample_prediction[0] * 1000:.2f}”)
File Naming Tips
- Use descriptive names like model_name_version.pkl or model_name_version.joblib.
- Maintain a versioning system if you frequently update the model, e.g., house_price_model_v1.pkl.
Best Practices for Saving Models
- Test Before Saving: Ensure the model performs well on the test set.
- Include Metadata: Save additional information like preprocessing steps, feature names, and version numbers.
- Secure Storage: Use secure and accessible storage options (e.g., AWS S3, Google Drive) for production models.
- Environment Compatibility: Ensure the saved model is compatible with the deployment environment.
Frequently Asked Questions
- Q: When should I use joblib over pickle?
A: Use joblib when dealing with large models or datasets, as it is faster and more efficient for handling NumPy arrays. - Q: Can I save models trained with libraries like TensorFlow or PyTorch using pickle or joblib?
A: No, TensorFlow and PyTorch have their own methods (.save() and torch.save() respectively) for saving models. - Q: What happens if the environment changes after saving the model?
A: The model may not load properly. Ensure consistent versions of libraries used during training and deployment. - Q: Where should I store the saved models in production?
A: Use cloud storage (e.g., AWS S3, Google Cloud) or secure databases for easy access and reliability. - Q: Can I save preprocessing steps along with the model?
A: Yes, you can save preprocessing objects (like StandardScaler) in the same way, ensuring consistency during deployment.
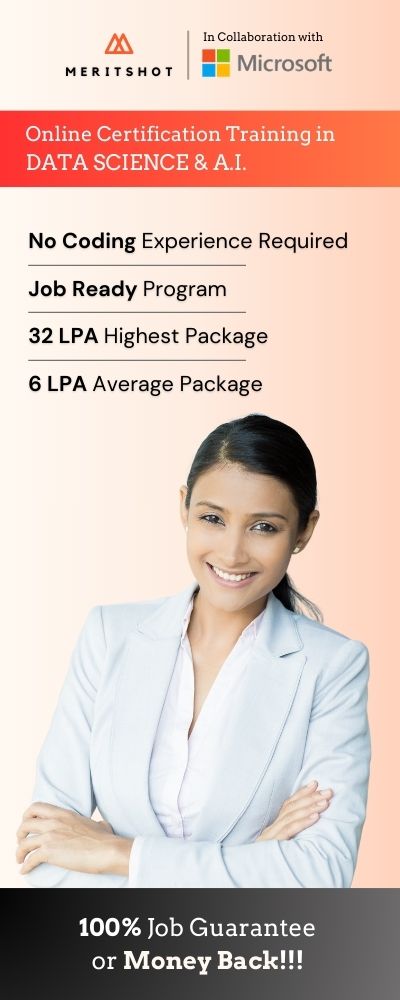