Meritshot Tutorials
- Home
- »
- Returning Predictions as JSON or HTML
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Serving JSON ResponsesServing JSON Responses
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Returning Predictions as JSON or HTML
After receiving user input and making a prediction with your machine learning model, it’s essential to send the prediction back to the user in a user-friendly format. In Flask, you can return predictions either as a JSON response (commonly used for APIs) or as HTML content (for web pages). Let’s look at how to return predictions in both formats.
- Returning Predictions as JSON
Returning predictions in JSON format is ideal for API-based applications where the client (e.g., a front-end web application or mobile app) makes requests to the Flask backend. JSON is lightweight and easy to parse, making it a popular choice for web services and APIs.
- Flask’s jsonify Function: Flask provides the jsonify() function to return responses in JSON format. It automatically converts Python data structures (such as dictionaries or lists) into JSON.
Example: Returning a prediction as JSON
from flask import Flask, request, jsonify
import pickle
import numpy as np
app = Flask(__name__)
# Load the trained model (make sure the model file is available)
with open(‘model.pkl’, ‘rb’) as model_file:
model = pickle.load(model_fil
@app.route(‘/predict’, methods=[‘POST’])
def predict():
# Get user input
feature1 = float(request.form[‘feature1’])
feature2 = float(request.form[‘feature2’])
# Prepare the input for the model
user_input = np.array([[feature1, feature2]])
# Make the prediction
prediction = model.predict(user_input)
# Create a dictionary to hold the response data
result = {
‘prediction’: prediction[0]
}
# Return the prediction as a JSON response
return jsonify(result)
if __name__ == “__main__”:
app.run(debug=True)
In this example:
- The jsonify() function converts the Python dictionary result into a JSON object.
- The response will look like this:
{
“prediction”: 42.5
}
The client-side application (JavaScript, mobile app, etc.) can then easily parse the JSON response and display the prediction.
2. Returning Predictions as HTML
Returning predictions as HTML is more suitable when you want to display the prediction directly on a web page, allowing the user to see the result without needing additional client-side parsing.
Example: Returning a prediction in HTML format
from flask import Flask, request, render_template
import pickle
import numpy as np
app = Flask(__name__)
# Load the trained model (make sure the model file exists)
with open(‘model.pkl’, ‘rb’) as model_file:
model = pickle.load(model_file)
@app.route(‘/’)
def home():
return render_template(‘index.html’) # Show the form to the user
@app.route(‘/predict’, methods=[‘POST’])
def predict():
# Get user input
feature1 = float(request.form[‘feature1’])
feature2 = float(request.form[‘feature2’])
# Prepare the input for the model
user_input = np.array([[feature1, feature2]])
# Make the prediction
prediction = model.predict(user_input)
# Return the prediction as part of the rendered HTML page
return render_template(‘result.html’, prediction=prediction[0])
if __name__ == “__main__”:
app.run(debug=True)
In this example:
- After making the prediction, we pass the prediction to a template (result.html) to display it.
- The result.html file can include the prediction dynamically:
<h1>Prediction Result: {{ prediction }}</h1>
The result will be displayed on the page as a simple heading:
Prediction Result: 42.5
3. Which Format Should I Choose?
- JSON: Use JSON if you’re building an API that will be consumed by other applications or services (e.g., a mobile app or JavaScript frontend). JSON is lightweight, and many front-end libraries can easily parse JSON data.
- HTML: Use HTML if you are building a web application where you want to display the prediction directly to the user in a readable format. HTML is appropriate for Flask apps that involve user interactions on a webpage.
Frequently Asked Questions
- Can I return both JSON and HTML from the same route?
- Answer: Yes, you can use conditional logic to return different formats based on the client request. For example, if the client sends an Accept: application/json header, you can return JSON, and if it requests HTML, you can render an HTML template.
- How can I handle errors when returning predictions?
- Answer: You can handle errors by checking the validity of user inputs before making predictions. If any error occurs, you can return a JSON response with an error message or render an error page in HTML.
return jsonify({‘error’: ‘Invalid input’})
- Can I return multiple predictions at once?
- Answer: Yes, you can modify the model to accept multiple inputs and return multiple predictions. In that case, the JSON response would include an array of predictions.
- How do I ensure that the prediction response is accurate and fast?
- Answer: To ensure accuracy and speed, you should validate user inputs and optimize your machine learning model. Additionally, you can implement caching mechanisms if the predictions are computationally expensive.
- Can I return predictions as both JSON and HTML for different types of users?
- Answer: Yes, you can implement both JSON and HTML responses in your Flask app based on the client’s needs. You can set up routes that return JSON for API consumption and HTML for web users.
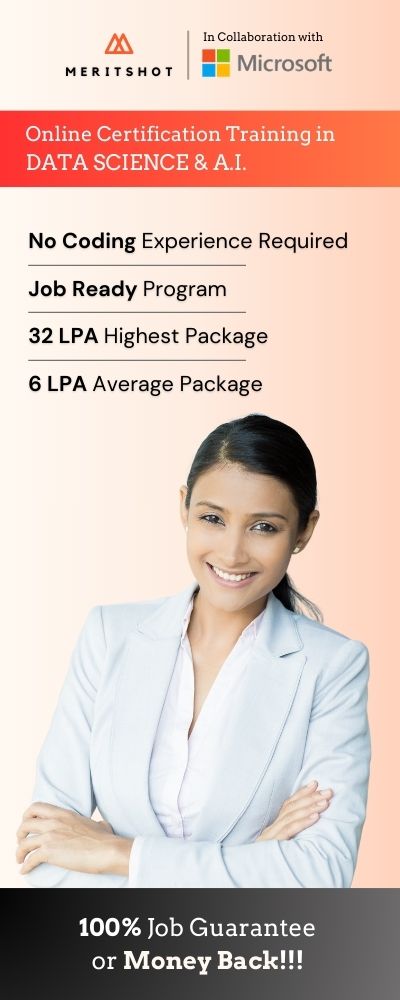