Meritshot Tutorials
- Home
- »
- R-Operators
R Tutorial
-
R-OverviewR-Overview
-
R Basic SyntaxR Basic Syntax
-
R Data TypesR Data Types
-
R-Data StructuresR-Data Structures
-
R-VariablesR-Variables
-
R-OperatorsR-Operators
-
R-StringsR-Strings
-
R-FunctionR-Function
-
R-ParametersR-Parameters
-
Arguments in R programmingArguments in R programming
-
R String MethodsR String Methods
-
R-Regular ExpressionsR-Regular Expressions
-
Loops in R-programmingLoops in R-programming
-
R-CSV FILESR-CSV FILES
-
Statistics in-RStatistics in-R
-
Probability in RProbability in R
-
Confidence Interval in RConfidence Interval in R
-
Hypothesis Testing in RHypothesis Testing in R
-
Correlation and Covariance in RCorrelation and Covariance in R
-
Probability Plots and Diagnostics in RProbability Plots and Diagnostics in R
-
Error Matrices in RError Matrices in R
-
Curves in R-Programming LanguageCurves in R-Programming Language
R-Operators
In this article, you will learn about different R operators with the help of examples.
An R operator is a symbol or keyword used in the R programming language to perform various operations on data values or variables. These operators manipulate data or evaluate relationships between values, enabling a range of actions from arithmetic computations to logical evaluations.
R has many operators to carry out different mathematical and logical operations. Operators perform tasks including arithmetic, logical and bitwise operations.
Type of operators in R
Operators in R can mainly be classified into the following categories:
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Assignment Operators
- Miscellaneous Operators
These operators form the building blocks for computations and decision-making in R scripts and programs.
Let’s Discuss these Operators one-by-one:
Arithmetic Operators in R:
Arithmetic operators in ‘R’ are used to perform mathematical operations on numbers (or variables representing numbers). Below is a detailed explanation of each
arithmetic operator, including syntax and examples.
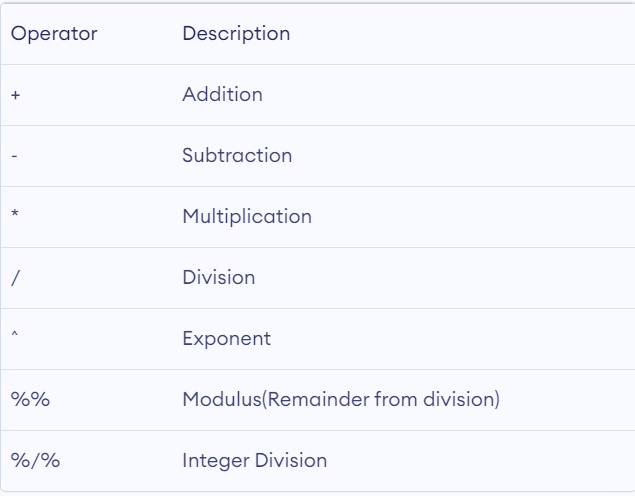
Let’s look at an example illustrating the use of the above operators:
1. Addition (+)
The addition operator adds two values.
Syntax:
result <- value1 + value2
Example:
x <- 5
y <- 3
sum <- x + y # sum = 8
2. Subtraction (–)
The subtraction operator subtracts one value from another
Syntax:
result <- value1 – value2
Example:
x <- 10
y <- 4
difference <- x – y # difference = 6
3. Multiplication (*)
The multiplication operator multiplies two values.
Syntax:
result <- value1 * value2
Example:
x <- 7
y <- 2
product <- x * y # product = 14
4. Division (/)
The division operator divides one value by another.
Syntax:
result <- value1 / value2
Example:
x <- 15
y <- 3
quotient <- x / y # quotient = 5
5. Exponentiation (^ or **)
The exponentiation operator raises one value to the power of another.
Syntax:
result <- base ^ exponent
Example:
x <- 2
y <- 3
power <- x ^ y # power = 8 (2 raised to the power 3)
6. Modulus (%%)
The modulus operator returns the remainder of the division between two numbers
Syntax:
remainder <- value1 %% value2
Example:
x <- 17
y <- 5
remainder <- x %% y # remainder = 2 (17 divided by 5 leaves remainder 2)
7. Integer Division (%/%)
The integer division operator returns the quotient of the division, discarding the fractional part.
Syntax:
Integer_quotient <- value1 %/% value2
Example:
x <- 17
y <- 5
integer_quotient <- x %/% y
# integer_quotient = 3 (17 divided by 5 equals 3 with remainder discarded)
Combining Arithmetic Operators
You can combine multiple arithmetic operators in a single expression. Operations follow standard precedence rules (order of operations):
- Parentheses ( )
- Exponentiation ^
- Multiplication and Division *, /, %%, %/%
- Addition and Subtraction +, –
Example:
x <- 10
y <- 2
z <- 3
result <- x + y * z – (x %% z) # result = 10 + 2 * 3 – (10 %% 3) = 16 – 1 = 15
Relational Operators:
Relational (or comparison) operators in R are used to compare two values, vectors, or other data structures. The result of a comparison is either TRUE or FALSE, which can be used in control flow (e.g., if statements) or logical operations.
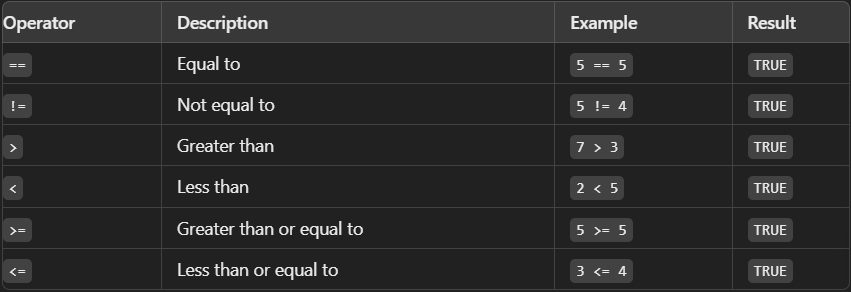
Let’s see an example for this:
1. Equal To (==)
This operator checks if two values are equal. It returns ‘TRUE’ if both values are identical, otherwise ‘FALSE’.
Syntax:
result <- value1 == value2
Example:
x <- 10
y <- 10
is_equal <- x == y # TRUE, because 10 is equal to 10
2. Not Equal To (!=)
This operator checks if two values are not equal. It returns TRUE if the values are different, otherwise FALSE.
Syntax:
result <- value1 != value2
Example 2: Basic Arithmetic Operation
x <- 5
y <- 7
is_not_equal <- x != y # TRUE, because 5 is not equal to 7.
3. Greater Than (>)
This operator checks if one value is greater than another. It returns TRUE if the left value is larger than the right value.
Syntax:
result <- value1 > value2
Example:
x <- 10
y <- 5
is_greater <- x > y # TRUE, because 10 is greater than 5
1. Less Than (<)
This operator checks if one value is less than another. It returns TRUE if the left value is smaller than the right value.
Syntax:
result <- value1 < value2
Example:
x <- 2
y <- 4
is_less <- x < y # TRUE, because 2 is less than 4.
5. Greater Than or Equal To (>=)
This operator checks if one value is greater than or equal to another. It
returns TRUE if the left value is either greater than or equal to the right value.
Syntax:
result <- value1 >= value2
Example:
x <- 5
y <- 5
is_greater_equal <- x >= y # TRUE, because 5 is equal to 5
Less Than or Equal To (<=)
This operator checks if one value is less than or equal to another. It returns TRUE if the left value is either less than or equal to the right value.
Syntax:
result <- value1 <= value2
Example:
x <- 3
y <- 5
is_less_equal <- x <= y # TRUE, because 3 is less than 5
These relational operators are crucial in data analysis, control flow, and logical
reasoning in R. They provide the foundation for making comparisons and decisions based on data values
Logical Operators
Logical operators in R are used to combine or negate logical values (TRUE or FALSE). They play a key role in decision-making and control flow. Logical operators are often used with relational operators to create more complex conditional statements.
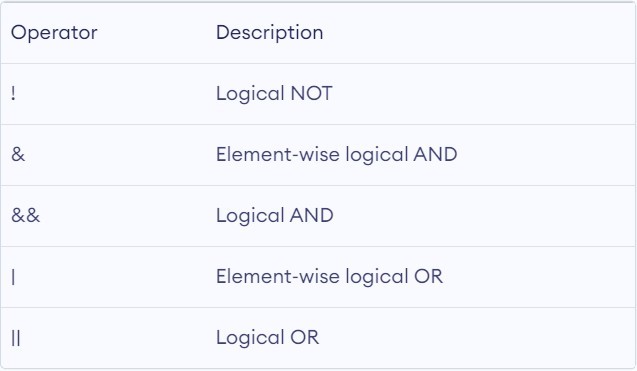
Let’s see an example for this:
1. NOT Operator (!)
The ! operator is used for logical negation. It takes a logical value (or vector of logical values) and returns the opposite. If the value is TRUE, it returns FALSE, and vice versa.
Syntax:
result <- !logical_value
Example:
x <- TRUE
result <- !x # result = FALSE
2. Element-wise Logical AND (&)
The & operator performs element-wise AND between two logical vectors. It returns TRUE only if both corresponding elements are TRUE; otherwise, it returns FALSE.
Syntax:
result <- vector1 & vector2
Example:
x <- c(TRUE, FALSE, TRUE)
y <- c(TRUE, TRUE, FALSE)
result <- x & y # result = c(TRUE, FALSE, FALSE)
Here, the operation is applied element by element:
- TRUE & TRUE returns TRUE
- FALSE & TRUE returns FALSE
- TRUE & FALSE returns FALSE
3. Logical AND (&&)
The && operator is used for logical AND but only evaluates the first element of each vector. It is used in situations where you only care about the first element of the
vectors.
Syntax:
result <- value1 && value2
Example:
x <- c(TRUE, FALSE, TRUE)
y <- c(TRUE, TRUE, FALSE)
result <- x && y # result = TRUE (only the first elements are compared)
4. Element-wise Logical OR (|)
The | operator performs element-wise OR between two logical vectors. It returns TRUE if at least one of the corresponding elements is TRUE.
Syntax:
result <- vector1 | vector2
Example:
x <- c(TRUE, FALSE, TRUE)
y <- c(FALSE, TRUE, FALSE)
result <- x | y # result = c(TRUE, TRUE, TRUE)
Here, the operation is applied element by element:
- TRUE | FALSE returns TRUE
- FALSE | TRUE returns TRUE
- TRUE | FALSE returns TRUE
5. Logical OR (||)
The || operator is used for logical OR but only evaluates the first element of each vector. It is typically used in conditional statements.
Example:
x <- 7
y <- 12
if (x > 5 && y < 20) {
print(“Both conditions are true”)
}
In this example, both x > 5 and y < 20 are TRUE, so the message is printed.a
Examples Summary
# NOT operator
!TRUE # FALSE
# Element-wise logical AND
c(TRUE, FALSE, TRUE) & c(TRUE, TRUE, FALSE)
# c(TRUE, FALSE, FALSE)
# Logical AND (first element only)
TRUE && FALSE
# FALSE
# Element-wise logical OR
c(TRUE, FALSE, TRUE) | c(FALSE, TRUE, FALSE)
# c(TRUE, TRUE, TRUE)
# Logical OR (first element only)
TRUE || FALSE # TRUE
These operators are frequently used in data analysis, control structures, and logical conditions.
Note: The long form of AND and OR (&& and ||) are preferred for if statements as the short form can produce a vector value.
- Assignment Operators
Assignment operators in R are used to assign values to variables. There are different types of assignment operators, and they serve various purposes. The most commonly used are <-, ->, and =
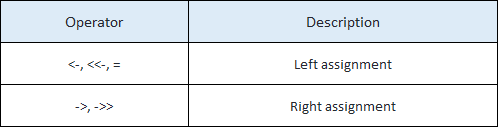
- <- assigns a value to a variable from right to
- -> assigns a value to a variable left to
- <<- is a global version of <-.
- ->> is a global version of ->.
- = works the same way as <-, but it’s use is discouraged.
Let’s See an Example for this:
1. Leftward Assignment (<-)
The most common assignment operator in R is the leftward arrow (<-). It assigns the value on the right-hand side to the variable on the left-hand side.
Syntax:
variable <- value
Example:
x <- 10
This assigns the value 10 to the variable x.
2. Rightward Assignment (->)
The rightward assignment operator (->) assigns the value on the left-hand side to the variable on the right-hand side.
Syntax:
value -> variable
Example:
10 -> x
This also assigns the value 10 to the variable x, but uses rightward assignment.
3. Assignment Using =
The = operator can also be used for leftward assignment, although it is less commonly used than <-.
Syntax:
variable = value
Example:
x <- c(TRUE, FALSE, TRUE)
y <- c(FALSE, TRUE, FALSE)
result <- x || y
# result = TRUE (only the first elements are compared).
Syntax:
result <- value1 || value2
Example:
x = 20
This assigns the value 20 to the variable x.
Note: While = can be used for assignment, it is generally avoided in favor
of <- because = is also used for arguments in function calls. Using <- makes the code more readable and avoids confusion.
4. Global Assignment (<<- and ->>)
The <<- and ->> operators perform assignments in the global environment (i.e., outside of the current function or scope). These are used when a variable needs to be updated globally from within a function.
- <<- performs a global leftward
- ->> performs a global rightward
Example of Global Assignment:
f <- function() {
x <<- 5 # x is assigned globally
}
f()
# Call the function
print(x) # Output: 5
In this case, x is updated globally, even though the assignment is made inside the function f().
- Miscellaneous Operators in R
Miscellaneous operators in R perform specialized operations that go beyond arithmetic, relational, and logical operators. These include sequence generation, matrix multiplication, membership testing, and others that provide additional functionality in R programming.
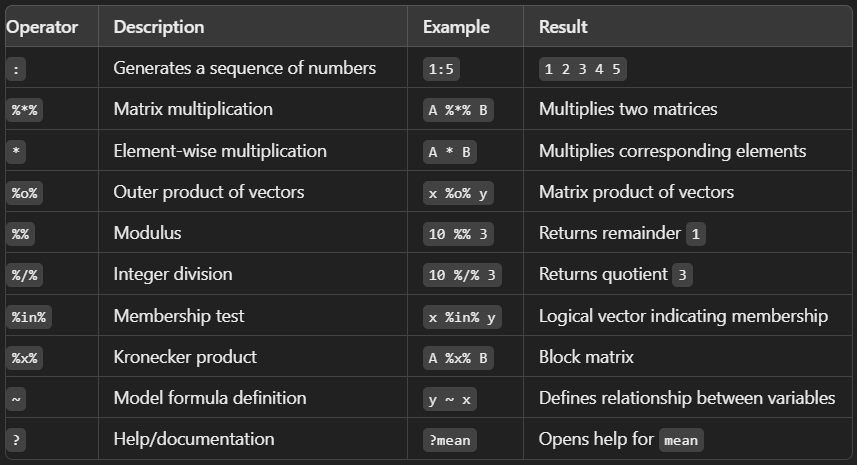
Let’s See the examples of these operators:
- Colon Operator (:)
Creates a sequence of numbers from the left argument to the right one.
Syntax:
start:end
Example:
seq <- 1:5
# Creates sequence 1, 2, 3, 4, 5
2. In Operator (%in%)
Returns TRUE if the left argument is found in the vector or set on the right.
Syntax:
value %in% vector
Example:
result <- 2 %in% c(1, 2, 3)
# result = TRUE
3. Matrix Multiplication Operator (%*%)
Performs matrix multiplication between two matrices.
Syntax:
matrix1 %*% matrix2
Example:
A <- matrix(c(1, 2, 3, 4), nrow = 2)
B <- matrix(c(5, 6, 7, 8), nrow = 2)
result <- A %*% B # Performs matrix multiplication
4. Element-wise Multiplication (*)
Multiplies corresponding elements of two vectors, matrices, or arrays.
Syntax:
vector1 * vector2
Example:
A <- c(1, 2, 3) B <- c(4, 5, 6) result <- A * B
# result = c(4, 10, 18)
5. Modulus Operator (%%)
Returns the remainder of the division of the left argument by the right argument.
Syntax:
value1 %% value2
Example:
result <- 10 %% 3
# result = 1
6. Integer Division Operator (%/%)
Returns the integer quotient of the division of the left argument by the right, discarding the fractional part.
Syntax:
value1 %/% value2
Example:
result <- 10 %/% 3
# result = 3
Syntax:
vector1 * vector2
Example:
result <- 10 %/% 3
# result = 3
7. Outer Product Operator (%o%)
Computes the outer product of two vectors.
Syntax:
vector1 %o% vector2
Example:
x <- c(1, 2)
y <- c(3, 4)
result <- x %o% y
# result = matrix(c(3, 4, 6, 8), nrow = 2)
8, Kronecker Product Operator (%x%)
Description: Computes the Kronecker product of two matrices.
Syntax:
matrix1 %x% matrix2
Example:
A <- matrix(c(1, 2), nrow = 1)
B <- matrix(c(0, 1, 1, 0), nrow = 2) result <- A %x% B
# result = matrix(c(0, 1, 0, 2, 1, 0, 2, 0), nrow = 2)
9. Tilde Operator (~)
Description: Defines a model formula, relating the dependent variable to one or more independent variables in statistical modeling.
Syntax:
dependent ~ independent
Example:
model <- lm(Sepal.Length ~ Sepal.Width, data = iris)
10. Help Operator (?)
Description: Retrieves documentation for a specified function or topic.
Syntax:
?function_name
Example:
?mean # Opens help for the mean function
These operators provide diverse functionality, from sequence generation to matrix operations and model definitions.
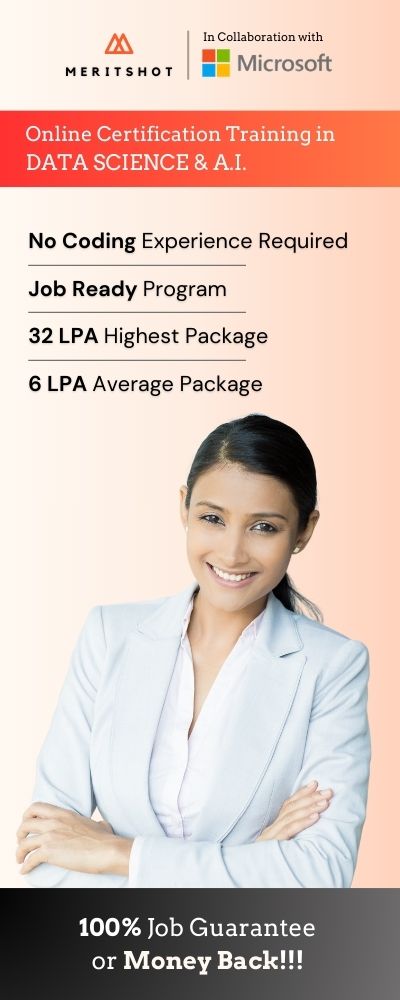