Meritshot Tutorials
- Home
- »
- R-Function
R Tutorial
-
R-OverviewR-Overview
-
R Basic SyntaxR Basic Syntax
-
R Data TypesR Data Types
-
R-Data StructuresR-Data Structures
-
R-VariablesR-Variables
-
R-OperatorsR-Operators
-
R-StringsR-Strings
-
R-FunctionR-Function
-
R-ParametersR-Parameters
-
Arguments in R programmingArguments in R programming
-
R String MethodsR String Methods
-
R-Regular ExpressionsR-Regular Expressions
-
Loops in R-programmingLoops in R-programming
-
R-CSV FILESR-CSV FILES
-
Statistics in-RStatistics in-R
-
Probability in RProbability in R
-
Confidence Interval in RConfidence Interval in R
-
Hypothesis Testing in RHypothesis Testing in R
-
Correlation and Covariance in RCorrelation and Covariance in R
-
Probability Plots and Diagnostics in RProbability Plots and Diagnostics in R
-
Error Matrices in RError Matrices in R
-
Curves in R-Programming LanguageCurves in R-Programming Language
R-Function
A function is a set of statements organized together to perform a specific task. R has a large number of in-built functions and the user can create their own functions.
In R, a function is an object so the R interpreter is able to pass control to the function, along with arguments that may be necessary for the function to accomplish the actions.
The function in turn performs its task and returns control to the interpreter as well as any result which may be stored in other objects.
Function Definition
An R function is created by using the keyword function. The basic syntax of an R function definition is as follows –
For example,
function_name <- function(arg_1, arg_2, …) {
#Function body
return(result)
}
Functions are used to avoid repeating the same task and to reduce complexity. To understand and maintain our code, we logically break it into smaller parts using the function. A function should be
- Written to carry out a specified
- May or may not have arguments
- Contain a body in which our code is
- May or may not return one or more output
Components of Functions
There are four components of function, which are as follows:
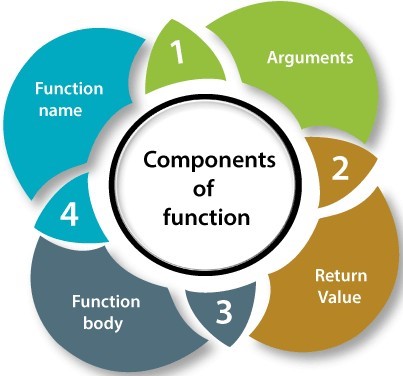
- Function Name − This is the actual name of the It is stored in R environment as an object with this name.
- Arguments − An argument is a placeholder. When a function is invoked, you pass a value to the argument. Arguments are optional; that is, a function may contain no arguments. Also arguments can have default values.
- Function Body − The function body contains a collection of statements that defines what the function
- Return Value − The return value of a function is the last expression in the function body to be evaluated.
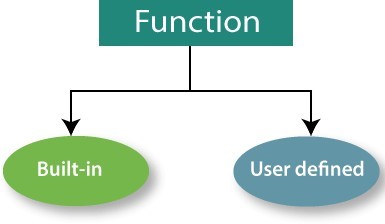
R has many in-built functions which can be directly called in the program without defining them first. We can also create and use our own functions referred as user defined functions.
Built-in Function
Simple examples of in-built functions
are seq(), mean(), max(), sum(x) and paste(…) etc. They are directly called by user written programs
# Create a sequence of numbers from 32 to 44.
print(seq(32,44))
# Find mean of numbers from 25 to 82.
print(mean(25:82))
# Find sum of numbers frm 41 to 68.
print(sum(41:68))
When we execute the above code, it produces the following result –
32 33 34 35 36 37 38 39 40 41 42 43 44
53.5
1526
User-defined Function
We can create user-defined functions in R. They are specific to what a user wants and once created they can be used like the built-in functions. Below is an example of how a function is created and used.
# Create a function to print squares of numbers in sequence.
new.function <- function(a) { for(i in 1:a) {
b <- i^2
print(b)
}
}
Calling a Function
# Create a function to print squares of numbers in sequence.
new.function <- function(a) {
for(i in 1:a) { b <- i^2
print(b)
}
}
# Call the function new.function supplying 6 as an argument.
new.function(6)
When we execute the above code, it produces the following result –
1
4
9
16
25
36
Calling a Function without an Argument
# Create a function without an argument.
new.function <- function() {
for(i in 1:5) {
print(i^2)
}
}
# Call the function without supplying an argument.
new.function()
When we execute the above code, it produces the following result – 1
4
9
16
25
Calling a Function with Argument Values (by position and by name)
The arguments to a function call can be supplied in the same sequence as defined in the function or they can be supplied in a different sequence but assigned to the names of the arguments.
# Create a function with arguments. new.function <- function(a,b,c) {
result <- a * b + c
print(result)
}
# Call the function by position of arguments.
new.function(5,3,11)
# Call the function by names of the arguments.
new.function(a = 11, b = 5, c = 3)
When we execute the above code, it produces the following result –
26
28
Calling a Function with Default Argument
We can define the value of the arguments in the function definition and call the function without supplying any argument to get the default result. But we can also call such functions by supplying new values of the argument and get non default result.
# Create a function with arguments. new.function <- function(a = 3, b = 6) {
result <- a * b
print(result)
}
# Call the function without giving any argument.
new.function()
# Call the function with giving new values of the argument.
new.function(9,5)
When we execute the above code, it produces the following result – 18
45
Anonymous Functions in R
An anonymous function is a function that is defined without a name. These functions are often used when the function is only needed for a short period of time, or in contexts where you don’t need to reuse the function elsewhere in your code.
In R, you can create anonymous functions using the function() keyword without assigning them to a variable (which would be naming them). Anonymous functions are often used as arguments to other functions, such as
in apply functions (lapply(), sapply(), etc.) or other functional programming contexts.
Syntax:
function(arg1, arg2, …) {
# Function body
}
Usage Example:
- Anonymous Function within lapply(): Let’s say you want to square each element of a Instead of defining a named function, you can use an anonymous
function inside lapply(). numbers <- list(1, 2, 3, 4)
squared <- lapply(numbers, function(x) x^2)
print(squared)
# Outputs: list(1, 4, 9, 16)
- Anonymous Function as Argument: If you are performing a simple operation
that doesn’t require a full named function, you can pass an anonymous function directly.
result <- sapply(1:5, function(x) x * 2)
print(result)
# Outputs: 2 4 6 8 10
Why Use Anonymous Functions?
- Short-Lived Operations: Anonymous functions are ideal for one-off tasks where you don’t need to reuse the
- Concise Code: They allow you to write cleaner, more concise code, especially when working with functions like apply().
- Flexibility: Useful in functional programming paradigms, where functions can be passed as arguments or returned from other
When to Use Anonymous Functions:
- For small, single-use
- When passing a function as an argument to higher-order functions like apply(), Map(),
- To avoid polluting the global environment with extra named
Calling A Function in R:
Once a function is defined, you can call it by using the function name followed by parentheses (). Inside the parentheses, you pass the required arguments (if any) to the function.
Basic Syntax for Calling a Function:
function_name(arguments)
Example 1: Simple Function Call
Here’s a simple example where we define a function that adds two numbers and then call it:
# Define a function
add_numbers <- function(a, b) {
return(a + b)
}
# Call the function with arguments
result <- add_numbers(5, 3)
print(result) # Outputs: 8
In this example, we pass the values 5 and 3 as arguments to the function add_numbers(), which returns their sum.
Calling a Function with Default Arguments
If a function has default arguments, you can call it without passing all the arguments. The function will use the default values for the missing arguments.
Example 1: Simple Function Call
# Define a function with a default value for ‘b’ multiply <- function(a, b = 2) {
return(a * b)
}
# Call the function with only one argument
result1 <- multiply(4) # Uses default value of b (2)
print(result1) # Outputs: 8
# Call the function with both arguments
result2 <- multiply(4, 3)
# Overrides default value of b
print(result2) # Outputs: 12
In this case, when you call multiply(4), it uses the default value b = 2. When you call multiply(4, 3), the default is overridden, and b is set to 3.
Calling a Function Without Arguments
Some functions may not take any arguments. You can call these simply with empty parentheses.
Example:
# Define a function with no arguments greet <- function() {
return(“Hello, Meritshot!”) }
# Call the function
message <- greet() print(message)
# Outputs: “Hello, Meritshot!”
Passing Named Arguments
You can also call a function by specifying arguments by name, regardless of their order in the function definition.
Example:
# Define a function with two arguments
introduce <- function(name, age) {
return(paste(“My name is”, name, “and I am”, age, “years old.”))
} # Call the function using named arguments
message <- introduce(age = 22, name = “Aaditya”)
print(message) # Outputs: “My name is Aaditya and I am 22 years old.”
Here, by using named arguments (age = 22, name = “Aaditya”), you can pass values in any order.
Calling a Function with Variable Number of Arguments
In R, you can create functions that accept a variable number of arguments using….. This allows you to
pass any number of arguments to the function.
Example:
# Define a function that accepts multiple
arguments sum_numbers <- function( ) {
return(sum(… ))
}
# Call the function with different numbers of arguments
result1 <- sum_numbers(1, 2, 3)
result2 <- sum_numbers(4, 5, 6, 7)
print(result1) # Outputs: 6
print(result2) # Outputs: 22
Here, the function sum_numbers() can accept any number of arguments due to the use of ….
Summary:
- A function call is done by using the function’s name followed by
- Arguments are passed inside the
- Default arguments can be left out of the function
- You can also pass named arguments to call the function in any
- Functions can take variable numbers of arguments using ….
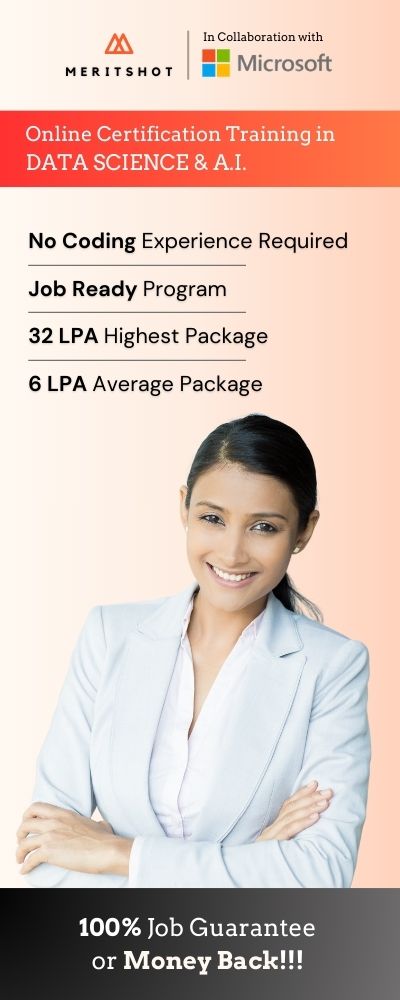