Meritshot Tutorials
- Home
- »
- Protecting Sensitive Data
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Protecting Sensitive Data
Sensitive data includes critical information such as API keys, model files, user credentials, and configuration settings. Mishandling this data can lead to security vulnerabilities, unauthorized access, or data breaches.
What Counts as Sensitive Data?
- API Keys and Credentials:
- API keys for third-party services (e.g., cloud platforms, payment gateways).
- Database usernames and passwords.
- Configuration Settings:
- Database URLs, secret keys, or tokens used in application settings.
- Machine Learning Model Files:
- Pretrained model files that are part of your deployment.
- User Information:
- Passwords, email addresses, personal data, and any personally identifiable information (PII).
Key Strategies for Protecting Sensitive Data
- Use Environment Variables:
- Store sensitive information (e.g., API keys, database credentials) in environment variables instead of hardcoding them into your application code.
- Tools like python-dotenv can load variables from a .env file into your application securely.
- Encrypt Data:
- Encrypt sensitive data both at rest (stored in files, databases, etc.) and in transit (during communication).
- Use HTTPS for all network communication to secure data in transit.
- Encrypt stored passwords using robust algorithms like bcrypt or Argon2.
- Access Control:
- Restrict access to sensitive files and directories. Use file permissions and user roles to ensure that only authorized users can access them.
- Implement role-based access control (RBAC) to manage user access effectively.
- Data Minimization:
- Only collect and retain the minimum amount of data necessary for your application to function.
- Avoid storing sensitive or personal information unless absolutely required.
- Monitor and Audit:
- Regularly monitor and audit the usage of sensitive data to detect any suspicious activity or breaches.
- Use logging frameworks to track access to sensitive endpoints.
Real-World Examples
- Environment Variables:
- Store sensitive keys in a .env file:
API_KEY=your_api_key_here
DATABASE_URL=your_database_url_here
- Load these variables securely in Python using:
import os
api_key = os.getenv(‘API_KEY’)
- HTTPS Encryption:
- Enable HTTPS to encrypt data between the client and the server. For Flask applications, this can be achieved by deploying your app behind a reverse proxy like Nginx with an SSL certificate.
- Encrypting Model Files:
- Protect your machine learning model files by encrypting them before deployment.
- Ensure the decryption process is secure and only occurs on trusted servers.
- Storing Passwords Securely:
- Always hash passwords before storing them in a database using secure hashing algorithms like bcrypt.
Best Practices
- Never Hardcode Secrets:
- Avoid storing API keys, passwords, or other sensitive data directly in your codebase, especially in public repositories.
- Use Secret Management Tools:
- Leverage tools like AWS Secrets Manager, Azure Key Vault, or HashiCorp Vault for secure storage and retrieval of sensitive information.
- Implement Secure Authentication:
- Use multi-factor authentication (MFA) for admin accounts and sensitive systems.
- Regularly Update Dependencies:
- Ensure all libraries and frameworks you use are updated to their latest, secure versions to prevent vulnerabilities.
Frequently Asked Questions
- Why shouldn’t sensitive data be hardcoded into the application?
- Hardcoded data can be exposed if your code is shared, especially in public repositories. This creates a significant security risk.
- What is the purpose of environment variables?
- Environment variables provide a secure way to store and manage sensitive information outside the application code, minimizing the risk of exposure.
- How can I encrypt sensitive data in a Flask application?
- Use libraries like cryptography or pycrypto for encryption and decryption. For network communication, always enforce HTTPS.
- What happens if API keys are accidentally leaked?
- If an API key is leaked, unauthorized users may exploit your resources or services. Immediately revoke and regenerate the key to mitigate the impact.
- Can I use a .env file in production?
- While .env files are useful for development, use secure secret management tools in production to avoid relying on flat files.
- How does HTTPS protect sensitive data?
- HTTPS encrypts data transmitted between the client and server, preventing it from being intercepted or tampered with by attackers.
- What are some common tools for managing secrets?
- AWS Secrets Manager, Azure Key Vault, Google Secret Manager, and HashiCorp Vault are widely used for managing and securing sensitive data.
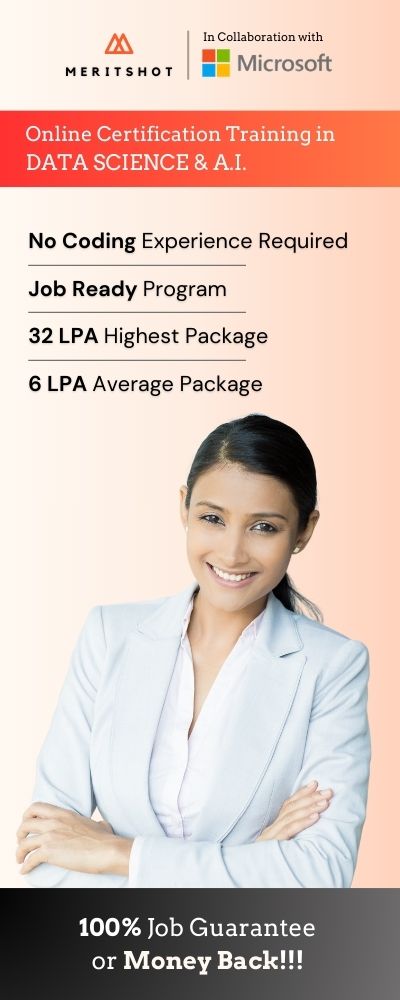