Meritshot Tutorials
- Home
- »
- Handling Requests and Responses
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Serving JSON ResponsesServing JSON Responses
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Handling Requests and Responses
6.1 GET vs. POST Methods
In Flask (and web development in general), the GET and POST methods are two of the most commonly used HTTP request methods. Understanding the difference between them is essential for handling requests and responses in a Flask application, especially when dealing with user inputs and data submission.
1. GET Method
- Characteristics of GET:
- Data is sent in the URL, which is visible to the user (e.g., http://example.com?name=value).
- The GET method has size limitations because URLs are limited in length.
- It is generally considered less secure because the data is exposed in the URL.
- GET is used for retrieving data or requesting a resource without modifying the server state.
Example of GET Request in Flask:
@app.route(‘/hello’, methods=[‘GET’])
def hello():
name = request.args.get(‘name’, ‘Guest’) # Get ‘name’ parameter from URL
return f”Hello, {name}!”
In this example:
- The user can visit a URL like http://localhost:5000/hello?name=John to see the message “Hello, John!”.
- The data (e.g., name=John) is passed in the URL query string.
Common Use Cases for GET:
- Fetching data from an API.
- Rendering a webpage with dynamic content based on URL parameters (like a search query).
- Retrieving information from a server without causing side effects.
- POST Method
The POST method is used to send data to the server. Unlike GET, POST sends the data in the body of the request, which is not visible in the URL. It is typically used for submitting form data or creating or updating resources on the server.
- Characteristics of POST:
- Data is sent in the body of the request, making it more secure than GET.
- POST is not limited by URL length, so it can handle large amounts of data.
- It is used for actions that modify the server’s state, such as creating, updating, or deleting resources.
- POST is more secure than GET for sending sensitive information (like passwords) because the data is not exposed in the URL.
iExample of POST Request in Flask:
@app.route(‘/submit’, methods=[‘POST’])
def submit():
user_data = request.form[‘input_data’] # Get ‘input_data’ from the form
return f”Data submitted: {user_data}”
In this example:
- The user submits a form using POST, and the data entered is sent in the body of the request.
- The input_data field is extracted from request.form.
Common Use Cases for POST:
- Submitting form data (e.g., login forms, feedback forms).
- Sending data to an API that creates or updates resources.
- Handling file uploads.
- Performing operations that involve sensitive or large amounts of data (e.g., user authentication).
Key Differences Between GET and POST:
Feature | GET | POST |
Visibility of Data | Data is visible in the URL (e.g., ?name=value) | Data is hidden in the request body |
Data Length | Limited by URL length (usually a few KB) | No significant limit (depends on server config) |
Security | Less secure (data is visible in URL) | More secure (data is hidden in the request body) |
Caching | Can be cached by browsers or intermediaries | Not cached by default |
Use Case | Retrieving data (e.g., search, get a page) | Submitting data (e.g., form submission, creating data) |
Idempotence | Safe and idempotent (does not modify server state) | Not idempotent (can modify server state) |
How to Use GET and POST in Flask:
In Flask, the request object is used to handle incoming data. Here’s how you use GET and POST methods:
- GET Method: You can access query parameters from the URL using request.args:
python
Copy code
name = request.args.get(‘name’, ‘Guest’) # Default value is ‘Guest’ if ‘name’ is not provided
- POST Method: You can access form data submitted via POST using request.form:
python
Copy code
input_data = request.form[‘input_data’]
When to Use GET vs. POST
- GET: Use the GET method when the action is purely to retrieve data or display information without modifying the server’s state. For example, displaying a webpage, showing search results, or retrieving user details from an API.
- POST: Use the POST method when the action involves submitting data that will create, update, or delete a resource. This includes actions such as submitting a form, making predictions with a machine learning model, or performing a database update.
Frequently Asked Questions
- Can I use GET for submitting form data in Flask?
- Answer: Technically yes, but it is not recommended. GET appends form data in the URL, making it visible and less secure. For sensitive or large data, use POST.
- What is the advantage of using POST over GET?
- Answer: POST is more secure as data is sent in the body of the request, not in the URL. It also allows for larger data payloads and is typically used for actions that change server data (e.g., submitting a form or making a prediction).
- Why should I use POST for machine learning model predictions?
- Answer: Since model predictions often involve sending data (e.g., user input) to the backend, POST is preferred because it handles larger and more secure payloads compared to GET. POST also keeps sensitive data (like passwords or personal details) out of the URL.
- Can I cache GET requests in Flask?
- Answer: Yes, GET requests can be cached by browsers or intermediary caches (e.g., proxies). This makes GET suitable for retrieving data that does not change frequently, such as static resources or search results.
- What happens if I use POST for retrieving data instead of GET?
- Answer: While you can technically use POST for data retrieval, it is not considered best practice. POST is used for actions that modify the server’s state, while GET should be used for fetching data or viewing a resource without causing side effects.
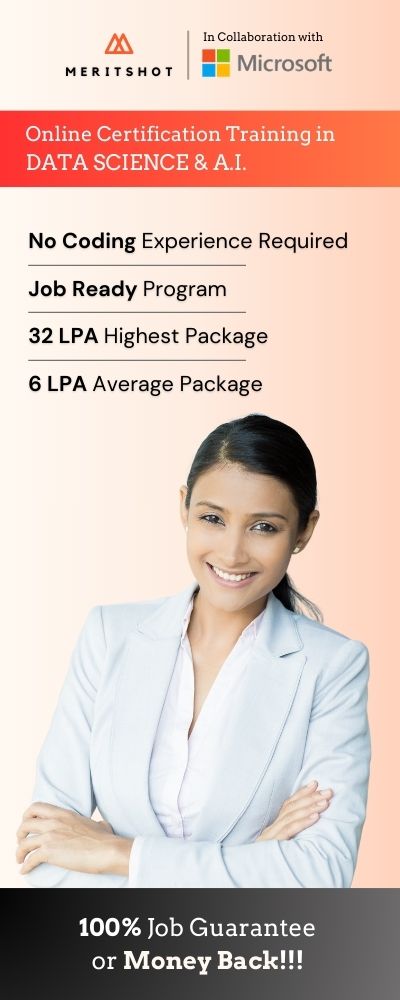