Meritshot Tutorials
- Home
- »
- Deploying on AWS, GCP, or Azure
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Deploying on AWS, GCP, or Azure
Deploying your Flask application on cloud platforms like AWS, Google Cloud Platform (GCP), or Microsoft Azure provides greater flexibility, scalability, and control. These cloud services offer advanced features for hosting web applications, managing resources, and ensuring high availability, which are essential for production-level apps.
Why Choose AWS, GCP, or Azure for Flask Deployment?
- Scalability and Reliability:
- Cloud platforms like AWS, GCP, and Azure provide resources that can scale up or down based on demand. These platforms ensure high availability with robust backup and recovery options.
- Security:
- These platforms offer extensive security measures, such as firewalls, encryption, and identity management, to protect your application and its data.
- Global Reach:
- Deploying on these platforms gives you the advantage of multiple data centers worldwide, improving the performance and availability of your application across different regions.
- Managed Services:
- Cloud providers offer managed services like databases, machine learning tools, monitoring, and analytics, allowing you to offload operational overhead and focus on your application’s core logic.
Steps for Deploying Flask on AWS, GCP, or Azure
The deployment process varies slightly depending on the cloud platform, but the general steps involve setting up the infrastructure, configuring the environment, deploying the application, and managing the resources.
Deploying on AWS (Amazon Web Services)
AWS provides multiple services that can host your Flask application, such as Elastic Beanstalk, EC2, and Lightsail.
Using Elastic Beanstalk
- Set Up AWS Account:
- Create an AWS account at aws.amazon.com.
- Ensure your AWS CLI is configured on your local machine using aws configure.
- Prepare Flask Application:
- Ensure your Flask app has the necessary files, including requirements.txt, Procfile, and runtime.txt (to specify the Python version).
- Initialize Elastic Beanstalk:
- Install the Elastic Beanstalk CLI (eb), and initialize your app:
eb init -p python-3.x flask-app
- Deploy the Application:
- Create an environment and deploy the app using:
eb create flask-env
eb deploy
- Access the Application:
- Once deployed, you can access your Flask application using the provided URL:
eb open
- Scaling and Monitoring:
- Elastic Beanstalk automatically scales your application based on traffic and provides integrated monitoring via Amazon CloudWatch.
Deploying on Google Cloud Platform (GCP)
GCP provides several ways to deploy a Flask app, including Google App Engine (GAE), Compute Engine, or Kubernetes Engine. We’ll focus on deploying using Google App Engine.
Using Google App Engine (GAE)
- Set Up GCP Account:
- Create an account at console.cloud.google.com.
- Install the Google Cloud SDK and authenticate your account.
- Prepare Flask Application:
- Similar to AWS, make sure your app is structured with a requirements.txt, app.yaml, and Procfile.
- Create a Google Cloud Project:
- Create a new project using the GCP console and enable the App Engine service for that project.
- Deploy Flask App:
- Deploy your Flask app by running the following command in your project directory:
gcloud app deploy
- Access Your App:
- Once the deployment is successful, you can access your app via the URL provided by GCP.
- Monitoring and Scaling:
- GCP automatically handles scaling based on incoming traffic. Use the Google Cloud Console to monitor application performance and logs.
Deploying on Microsoft Azure
Azure provides services such as Azure App Service, Virtual Machines, and Kubernetes Service for hosting Flask applications. We’ll demonstrate deploying using Azure App Service.
Using Azure App Service
- Set Up Azure Account:
- Create an Azure account at azure.com.
- Install the Azure CLI tool and authenticate by running:
az login
- Prepare Flask Application:
- Ensure you have requirements.txt, web.config, and a startup.sh (for Linux) or Procfile (for Windows) in your Flask app.
- Create an Azure App Service:
- Create a new App Service in the Azure portal, select the runtime stack (e.g., Python 3.8), and configure it for deployment.
- Deploy Flask Application:
- Deploy your Flask app using Git or via the Azure CLI:
az webapp up –name flaskapp –resource-group myResourceGroup
- Access the Application:
- Once deployed, your app will be accessible via the URL provided by Azure App Service.
- Scaling and Monitoring:
- Azure App Service automatically scales based on demand. Use the Azure portal to view logs and set up scaling policies.
Best Practices for Deploying on Cloud Platforms
- Use CI/CD Pipelines:
- Set up continuous integration/continuous deployment (CI/CD) pipelines to automate testing and deployment of new versions of your application.
- Monitor Performance:
- Use the monitoring tools provided by AWS CloudWatch, GCP Stackdriver, or Azure Monitor to keep track of your app’s performance and troubleshoot any issues.
- Secure Your Application:
- Ensure that your cloud environment is secure by configuring firewalls, using secure storage for sensitive data, and enabling encryption at rest and in transit.
- Optimize for Cost:
- Regularly review your cloud usage and adjust resources accordingly to avoid unnecessary charges. Utilize free tiers or cost-effective services for small projects.
Frequently Asked Questions
- Which cloud platform should I choose for deploying my Flask app?
- The choice depends on your specific needs. AWS, GCP, and Azure are all great options. AWS offers comprehensive services, GCP excels in machine learning tools, and Azure is a great choice for integration with Microsoft products.
- Do I need to configure a database for my Flask app on the cloud?
- If your application requires data storage, you can provision cloud databases (e.g., Amazon RDS, Google Cloud SQL, Azure SQL Database) depending on the platform.
- Can I use a custom domain with my cloud-deployed Flask app?
- Yes, you can configure a custom domain with all major cloud platforms. You’ll need to update DNS settings to point to the provided cloud app URL.
- Is there a free tier available for deploying Flask apps?
- Yes, all three platforms (AWS, GCP, and Azure) provide free tiers with limited resources for testing or personal projects. Be sure to check the limits to avoid incurring charges.
- Can I scale my Flask application on the cloud?
- Yes, all major cloud platforms provide scaling options. You can scale horizontally (adding more instances) or vertically (increasing instance size) depending on your traffic needs.
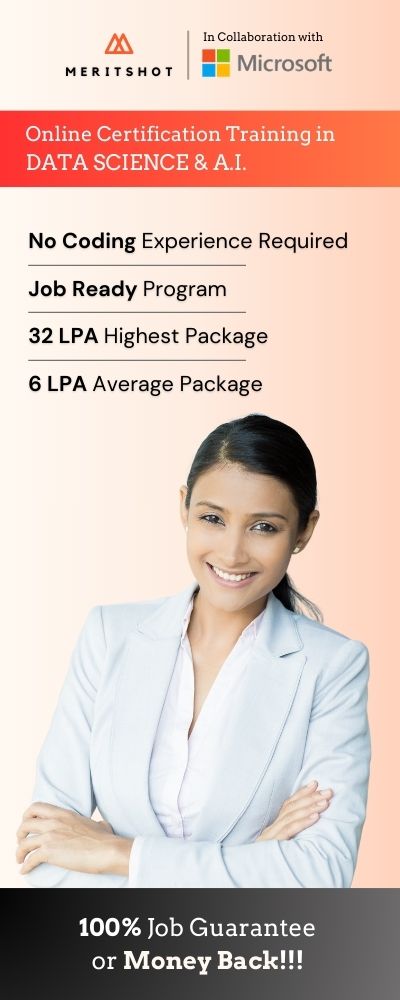