Meritshot Tutorials
- Home
- »
- Containerizing Flask Apps with Docker
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Containerizing Flask Apps with Docker
Containerizing your Flask application with Docker is a powerful way to package it along with all of its dependencies, configurations, and environment settings. This ensures that your application runs consistently across different environments, whether in development, testing, or production. Docker enables you to isolate your app and its dependencies into a container, which can be easily shared and deployed.
Why Containerize Flask Apps with Docker?
Containerization with Docker offers several advantages for Flask applications:
- Consistency Across Environments: Docker ensures that the app behaves the same way across different environments. Whether you are running it locally, in a test environment, or in production, Docker guarantees that the dependencies and configurations are exactly the same, eliminating the “it works on my machine” problem.
- Isolation: Docker containers isolate the Flask app and its dependencies from the host system and other applications. This isolation prevents conflicts between different versions of libraries or dependencies.
- Easy Deployment: Once your Flask app is containerized, you can easily deploy it to any server that supports Docker. This includes cloud platforms like AWS, GCP, or Azure, or even on your local machine for testing.
- Simplified Dependency Management: All the dependencies (e.g., Flask, database drivers) are encapsulated within the container, which simplifies dependency management. You no longer need to worry about missing or incompatible dependencies on the host machine.
Steps for Containerizing a Flask App
To containerize a Flask application with Docker, follow these steps:
1. Create the Flask Application
Let’s assume you have a simple Flask app. Here’s an example:
# app.py
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def hello_world():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
2. Create a requirements.txt File
The requirements.txt file lists all the dependencies that your Flask app needs to run. You can generate this file by running:
pip freeze > requirements.txt
An example of the requirements.txt for the Flask app might look like:
Flask==2.1.1
3. Create a Dockerfile
The Dockerfile defines the instructions for building the Docker image. Here’s an example Dockerfile for a Flask app:
# Use the official Python base image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install the Python dependencies
RUN pip install –no-cache-dir -r requirements.txt
# Expose the port the app will run on
EXPOSE 5000
# Define the command to run the app
CMD [“python”, “app.py”]
4. Build the Docker Image
Now that you have a Dockerfile, you can build your Docker image by running the following command in the directory where your Dockerfile and requirements.txt are located:
docker build -t flask-app .
This command tells Docker to build an image named flask-app based on the instructions in the Dockerfile.
5. Run the Docker Container
Once the image is built, you can run the Flask app in a Docker container using the following command:
docker run -p 5000:5000 flask-app
This command will run the flask-app image, bind port 5000 on your local machine to port 5000 in the container, and start the Flask application.
You can access the app by navigating to http://localhost:5000 in your web browser.
Key Concepts in Containerizing Flask Apps
- Dockerfile:
- A Dockerfile is the blueprint for creating a Docker image. It defines the base image, the application files to copy, the dependencies to install, and the commands to run when the container starts.
- Docker Image:
- A Docker image is a lightweight, standalone, and executable package that contains all the code, runtime, libraries, and dependencies needed to run your app.
- Docker Container:
- A Docker container is a running instance of a Docker image. It is a lightweight, isolated environment where your app runs.
- Port Binding:
- When you run a Docker container, you can bind ports from the container to your local machine to make the application accessible. In the example above, -p 5000:5000 binds port 5000 of the container to port 5000 on your local machine.
Benefits of Dockerizing Your Flask App
- Portability:
- Docker containers can be run on any machine that has Docker installed, making it easy to deploy your Flask application across different environments.
- Simplified Deployment:
- Once the Flask app is containerized, you can deploy it anywhere, whether on cloud platforms like AWS, Azure, GCP, or on-premises.
- Efficient Resource Utilization:
- Docker containers are lightweight and share the host system’s kernel, so they are more efficient compared to virtual machines, which require their own operating system.
- Version Control:
- With Docker, you can easily manage versions of your application and its dependencies. This ensures that your app runs the same way across all environments.
Conclusion
Containerizing Flask applications with Docker enhances portability, consistency, and deployment efficiency. Docker eliminates environment-specific issues and ensures that your Flask app can run seamlessly in any environment, from local development to production.
By following the steps outlined above, you can easily containerize your Flask application and leverage the power of Docker to make your app more scalable and portable.
Frequently Asked Questions
- What is Docker?
- Answer: Docker is a platform that automates the deployment of applications inside lightweight, portable containers. These containers bundle your application and its dependencies together, ensuring that it runs consistently across different environments.
- Why should I containerize my Flask app with Docker?
- Answer: Containerizing your Flask app with Docker provides several advantages, such as portability, consistency across environments, simplified dependency management, and easier deployment. Docker allows you to isolate your app and its dependencies, ensuring that it runs smoothly on any system without conflicts.
- What is a Dockerfile?
- Answer: A Dockerfile is a text file that contains a set of instructions to build a Docker image. It defines the base image to use, the application files to copy, the commands to install dependencies, and the entry point for the container when it starts.
- What is the difference between a Docker image and a Docker container?
- Answer: A Docker image is a static blueprint that contains all the dependencies, libraries, and the application code necessary to run an app. A Docker container is a running instance of that image. In simple terms, an image is like a template, and a container is a running copy of that template.
- Can I use Docker to deploy my Flask app in production?
- Answer: Yes, Docker is commonly used in production environments. Once you containerize your Flask app, you can deploy it on any platform that supports Docker, including cloud services like AWS, GCP, and Azure, or on your local servers.
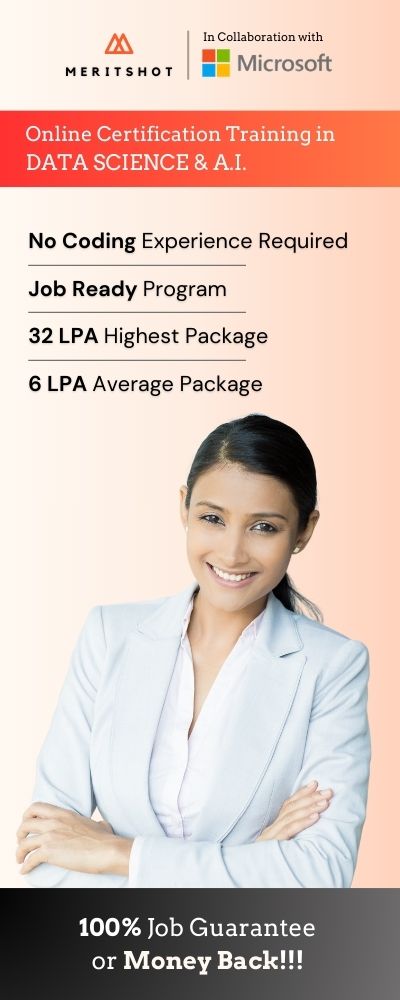