Meritshot Tutorials
- Home
- »
- Batch Predictions vs. Single Predictions
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Batch Predictions vs. Single Predictions
When deploying machine learning models, choosing between batch predictions and single predictions is crucial. Each approach has its advantages and drawbacks, depending on the application’s requirements, data volume, and real-time constraints.
What Are Batch Predictions?
Batch predictions involve processing multiple inputs at once. Instead of handling one prediction request at a time, the model processes a collection of inputs in a single operation.
Advantages of Batch Predictions
- Efficiency: Reduces the overhead of multiple API calls by processing data in bulk.
- Speed: For large datasets, it is often faster to process in batches than making repeated single prediction calls.
- Lower Cost: Reduces server load and minimizes the number of requests to the backend.
- Ideal for Scheduled Jobs: Useful for non-real-time applications like generating predictions for a daily report.
Disadvantages of Batch Predictions
- Higher Memory Requirements: Processing large batches requires more memory, which might be a constraint for resource-limited servers.
- Latency for Small Data: If the input size is small, waiting to accumulate a full batch can introduce unnecessary delays.
- Error Handling: If one input in the batch is problematic, it might affect the entire batch unless error-handling mechanisms are implemented.
What Are Single Predictions?
Single predictions process one input at a time. Each request receives an immediate response for that particular input.
Advantages of Single Predictions
- Real-Time Responses: Ideal for interactive applications where immediate feedback is required.
- Lower Memory Usage: Handles one input at a time, requiring less memory and computational power.
- Simpler Error Handling: Easier to debug issues with individual inputs as they are processed independently.
- Flexible: Suitable for scenarios where users submit inputs one by one.
Disadvantages of Single Predictions
- Less Efficient for Large Data: Processing many small requests increases overhead due to repeated API calls.
- Higher Cost: Can result in more server load and computational expense for high-frequency requests.
When to Use Batch Predictions
- Scenario 1: Predicting sales trends for a dataset of thousands of transactions in one go.
- Scenario 2: Running nightly predictions on data collected throughout the day.
- Scenario 3: Non-urgent tasks such as analyzing customer data for marketing insights.
When to Use Single Predictions
- Scenario 1: Real-time fraud detection for a single transaction.
- Scenario 2: Chatbots or interactive tools where users input data one at a time.
Scenario 3: Mobile or web apps where predictions are made based on user interactions.
Implementing Batch Predictions and Single Predictions in Flask
- Batch Predictions:
- Accept an array of inputs via a POST request.
- Loop through or use vectorized operations to process all inputs.
- Return an array of predictions.
- Single Predictions:
- Accept one input via a POST or GET request.
Process the input and return the result as a single prediction.
Key Considerations
- Performance Optimization: For batch predictions, ensure the server has adequate memory and CPU/GPU resources. For single predictions, optimize latency to maintain responsiveness.
- Error Handling: In batch predictions, identify and log problematic inputs while continuing with the rest of the batch. In single predictions, return specific error messages for invalid inputs.
- Hybrid Approach: In some cases, you may use a hybrid approach where small batches are created dynamically based on incoming requests, balancing real-time and efficiency needs.
Frequently Asked Questions
- Which approach is better for handling real-time user inputs?
- Single predictions are better for real-time applications where immediate feedback is required.
- Can I process very large datasets using batch predictions?
- Yes, but ensure to divide the dataset into smaller batches if memory constraints exist.
- What are the limitations of batch predictions?
- Batch predictions require more memory, and errors in a single input might impact the entire batch without proper handling.
- How can I optimize single predictions for high-frequency requests?
- Use caching and optimize model inference speed to reduce latency.
- Is it possible to switch between batch and single predictions dynamically?
- Yes, design your API to handle both scenarios based on the request payload (e.g., process as a batch if an array is provided or as a single prediction if only one input is given).
- How do I handle errors in batch predictions?
- Implement error-handling mechanisms to skip or log invalid inputs and continue processing the rest of the batch.
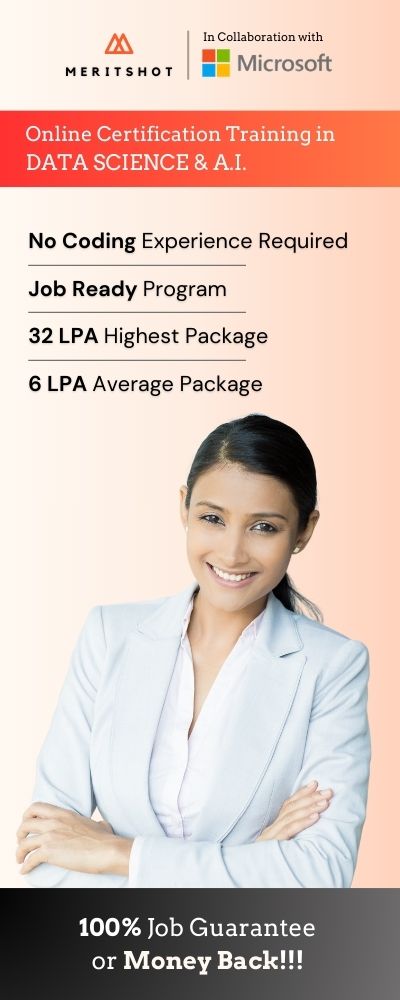