Meritshot Tutorials
- Home
- »
- Basics of Flask
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Serving JSON ResponsesServing JSON Responses
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Basics of Flask
2.1 "Hello, World!" in Flask
Creating a “Hello, World!” application is the first step to understanding how Flask works. This simple example demonstrates how to set up a Flask application, define routes, and run the application on a local server.
Step 1: Create the Project Directory
- Open your terminal or command prompt.
- Create a new directory for your project:
mkdir flask_basics
cd flask_basics
- (Optional but recommended) Create a virtual environment:
python -m venv venv
- Activate the virtual environment:
venv\Scripts\activate
- On macOS/Linux:
source venv/bin/activate
- Install Flask in the virtual environment:
pip install flask
Step 2: Create the Flask Application
- Create a file named app.py in the project directory. This file will contain your Flask application.
- Write the following code in app.py:
from flask import Flask
# Create a Flask app instance
app = Flask(__name__)
# Define a route for the root URL (‘/’)
@app.route(‘/’)
def hello_world():
return “Hello, World!”
# Run the app
if __name__ == ‘__main__’:
app.run(debug=True)
Explanation of the Code
- Import Flask:
from flask import Flask imports the Flask library to create the application. - Create the App Instance:
app = Flask(__name__) creates a Flask application instance. The __name__ argument helps Flask locate resources and determine the application’s root path. - Define a Route:
- @app.route(‘/’) is a decorator that maps the URL ‘/’ (the root URL) to the hello_world function.
- The function hello_world() returns the response “Hello, World!”, which will be displayed in the browser.
- Run the App:
- app.run(debug=True) starts the Flask development server.
The debug=True option enables automatic reloading and provides detailed error messages for debugging.
Step 3: Run the Flask Application
- Open the terminal and navigate to the directory containing app.py:
cd flask_basics
- Run the application:
python app.py
- You’ll see an output similar to this:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
* Restarting with stat
* Debugger is active!
- Open a web browser and go to http://127.0.0.1:5000/.
You’ll see the message “Hello, World!” displayed.
Key Notes
- Dynamic Reloading: With debug=True, any changes made to your code will automatically reload the server.
- Stopping the Server: Press CTRL+C in the terminal to stop the Flask development server.
Frequently Asked Questions
- Q: What is Flask?
A: Flask is a lightweight Python web framework that allows developers to build web applications and APIs quickly and easily. It is known for its simplicity and flexibility. - Q: Why is the “Hello, World!” example important?
A: This example helps beginners understand the basics of Flask, such as creating an app instance, defining routes, and running a local server. - Q: What does @app.route(‘/’) do?
A: This decorator maps the root URL (/) of the application to the hello_world function. When a user visits the root URL, the function is executed, and its return value is displayed in the browser. - Q: Why do I need to use if __name__ == ‘__main__’:?
A: This ensures that the Flask app runs only when the script is executed directly, not when it is imported as a module in another script. - Q: What is the purpose of debug=True?
A: The debug=True flag enables the debug mode, which automatically reloads the server when code changes are detected and provides detailed error messages for troubleshooting. - Q: How can I access the Flask app from another device on the same network?
A: Instead of 127.0.0.1, run the app with app.run(host=’0.0.0.0′) to allow access from other devices on the same network. Use your machine’s local IP address to access the app.
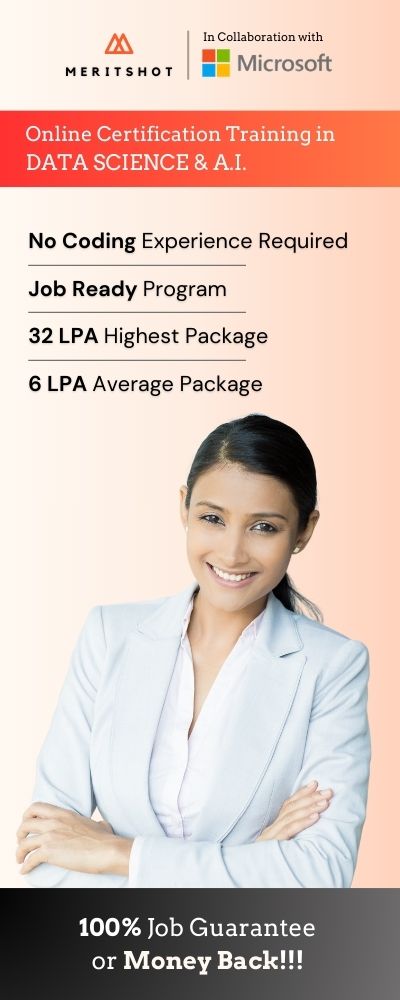