Meritshot Tutorials
- Home
- »
- Adding Authentication and Security
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Adding Authentication and Security
10.1 Securing Flask Endpoints
When deploying machine learning models using Flask, security is a critical aspect to protect the application from unauthorized access, data breaches, and malicious attacks. Flask provides several ways to secure endpoints effectively.
Why Secure Flask Endpoints?
- Prevent Unauthorized Access: Ensure that only authenticated and authorized users can access certain resources or endpoints.
- Protect Sensitive Data: Safeguard user inputs, model predictions, and other sensitive information.
- Mitigate Security Threats: Reduce vulnerabilities like SQL injection, Cross-Site Scripting (XSS), and Cross-Site Request Forgery (CSRF).
- Ensure Compliance: Adhere to data protection regulations, such as GDPR or HIPAA, when handling user data.
Common Security Practices for Flask Endpoints
- Authentication and Authorization
- Use authentication to verify the user’s identity.
- Apply authorization to determine what resources the user can access.
- Implement tools like Flask-Login or Flask-JWT-Extended for managing user authentication.
- HTTPS for Secure Communication
- Always deploy the Flask app using HTTPS instead of HTTP to encrypt data transfer between the server and client.
- Use tools like Let’s Encrypt for free SSL certificates.
- Input Validation
- Validate and sanitize user inputs to prevent malicious data from being processed.
- Use libraries like WTForms or Flask-WTF for robust input validation.
- Cross-Origin Resource Sharing (CORS)
- Restrict access to your API by specifying allowed origins with Flask-CORS.
- Avoid exposing your endpoints to all origins unless absolutely necessary.
- Rate Limiting
- Prevent abuse or denial-of-service (DoS) attacks by limiting the number of requests a user can make.
- Use libraries like Flask-Limiter to implement rate limiting.
- Error and Exception Handling
- Avoid exposing sensitive information in error messages.
- Customize error handling to log errors without revealing stack traces or debug messages to users.
- Use API Keys or Tokens
- Require API keys or tokens for accessing certain endpoints.
- Implement tools like Flask-JWT-Extended or OAuth for secure token-based access.
- Disable Debug Mode in Production
- Running Flask in debug mode exposes sensitive application details. Always disable it in production environments.
Example: Adding Authentication to Flask Endpoints
Below is a high-level explanation of how to secure an endpoint using token-based authentication.
Steps to Add Token-Based Authentication
- Install Flask-JWT-Extended:
bash
Copy code
pip install flask-jwt-extended
- Set Up Token Authentication:
- Generate tokens during login and validate tokens for protected endpoints.
- Restrict Access to Certain Endpoints:
- Use decorators like @jwt_required() to restrict access to endpoints.
Best Practices for Securing Flask Endpoints
- Keep Secrets Secure:
- Store secret keys, database credentials, and API tokens in environment variables or secret managers, not in the codebase.
- Regular Security Audits:
- Regularly test your application for vulnerabilities using tools like OWASP ZAP or Burp Suite.
- Implement Logging:
- Monitor access logs to detect suspicious activities.
- Update Dependencies:
- Regularly update Flask and its libraries to patch known vulnerabilities.
- Use Content Security Policies (CSP):
- Define which scripts or resources can be loaded on your application to prevent XSS attacks.
Frequently Asked Questions
- What is the difference between authentication and authorization?
- Authentication verifies a user’s identity, while authorization determines what actions the user is permitted to perform.
- How do I secure sensitive data in API responses?
- Use HTTPS, limit response data to only necessary information, and avoid exposing internal server details.
- What should I do if I suspect unauthorized access to my API?
- Investigate the logs, block suspicious IP addresses, and update authentication mechanisms like tokens or passwords.
- Can I implement security without third-party libraries?
- Yes, but using libraries like Flask-JWT-Extended or Flask-CORS simplifies implementation and reduces the risk of errors.
- Is HTTPS necessary for local development?
- Not strictly necessary for local development, but always mandatory in production to secure communication.
- What happens if an API key is compromised?
- Immediately revoke the key, issue a new one, and investigate how it was compromised to prevent future incidents.
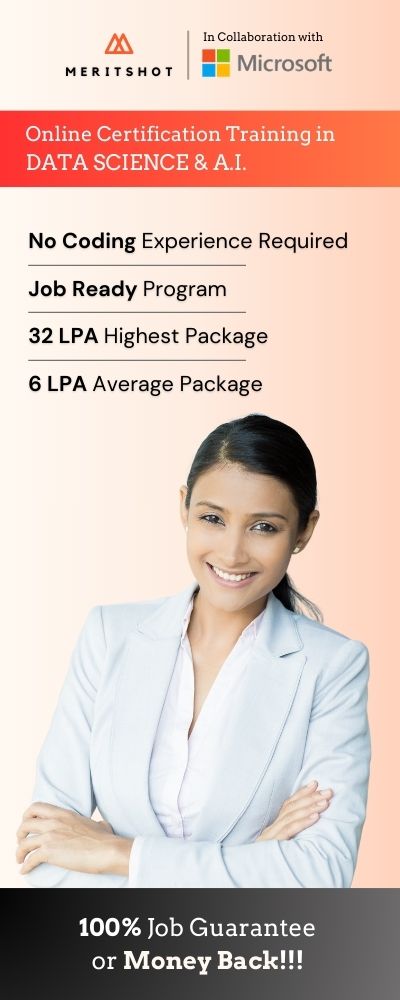