Meritshot Tutorials
- Home
- »
- Adding API Authentication (Token-Based)
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Adding API Authentication (Token-Based)
Authentication is a fundamental security measure that ensures only authorized users or systems can interact with your API. Token-based authentication is a robust and scalable method to secure APIs, particularly in modern applications where client-server communication is a critical component.
What is Token-Based Authentication?
Token-based authentication relies on tokens to verify a user’s identity and grant them access to secure resources. A token is a unique, encoded piece of data, often in the form of a JSON Web Token (JWT), that serves as proof of authentication.
- Why Tokens? Unlike session-based authentication, tokens are stateless, meaning the server does not need to maintain session information for each user. This makes token-based systems more scalable.
- How Tokens Work: Tokens are generated after successful authentication and must be included in every request to access protected endpoints.
How It Works
Token-based authentication involves a multi-step process that ensures secure access to API endpoints:
- User Authentication:
- The user provides their credentials (e.g., username and password) via a login form or API endpoint.
- The server verifies these credentials against a database.
- Token Issuance:
- If the credentials are valid, the server generates a token.
- For example, a JSON Web Token (JWT) might be issued. It typically contains encoded data such as the user ID, roles, and an expiration timestamp.
- The token is sent back to the client (e.g., a browser or mobile app).
- Token Usage:
- The client stores the token securely (e.g., in localStorage, sessionStorage, or an HTTP-only cookie).
- The token is included in the Authorization header of every subsequent request to protected endpoints.
- Validation:
- When the server receives a request, it checks the token for validity:
- Signature Validation: The server ensures the token hasn’t been tampered with by verifying its digital signature.
- Expiration Check: The server checks the expiration time embedded in the token.
- If the token is valid, access is granted to the requested resource.
- When the server receives a request, it checks the token for validity:
Advantages of Token-Based Authentication
- Stateless: Tokens do not require the server to maintain session state, making the system more scalable.
- Cross-Domain Usage: Tokens can be used across different domains and services, making them ideal for distributed systems.
- Decoupled Authentication: The authentication server can be separate from the resource server, enabling microservice architectures.
Best Practices for Token-Based Authentication
- Set Token Expiration Times:
- Tokens should have a short lifespan to minimize the impact of token theft.
- Example: Access tokens might expire after 15 minutes, while refresh tokens can last longer (e.g., 7 days).
- Use Secure Tokens:
- JSON Web Tokens (JWTs) are widely used for their simplicity and security.
- Ensure JWTs are signed with strong algorithms like HMAC SHA-256 or RSA.
- Secure Token Storage:
- Store tokens in secure locations:
- For web apps, use HTTP-only cookies to prevent cross-site scripting (XSS) attacks.
- Avoid storing tokens in localStorage for sensitive applications.
- Store tokens in secure locations:
- Implement Token Revocation:
- Maintain a token blacklist on the server to revoke tokens that are compromised or no longer valid.
- Use HTTPS:
- Always encrypt communication between the client and server using HTTPS to prevent token interception during transmission.
- Add Token Validation Layers:
- Include mechanisms to detect token reuse or misuse, such as IP address or user-agent validation.
Frequently Asked Questions
- What is the difference between session-based and token-based authentication?
- Session-based authentication requires the server to store session information for each user, while token-based authentication is stateless and does not require server-side storage of user sessions.
- What is a JSON Web Token (JWT)?
- A JWT is a compact, self-contained token format that encodes claims about the user. It typically contains a header, payload, and signature to ensure security and integrity.
- How can I protect tokens from being stolen?
- Use secure storage mechanisms like HTTP-only cookies to prevent client-side attacks (e.g., XSS). Always use HTTPS to encrypt data in transit.
- What happens if a token is stolen?
- If a token is stolen, the attacker can use it to access protected resources until it expires. To mitigate this risk, use short-lived tokens and implement token revocation mechanisms.
- Can token-based authentication work for mobile apps?
- Yes, token-based authentication is ideal for mobile apps as tokens can be stored securely in device-specific storage, such as Keychain (iOS) or Keystore (Android).
- What is the purpose of refresh tokens?
- Refresh tokens are long-lived tokens used to request new access tokens without requiring the user to log in again. This improves the user experience while maintaining security.
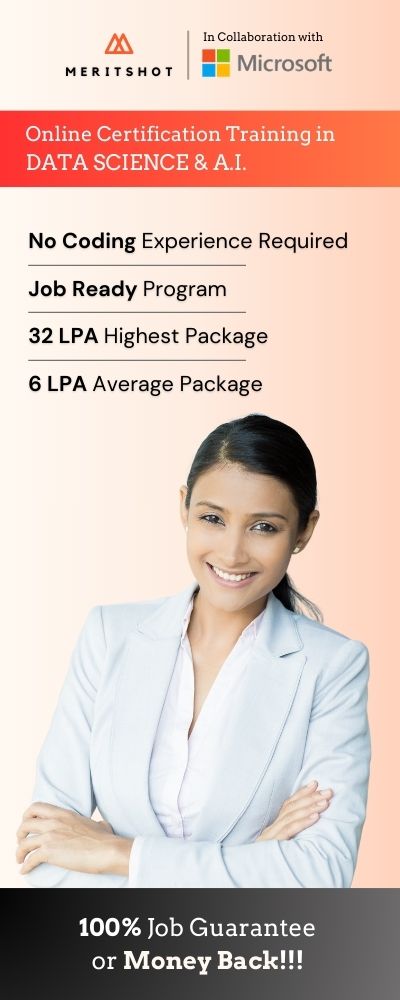