Meritshot Tutorials
- Home
- »
- Accepting User Input for Predictions
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Serving JSON ResponsesServing JSON Responses
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Accepting User Input for Predictions
One of the most important tasks in deploying a machine learning model through Flask is accepting user input and using it for making predictions. In a web application, this typically involves accepting data from users via HTML forms, and then passing that data to the machine learning model for prediction. Here’s how you can handle this process in Flask, from accepting input to generating predictions.
- Creating a Form for User Input
In a Flask application, you usually collect user input via HTML forms. These forms can collect various types of data, such as text, numbers, or even files, depending on the type of machine learning model and the prediction it requires.
- Basic HTML Form: Here’s an example of how to create a simple form where users can enter values that will be passed to your model for prediction.
<form action=”/predict” method=”POST”>
<label for=”feature1″>Feature 1:</label>
<input type=”text” id=”feature1″ name=”feature1″ required><br><br>
<label for=”feature2″>Feature 2:</label>
<input type=”text” id=”feature2″ name=”feature2″ required><br><br>
<input type=”submit” value=”Predict”>
</form>
In this form:
- action=”/predict” indicates that the form will submit data to the /predict endpoint of your Flask application.
- The method POST means the form data will be sent in the body of the request, which is ideal for handling user input securely.
2. Accepting User Input in Flask
Once the user submits the form, Flask will capture the data submitted via POST in the request.form object. You can access each of the input fields by referring to their name attribute.
- Handling POST Request in Flask: Here’s an example of how to handle the form data in Flask and pass it to the machine learning model for prediction:
from flask import Flask, request, render_template
import pickle
import numpy as np
app = Flask(__name__)
# Load the trained model (ensure the model file exists)
with open(‘model.pkl’, ‘rb’) as model_file:
model = pickle.load(model_file)
@app.route(‘/’)
def home():
return render_template(‘index.html’) # Show the form to the user
@app.route(‘/predict’, methods=[‘POST’])
def predict():
# Extract user input from the form
feature1 = float(request.form[‘feature1’]) # Convert input to float (or the expected type)
feature2 = float(request.form[‘feature2’])
# Prepare the data in the format expected by the model
user_input = np.array([[feature1, feature2]])
# Make prediction using the loaded model
prediction = model.predict(user_input)
# Return the prediction to the user
return f’The predicted value is: {prediction[0]}’
if __name__ == “__main__”:
app.run(debug=True)
In this example:
- The request.form[‘feature1’] and request.form[‘feature2’] capture the values entered by the user in the HTML form.
- The float() function is used to convert the input values to the expected type (e.g., float for numerical input).
- The model.predict() method makes a prediction based on the user’s input, and the result is displayed as part of the response.
- Handling Data Preprocessing
Before feeding the input data to the machine learning model, you may need to perform some data preprocessing (e.g., scaling, encoding categorical variables, etc.). In a real-world application, this preprocessing step should be the same as what was done during model training.
Example of Preprocessing Before Prediction:
from sklearn.preprocessing import StandardScaler
# Assuming the model expects scaled data
scaler = StandardScaler()
@app.route(‘/predict’, methods=[‘POST’])
def predict():
# Extract and preprocess input
feature1 = float(request.form[‘feature1’])
feature2 = float(request.form[‘feature2’])
# Preprocess the input data (e.g., scaling)
user_input = np.array([[feature1, feature2]])
user_input_scaled = scaler.fit_transform(user_input)
# Make prediction using the scaled data
prediction = model.predict(user_input_scaled)
return f’The predicted value is: {prediction[0]}’
In this case:
- scaler.fit_transform() standardizes the input data so that it matches the format expected by the model (assuming you applied scaling during model training).
- Returning Predictions to the User
Once the prediction is made, you can send it back to the user. This can be done by rendering a new HTML page or simply returning the result as a string on the same page.
- Returning Prediction in a Template: You can use Flask’s Jinja2 templating engine to display the prediction dynamically in the HTML page.
@app.route(‘/predict’, methods=[‘POST’])
def predict():
feature1 = float(request.form[‘feature1’])
feature2 = float(request.form[‘feature2’])
user_input = np.array([[feature1, feature2]])
# Make prediction
prediction = model.predict(user_input)
# Pass the prediction to the HTML template for rendering
return render_template(‘result.html’, prediction=prediction[0])
In the result.html file:
<h1>Prediction Result: {{ prediction }}</h1>
Frequently Asked Questions
- Can I accept user input other than text (e.g., file uploads)?
- Answer: Yes, Flask can handle file uploads (e.g., images, CSV files) using forms. Use request.files to handle file inputs. However, be sure to process the files properly before passing them to your model.
- How do I handle missing or incorrect user input?
- Answer: You should validate user input using request.form.get() with default values, and handle exceptions for invalid input types (e.g., non-numeric inputs) using try/except blocks.
- What if the user enters too much data or the wrong format?
- Answer: You can validate the user input before processing it. For example, check if the data is within expected ranges, if it’s the correct type (e.g., float or int), and if it matches the required format (e.g., date, email).
- Can I use a dropdown or radio button for input instead of text fields?
- Answer: Yes, you can use HTML elements like <select>, <option>, or <input type=”radio”> to allow the user to choose from predefined options. These can be accessed in Flask similarly to text inputs using request.form.
- How do I handle large datasets in a form input?
- Answer: For large datasets, it is better to upload a file (e.g., CSV) instead of using a form with many fields. You can use request.files to handle file uploads and parse them (e.g., using pandas for CSV files) before passing the data to the model.
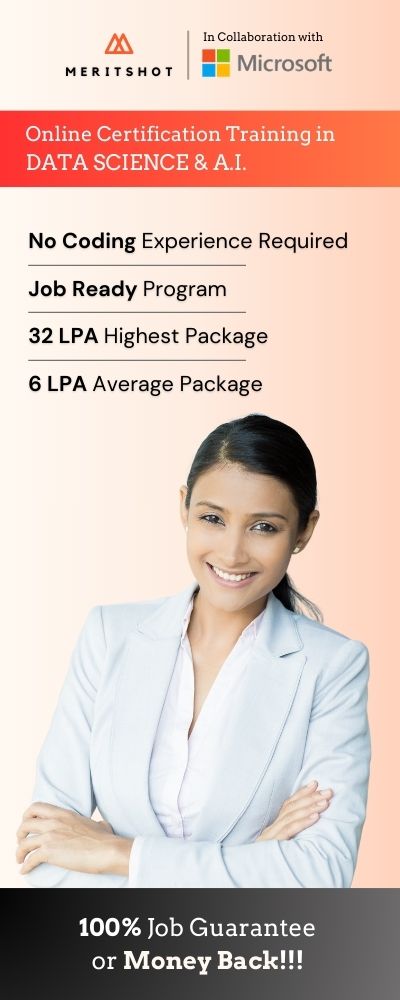