Meritshot Tutorials
- Home
- »
- Connecting the Frontend to the Backend
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Serving JSON ResponsesServing JSON Responses
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Connecting the Frontend to the Backend
Once you have created the HTML form in the frontend and set up your machine learning model on the backend, the next step is to connect the two. This means sending the data entered by the user to the Flask backend, processing the data, and returning the prediction to be displayed in the frontend.
This process involves the following steps:
- User Input (Frontend): The user submits data through an HTML form.
- Form Data Submission: The form sends the data to the backend using an HTTP request (usually POST).
- Backend Processing: The Flask backend receives the data, processes it, makes a prediction using the machine learning model, and returns the result.
- Display the Prediction (Frontend): The result from the backend (the prediction) is sent back to the frontend and displayed to the user.
Let’s go through each of these steps in detail:
Step 1: Frontend - HTML Form (User Input)
First, ensure that you have an HTML form where the user can input the required data for prediction. Here’s an example of a form that collects a feature (e.g., house size or sepal length) and submits it to the Flask backend:
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>ML Model Prediction</title>
</head>
<body>
<h1>Enter Data for Prediction</h1>
<form method=”POST” action=”/predict”>
<label for=”input_data”>Enter a feature (e.g., house size or sepal length):</label>
<input type=”text” id=”input_data” name=”input_data” required><br><br>
<button type=”submit”>Predict</button>
</form>
{% if prediction %}
<h3>Prediction: {{ prediction }}</h3>
{% endif %}
</body>
</html>
In the above form:
- The user enters data in the input field.
- The form uses the POST method to send the data to the /predict route.
Step 2: Backend - Flask Route to Handle Form Submission
When the user submits the form, the backend needs to handle the form submission. In Flask, you define a route to handle the form data and send it to the machine learning model for prediction.
Here’s an example of how you can handle the form submission in Flask:
from flask import Flask, render_template, request
import pickle # For loading the saved ML model
app = Flask(__name__)
# Load the trained model
model = pickle.load(open(‘model.pkl’, ‘rb’))
@app.route(“/”, methods=[“GET”, “POST”])
def index():
prediction = None
if request.method == “POST”:
# Retrieve input data from the form
input_data = float(request.form[“input_data”])
# Process the input and get the prediction from the model
prediction = model.predict([[input_data]])[0] # Make sure the input matches the model’s expectations
return render_template(“index.html”, prediction=prediction)
if __name__ == “__main__”:
app.run(debug=True)
In this code:
- The POST method receives the data from the form via request.form.
- input_data is then passed to the model to generate a prediction.
- The result (prediction) is sent back to the frontend via render_template, where it can be displayed.
Step 3: Frontend - Display the Prediction
Once the backend processes the data and makes a prediction, the result is sent back to the frontend. You can display the result on the same page as follows:
In the index.html template, use the Jinja2 syntax to display the prediction dynamically:
{% if prediction %}
<h3>Prediction: {{ prediction }}</h3>
{% endif %}
This will show the prediction result after the form is submitted and processed by the Flask backend.
Step 4: Testing the Connection
After setting up both the frontend (HTML form) and the backend (Flask route), test the connection by running the Flask app. Here’s how it works:
- The user enters data into the HTML form.
- When the form is submitted, the data is sent to the Flask backend.
- Flask processes the data, uses the trained machine learning model to make a prediction, and sends the result back to the frontend.
- The prediction is displayed to the user.
Step 4: Testing the Connection
After setting up both the frontend (HTML form) and the backend (Flask route), test the connection by running the Flask app. Here’s how it works:
- The user enters data into the HTML form.
- When the form is submitted, the data is sent to the Flask backend.
- Flask processes the data, uses the trained machine learning model to make a prediction, and sends the result back to the frontend.
- The prediction is displayed to the user.
Key Concepts in Connecting Frontend to Backend:
- POST Request: The HTML form uses a POST request to send data to the backend. This is important because POST ensures that data is submitted securely in the request body.
- Flask Routes: Flask routes handle both GET (loading the page) and POST (form submission) requests.
- Machine Learning Model: The trained machine learning model is used to make predictions based on user input.
- Jinja2 Templating: Flask uses Jinja2 for rendering dynamic content in the frontend, allowing the display of predictions.
Frequently Asked Questions
- How do I send data from an HTML form to Flask?
- Answer: You can send data from an HTML form to Flask using the POST method. The form’s action attribute should point to the Flask route where the form data will be processed.
- How do I access form data in Flask?
- Answer: In Flask, you can access form data using request.form[“field_name”], where field_name is the name attribute of the input field in your HTML form.
- How do I display the result of a prediction in the frontend?
- Answer: After processing the prediction in Flask, you can pass the result to the template using render_template(). In the template, you can display the result using Jinja2 syntax, like {{ prediction }}.
- How do I pass data from the frontend to the model in Flask?
- Answer: You pass data from the frontend to the model by retrieving the data from the form in Flask, processing it (e.g., converting it to the correct format), and passing it to the model for prediction.
- Can I handle multiple inputs from the form?
- Answer: Yes, you can handle multiple inputs by adding more fields to the form and retrieving them using request.form[“field_name”] for each input.
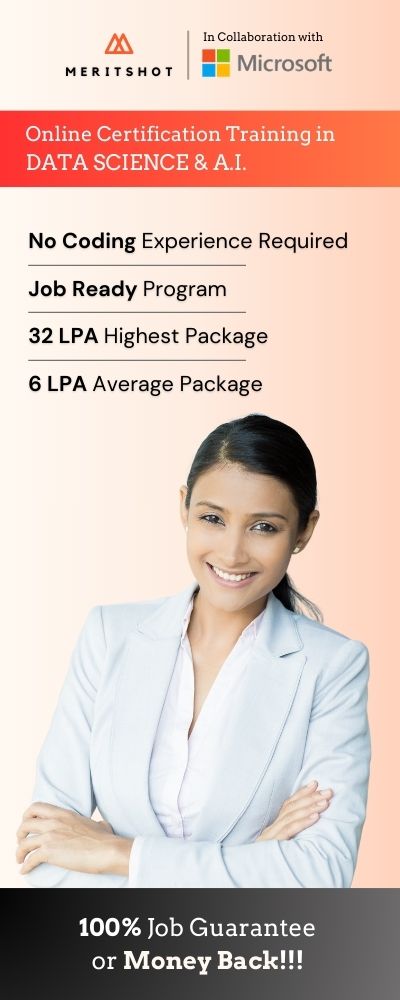