Meritshot Tutorials
- Home
- »
- Flask Templates and Jinja2 Basics
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
Flask Templates and Jinja2 Basics
Flask uses Jinja2 as its templating engine to render HTML pages dynamically. With Jinja2, you can embed Python-like expressions directly into HTML files, allowing the server to generate dynamic content that is based on data passed from the backend.
Key Concepts:
- Jinja2 Templates: These are HTML files that include placeholders for dynamic content. Flask processes these files and replaces the placeholders with actual data from your Python code.
- Flask render_template Function: This function is used to render a template and pass data to it. It’s essential for integrating dynamic Python code into the HTML content.
Creating a Template
- Creating the Template File:
In your Flask project, create a folder named templates. Inside this folder, create an HTML file (e.g., index.html), which will be used to render the webpage.
Example index.html template:
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>ML Model Prediction</title>
</head>
<body>
<h1>Enter Data for Prediction</h1>
<form method=”POST” action=”/predict”>
<label for=”input_data”>Enter your data:</label>
<input type=”text” id=”input_data” name=”input_data” required><br><br>
<button type=”submit”>Predict</button>
</form>
{% if prediction %}
<h3>Prediction: {{ prediction }}</h3>
{% endif %}
</body>
</html>
In this template:
- {{ prediction }}: This is a placeholder that will be replaced with the value of the prediction variable passed from the backend.
- {% if prediction %}: This conditional checks whether a prediction exists before displaying it on the page.
Rendering the Template in Flask
In the Flask backend, use the render_template function to send data to the HTML template.
Example backend code:
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route(“/”, methods=[“GET”, “POST”])
def index():
prediction = None
if request.method == “POST”:
# Example: Get user input
input_data = request.form[“input_data”]
# Process input_data and generate prediction (dummy example)
prediction = “Predicted value: 42” # Replace with model prediction logic
return render_template(“index.html”, prediction=prediction)
if __name__ == “__main__”:
app.run(debug=True)
In this backend code:
- The index route handles both GET and POST requests.
- If the request is POST (i.e., the user submits the form), it processes the input data and generates a prediction.
- The render_template function renders the index.html template and passes the prediction value to it.
Key Benefits of Using Flask Templates and Jinja2:
- Separation of Logic and Presentation: Keeps your HTML structure separate from your Python code, making it easier to manage and maintain the application.
- Dynamic Content Rendering: Enables dynamic rendering of content based on data from the backend (like predictions from a machine learning model).
- Conditional Rendering: Allows conditional display of content (e.g., only showing predictions if a prediction exists).
By leveraging Jinja2 templates, you can build dynamic, data-driven web pages that interact with your machine learning models seamlessly.
Frequently Asked Questions
1. What is Jinja2 in Flask?
Answer: Jinja2 is a templating engine used by Flask to render HTML pages dynamically. It allows you to embed Python-like expressions within HTML files and generate dynamic content based on data passed from the backend.
2. How do I pass data from Flask to an HTML template?
Answer: You pass data to a template using the render_template function in Flask. For example:
return render_template(“index.html”, variable_name=value)
In the template, you can access the value using {{ variable_name }}.
3. Can I use Python code in Jinja2 templates?
Answer: Yes, Jinja2 allows you to use Python-like expressions and control structures (such as if, for, etc.) in the template files. However, you cannot directly run Python code; instead, you use placeholders and filters to process data.
4. How do I handle forms in Flask templates?
Answer: Forms can be created using HTML <form> tags. When the form is submitted, the data can be accessed in Flask using request.form[“field_name”]. You can then process the data and send a response back to the template.
5. What is the purpose of render_template in Flask?
Answer: The render_template function is used to render an HTML template and pass data to it. It replaces the placeholders in the template with the data provided, creating a dynamic webpage.
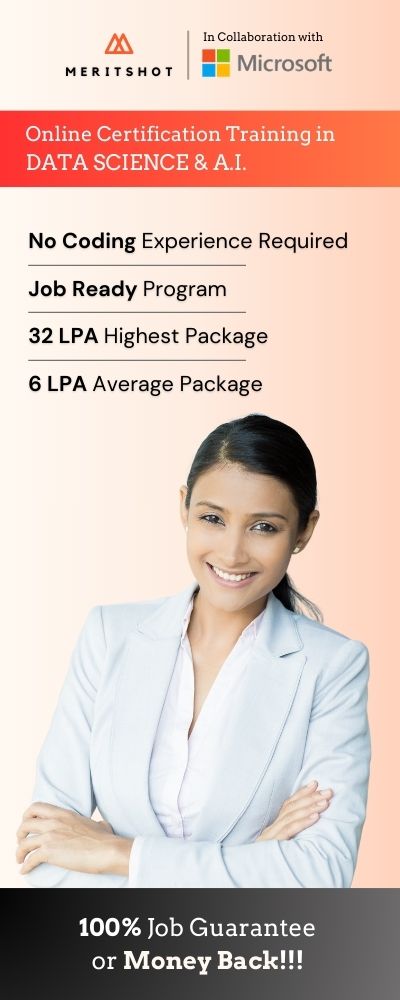