Meritshot Tutorials
- Home
- »
- Setting Up API Endpoints for Prediction
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
Setting Up API Endpoints for Prediction
In a Flask application, API endpoints are used to expose specific functionality of your app. For machine learning models, the primary endpoint would be one that accepts input data, processes it, and returns predictions. Setting up an API endpoint for serving predictions from a trained model is a common practice when deploying machine learning models.
In this section, we’ll walk through how to set up an endpoint like /predict to serve predictions from your model.
Understanding the /predict Endpoint
The /predict endpoint will typically:
- Receive input data from the user in a request (usually in JSON format).
- Preprocess the input data if necessary (e.g., scaling, reshaping).
- Make a prediction using the loaded machine learning model.
- Return the result (prediction) as a response in a user-friendly format, typically JSON.
Steps to Set Up the /predict Endpoint
Let’s create a simple Flask API with a /predict endpoint that serves predictions based on a trained machine learning model.
Step 1: Importing Required Libraries
from flask import Flask, request, jsonify
import pickle
import numpy as np
- Flask: Used to create the Flask app.
- request: Used to extract incoming request data.
- jsonify: Used to return data in JSON format.
- pickle: Used to load the saved machine learning model.
- numpy: Used for handling numerical data, especially when input data needs reshaping for the model.
Step 2: Loading the Trained Model
# Load the trained model (replace ‘model.pkl’ with your model file)
with open(“model.pkl”, “rb”) as model_file:
model = pickle.load(model_file)
In this step, we load the trained model using pickle. This model will be used for making predictions in the /predict endpoint.
Step 3: Defining the /predict Endpoint
app = Flask(__name__)
# Define the /predict endpoint for prediction
@app.route(“/predict”, methods=[“POST”])
def predict():
try:
# Extract the JSON data from the incoming request
data = request.get_json()
# Example of incoming data structure: {“features”: [feature1, feature2, …]}
features = np.array(data[“features”]).reshape(1, -1)
# Make a prediction using the loaded model
prediction = model.predict(features)
# Return the prediction result in JSON format
return jsonify({“prediction”: prediction[0]})
except Exception as e:
# Handle errors gracefully
return jsonify({“error”: str(e)}), 400
Explanation of Code:
- Route: The route /predict is defined to listen for POST requests. This is common for endpoints that take input data and return a response.
- Input Data: The data is expected to come in the form of a JSON request with a key features containing the input features as an array.
- Prediction: The model makes a prediction using the features received in the request.
Error Handling: If something goes wrong (e.g., missing or invalid data), a custom error message is returned.
Step 4: Running the Flask App
if __name__ == “__main__”:
app.run(debug=True)
This runs the Flask development server in debug mode so that any errors can be seen in real-time while you’re developing.
How to Send Data to the /predict Endpoint
Once the Flask app is running, you can test the /predict endpoint by sending a POST request with input data. Here’s an example using curl and Python’s requests library.
Using curl to Send Data
curl -X POST -H “Content-Type: application/json” \
-d ‘{“features”: [5.1, 3.5, 1.4, 0.2]}’
http://127.0.0.1:5000/predict
- -X POST: Indicates the type of request (POST).
- -H “Content-Type: application/json”: Specifies that the request body contains JSON data.
- -d ‘{“features”: [5.1, 3.5, 1.4, 0.2]}’: The data sent in the request.
Using Python’s requests library
import requests
url = “http://127.0.0.1:5000/predict”
data = {“features”: [5.1, 3.5, 1.4, 0.2]}
response = requests.post(url, json=data)
print(“Prediction:”, response.json())
Expected Response
If the prediction is successful, the server will return a JSON response with the prediction:
{
“prediction”: 0
}
If there is an error (e.g., missing or malformed data), the response might look like this:
json
Copy code
{
“error”: “ValueError: could not convert string to float: ‘invalid_data'”
}
Best Practices for Building Prediction APIs
- Input Validation: Always check that the input data conforms to the expected structure before passing it to the model. This can prevent issues related to missing or malformed data.
- Error Handling: Implement robust error handling to deal with unexpected scenarios, such as missing input features or invalid data types.
- Security: Consider implementing authentication and rate-limiting mechanisms to protect your API from unauthorized access and excessive requests.
- Testing: Test your endpoint thoroughly to ensure it works with both valid and invalid inputs. Use tools like Postman to test API endpoints easily.
- Model Versioning: For better version control, you can set up different endpoints for different versions of the model, like /v1/predict and /v2/predict.
Frequently Asked Questions
- Q: Can I make predictions on multiple inputs at once?
A: Yes, you can modify the /predict endpoint to accept an array of feature sets and return multiple predictions. Just adjust the input handling accordingly. - Q: Can I deploy this API to the cloud?
A: Yes, once your Flask app is ready, you can deploy it on platforms like Heroku, AWS, or Google Cloud. These platforms provide services to host web applications and APIs. - Q: How do I secure my API?
A: You can secure your Flask API using authentication methods like API keys or OAuth tokens. Additionally, consider using HTTPS for secure communication. - Q: What is the best way to handle large requests?
A: For large datasets, consider using batch processing or streaming responses. Flask can handle requests with large bodies, but you may need to optimize memory usage and timeout settings. - Q: Can I add multiple prediction endpoints for different models?
A: Yes, you can define multiple routes (e.g., /predict_house_price, /predict_iris) and associate them with different models. Each route can load and serve predictions from its respective model.
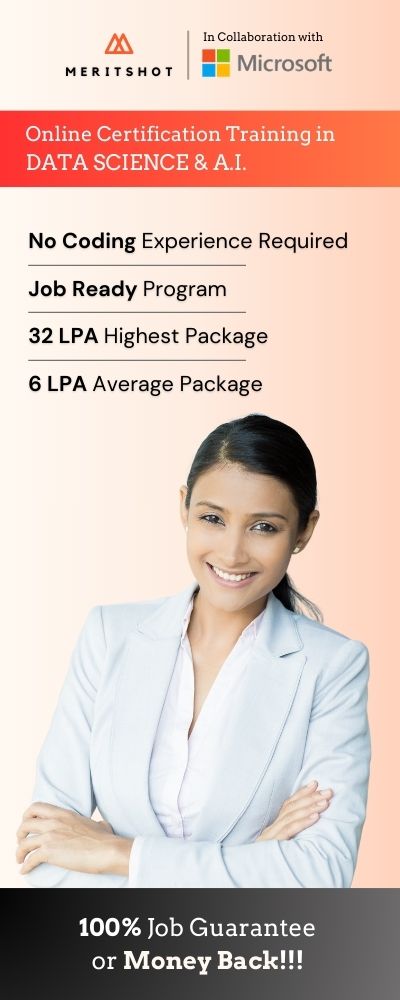