Meritshot Tutorials
- Home
- »
- Preparing Machine Learning Models for Deployment
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Serving JSON ResponsesServing JSON Responses
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Preparing Machine Learning Models for Deployment
Training a Simple Machine Learning Model
Before deploying a machine learning model with Flask, the model must be trained and tested in a separate Python environment. This section demonstrates how to train a simple machine learning model, which will later be saved and integrated into the Flask application.
Example: Predicting House Prices
Dataset
For this example, we’ll use the California Housing dataset available in the sklearn.datasets module. The goal is to predict house prices based on features like the number of rooms, population, and location.
Step 1: Import Required Libraries
Start by importing the necessary libraries for data processing and model training:
import numpy as np
import pandas as pd
from sklearn.datasets import fetch_california_housing
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
Step 2: Load the Dataset
We will load the California Housing dataset and inspect the features and target variable:
# Load the dataset
data = fetch_california_housing(as_frame=True)
df = data.frame
# Display the first few rows of the dataset
print(df.head())
Key Columns:
- MedInc: Median income of the block.
- HouseAge: Median age of the houses.
- AveRooms: Average number of rooms per household.
- AveOccup: Average household size.
- MedHouseVal: Median house value (target variable).
Step 3: Preprocess the Data
Split the dataset into training and testing sets, and separate the features (X) and target variable (y):
# Features and target
X = df.drop(columns=[“MedHouseVal”])
y = df[“MedHouseVal”]
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Step 4: Train the Machine Learning Model
We will use a simple Linear Regression model to predict house prices:
# Initialize the model
model = LinearRegression()
# Train the model
model.fit(X_train, y_train)
# Evaluate the model on the test data
y_pred = model.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print(f”Mean Squared Error: {mse}”)
Step 5: Test the Model
Test the model with sample input data to verify its predictions:
# Sample input data (Average values for simplicity)
sample_input = np.array([[3.87, 29.0, 6.9841, 1.0238, 3.1400, 37.88, -121.23]])
sample_prediction = model.predict(sample_input)
print(f”Predicted House Price: ${sample_prediction[0] * 1000:.2f}”)
Output Example
- Mean Squared Error (MSE): A metric indicating the model’s error on the test data.
- Predicted House Price: An estimated price for the input features.
Sample output for the above example:
Mean Squared Error: 0.52
Predicted House Price: $245,000.00
Next Steps
Once the model is trained and tested, the next steps involve:
- Saving the trained model using pickle or joblib (covered in Section 3.2).
- Loading the saved model into a Python script (covered in Section 3.3).
Frequently Asked Questions
- Q: Why use Linear Regression for this example?
A: Linear Regression is a simple algorithm and easy to implement for beginners. It’s suitable for understanding the deployment process before using complex models. - Q: Can I use a different dataset?
A: Absolutely! You can use any dataset relevant to your project, such as Iris, Titanic, or a custom dataset. - Q: How do I know my model is performing well?
A: Evaluate the model using metrics like Mean Squared Error (MSE), R² Score, or accuracy (for classification tasks). - Q: Can I deploy more complex models like Neural Networks?
A: Yes, you can deploy any model, including those built with TensorFlow, PyTorch, or Keras. The process remains the same. - Q: Should I scale the data before training?
A: Yes, scaling data (e.g., using StandardScaler or MinMaxScaler) improves model performance, especially for algorithms sensitive to feature magnitudes.
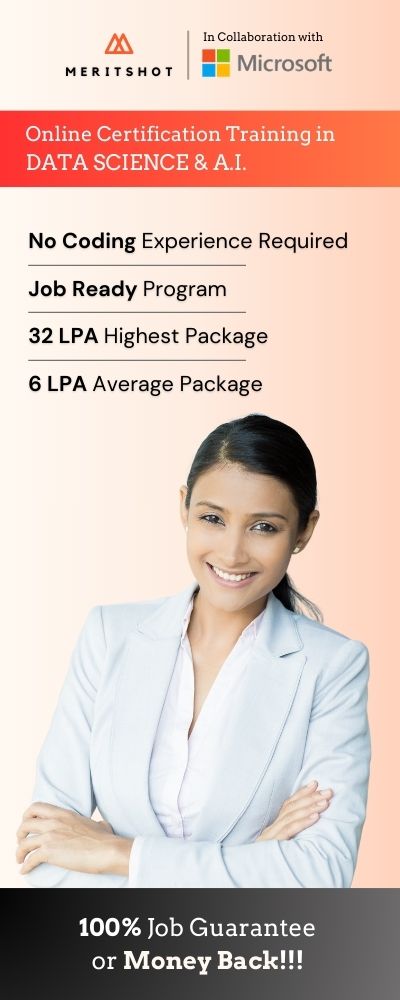