Meritshot Tutorials
- Home
- »
- Running the Development Server
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
Running the Development Server
Once you’ve written some code for your Flask application, the next step is to run the development server. Flask provides a built-in server for testing and debugging during development. This section will guide you through starting and managing the Flask development server.
Steps to Run the Development Server
- Navigate to Your Project Directory
Open your terminal or command prompt and navigate to the directory where your Flask application file (app.py) is located. Use the cd command:
cd /path/to/your/project
- Set the Flask Application
Before starting the development server, Flask needs to know which file contains your application. You can set the environment variable FLASK_APP to your application file.
For Windows (Command Prompt):
set FLASK_APP=app.py
For Windows (PowerShell):
$env:FLASK_APP = “app.py”
For macOS/Linux:
export FLASK_APP=app.py
- Enable Debug Mode (Optional)
To enable debug mode for automatic reloading and error debugging, set the FLASK_ENV variable to development.
For Windows (Command Prompt):
set FLASK_ENV=development
For Windows (PowerShell):
$env:FLASK_ENV = “development”
For macOS/Linux:
export FLASK_ENV=development
- Run the Flask Server
Start the Flask development server using the following command:
flask run
You should see output similar to the following:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
* Debug mode: on
Accessing Your Application
- Open your web browser.
- Navigate to http://127.0.0.1:5000 or http://localhost:5000 to view your application.
Customizing the Server
You can specify the host and port when running the server:
flask run –host=0.0.0.0 –port=8080
- Host: 0.0.0.0 makes the app accessible on your local network. By default, it’s 127.0.0.1 (localhost).
- Port: Specify any port number (default is 5000).
Example Output
Suppose your app.py contains the following:
from flask import Flask
app = Flask(__name__)
@app.route(“/”)
def home():
return “Welcome to Flask!”
When you run flask run and navigate to http://127.0.0.1:5000, you will see:
Welcome to Flask!
Stopping the Development Server
To stop the server, go to your terminal and press CTRL+C.
Common Errors and Fixes
- Error: “ModuleNotFoundError: No module named ‘flask'”
- Cause: Flask is not installed in your current environment.
- Fix: Install Flask using:
pip install flask
- Error: “The term ‘flask’ is not recognized as the name of a cmdlet”
- Cause: Python or Flask is not added to your PATH.
- Fix: Ensure Python and Flask are installed correctly and added to your system PATH.
- Error: “Address already in use”
- Cause: The default port 5000 is already occupied.
- Fix: Run the server on a different port:
flask run –port=8080
Frequently Asked Questions
- Q: Can I use a different filename instead of app.py?
A: Yes, you can use any filename, but you must specify it with the FLASK_APP environment variable.
Example:
set FLASK_APP=myfile.py
- Q: What does debug mode do?
A: Debug mode automatically reloads the server when you make changes to your code and displays detailed error messages in the browser. - Q: How do I stop the server from the terminal?
A: Press CTRL+C to terminate the Flask server. - Q: Can I access the server on another device?
A: Yes, by setting the host to 0.0.0.0 when running the server:
flask run –host=0.0.0.0
- Q: How can I run the server in the background?
A: Use a process manager like nohup or screen on Linux, or configure your Flask app for production deployment.
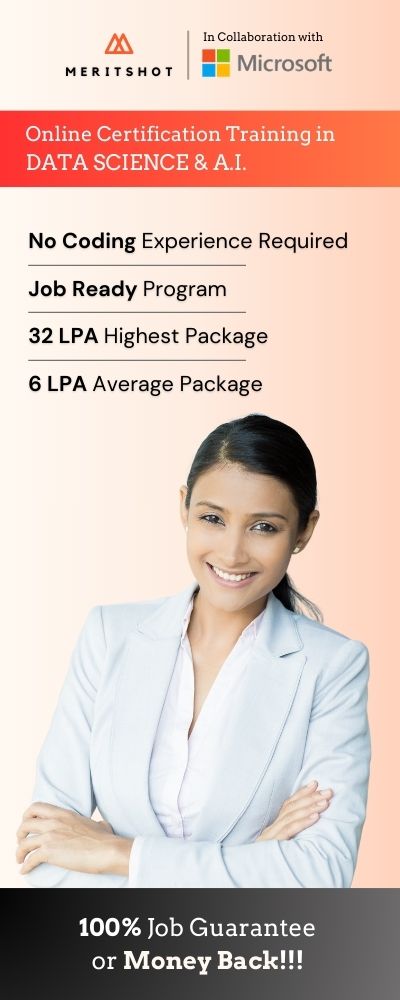