Meritshot Tutorials
- Home
- »
- Flask Application Structure
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Serving JSON ResponsesServing JSON Responses
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Flask Application Structure
As your Flask application grows, maintaining a clean and organized structure becomes essential. A well-organized application structure improves readability, scalability, and ease of debugging. This section outlines the typical structure of a Flask application and explains its key components.
Basic Flask Application Structure
For small projects, the structure can be simple and flat:
flask_app/
│
├── app.py # Main application file
├── templates/ # HTML templates (for rendering frontend)
├── static/ # Static files (CSS, JavaScript, images)
│ ├── css/
│ ├── js/
│ └── images/
└── README.md # Optional: Project description or instructions
Key Files and Directories:
- app.py:
- The main file where the Flask app is created, routes are defined, and the application logic resides.
- templates/:
- A folder for storing HTML files used by Flask’s rendering engine.
- Example: templates/index.html
- static/:
- Contains static resources such as CSS files, JavaScript files, and images.
- Example: static/css/style.css
Organized Flask Application Structure
For larger projects, splitting the application into modules makes the codebase easier to manage. Here’s a common structure:
flask_project/
│
├── app/
│ ├── __init__.py # Initializes the Flask app
│ ├── routes.py # Defines application routes
│ ├── models.py # Database models (if using SQLAlchemy)
│ ├── forms.py # Form logic (if using Flask-WTF)
│ ├── static/ # Static resources (CSS, JS, images)
│ │ ├── css/
│ │ ├── js/
│ │ └── images/
│ └── templates/ # HTML templates
│ ├── base.html # Base template for other pages
│ └── index.html # Homepage template
│
├── config.py # Configuration settings (e.g., database, app keys)
├── run.py # Entry point to run the application
├── requirements.txt # List of Python dependencies
└── README.md # Project documentation
Key Components:
1.app/ Directory:
- __init__.py: Initializes the Flask app and sets up extensions like SQLAlchemy or Flask-Migrate.
- routes.py: Contains all route definitions.
- models.py: Defines database models if a database is used.
- forms.py: Handles forms if using Flask-WTF.
2. config.py:
- Centralized location for app configurations such as database URIs, debug settings, and secret keys.
Example:
class Config:
SECRET_KEY = ‘your_secret_key’
SQLALCHEMY_DATABASE_URI = ‘sqlite:///site.db’
DEBUG = True
3. run.py:
- The entry point to start the application.
Example:
from app import create_app
app = create_app()
if __name__ == “__main__”:
app.run()
4. requirements.txt:
- Contains a list of dependencies for the project, which can be installed using:
pip install -r requirements.txt
5. README.md:
- Provides project details, setup instructions, and usage information.
Benefits of a Modular Structure
1. app/__init__.py:
from flask import Flask
def create_app():
app = Flask(__name__)
app.config[‘SECRET_KEY’] = ‘your_secret_key’
# Import and register blueprints
from .routes import main
app.register_blueprint(main)
return app
2. app/routes.py:
from flask import Blueprint, render_template
main = Blueprint(‘main’, __name__)
@main.route(‘/’)
def home():
return render_template(‘index.html’)
@main.route(‘/about’)
def about():
return “About Page”
3. app/templates/index.html:
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Home</title>
</head>
<body>
<h1>Welcome to My Flask App!</h1>
<p>This is the homepage.</p>
</body>
</html>
4. run.py:
from app import create_app
app = create_app()
if __name__ == “__main__”:
app.run(debug=True)
Benefits of a Modular Structure
- Improved Readability: Separating logic into different files makes the codebase easier to navigate.
- Scalability: Easier to add new features and expand the application.
- Collaboration: Different components (e.g., routes, templates, and models) can be handled by different team members.
- Reusability: Common components (e.g., templates or static files) can be reused across multiple routes.
Frequently Asked Questions
- Q: Do I need a complex structure for every Flask app?
A: No, for small projects, a simple structure with just app.py, templates/, and static/ is sufficient. For larger projects, use a modular structure. - Q: What is the purpose of __init__.py?
A: This file makes the app/ directory a Python package and is used to initialize the Flask app and extensions. - Q: Why use a virtual environment?
A: A virtual environment isolates your project dependencies, ensuring they don’t conflict with other projects on your system. - Q: What is a Blueprint in Flask?
A: Blueprints allow you to organize routes, views, and static files into modular components for better project management. - Q: How do I deploy an app with this structure?
A: After testing locally, you can deploy the app using platforms like Heroku, AWS, or Google Cloud by providing a WSGI entry point, such as run.py.
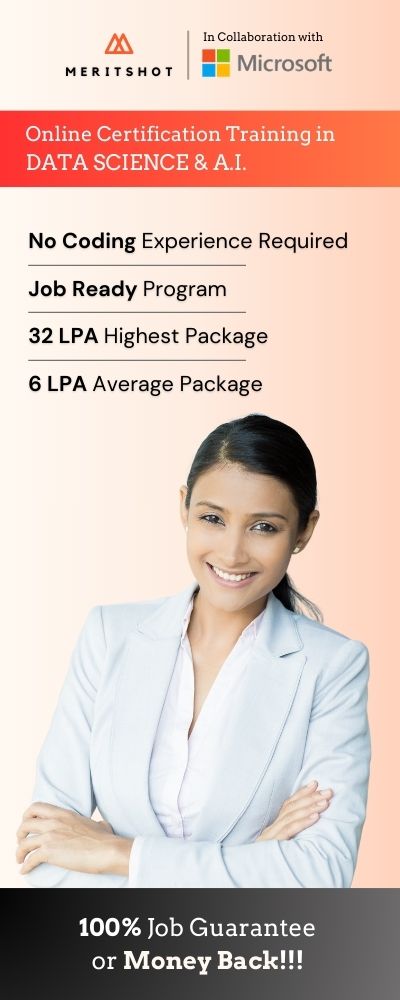