Meritshot Tutorials
- Home
- »
- Loops in R-programming
R Tutorial
-
R-OverviewR-Overview
-
R Basic SyntaxR Basic Syntax
-
R Data TypesR Data Types
-
R-Data StructuresR-Data Structures
-
R-VariablesR-Variables
-
R-OperatorsR-Operators
-
R-StringsR-Strings
-
R-FunctionR-Function
-
R-ParametersR-Parameters
-
Arguments in R programmingArguments in R programming
-
R String MethodsR String Methods
-
R-Regular ExpressionsR-Regular Expressions
-
Loops in R-programmingLoops in R-programming
-
R-CSV FILESR-CSV FILES
-
Statistics in-RStatistics in-R
-
Probability in RProbability in R
-
Confidence Interval in RConfidence Interval in R
-
Hypothesis Testing in RHypothesis Testing in R
-
Correlation and Covariance in RCorrelation and Covariance in R
-
Probability Plots and Diagnostics in RProbability Plots and Diagnostics in R
-
Error Matrices in RError Matrices in R
-
Curves in R-Programming LanguageCurves in R-Programming Language
Loops in R-programming
In R, loops are control flow structures that allow you to repeat a block of code multiple times. Loops are useful when you need to perform repetitive tasks, such as iterating over elements of a vector or
list, applying the same function to multiple data points, or automating tasks that involve repetition.
Types of Loops in R
- for Loop – Repeats code a specific number of times or iterates over elements of a
- while Loop – Repeats code as long as a condition is
- repeat Loop – Repeats code indefinitely until a break condition is
1. for Loop
The for loop is used to iterate over a sequence (like a vector, list, or data frame) and execute a block of code for each element in that sequence.
When you know the number of iterations beforehand, or when iterating over elements of a collection (like vectors or lists).
Syntax:
for (variable in sequence) {
# Code to be executed for each element in the sequence
}
Example:
# Iterate over a vector and
print the elements fruits <- c(“apple”, “banana”, “cherry”)
for (fruit in fruits) {
print(paste(“I like”, fruit))
}
Output
“I like apple”
“I like banana” “I like cherry”
2. while Loop
The while loop repeats a block of code as long as a specified condition is TRUE. It’s useful when you don’t know how many times the loop will run beforehand, but you know the condition for it to continue.
When the number of iterations is not fixed, but based on a condition.
Syntax:
while (condition) {
# Code to be executed while the condition is TRUE
}
Example:
# Print numbers from 1 to 5 using a while loop
i <- 1
while (i <= 5) {
print(i)
i <- i + 1 # Increment the value of i
}
Output
1
2
3
4
5
3. repeat Loop
The repeat loop will execute indefinitely until a break statement is encountered. It’s useful when you want a loop to run without knowing the exact number of iterations, but with a condition to stop it.
Syntax:
repeat {
# Code to be executed if (condition) { break
# Exit the loop if the condition is met } }
Example:
# Using repeat to loop until a condition is met
i <- 1
repeat {
print(i)
i <- i + 1
if (i > 5) {
break # Exit the loop when i is greater than 5
}
}
Output:
1
2
3
4
5
JUMP Statements
In R, jump statements are used to control the flow of loops by either terminating the loop early or skipping certain iterations. There are two key jump statements in R loops:
- break: Terminates the loop
- next: Skips the current iteration and moves to the next
Let us discuss these jump statements one-by-one:
- break Statement
The break statement is used to exit a loop immediately, regardless of the current iteration or condition. When R encounters a break, the loop terminates, and execution moves to the first line of code outside the loop.
Syntax:
Break
Example: Exiting a Loop Using break
# Exiting the loop when i reaches 3
for (i in 1:5) {
if (i == 3) {
break # Exit the loop when i equals 3
}
print(i) }
Output:
1
2
In this example, the loop terminates when i equals 3, so the numbers 1 and 2 are printed, and the loop ends.
2. next Statement
The next statement is used to skip the current iteration of a loop and move to the next one. This means that the code following the next statement within the loop is not executed for that iteration.
Syntax:
Next
Example:
#Skipping Even Numbers Using next
# Skipping even numbers in the loop
for (i in 1:5) {
if (i %% 2 == 0) {
next # Skip even numbers
}
print(i) # Only odd numbers are printed
}
Output:
1
3
5
Combining break and next in Loops
You can use both break and next within the same loop to control the flow more precisely.
Example: Skipping Odd Numbers and Stopping at 4
# Skipping odd numbers and stopping the loop when i equals 4
for (i in 1:6) {
if (i %% 2 != 0) {
next # Skip odd numbers
}
print(i) # Print even numbers
if (i == 4) {
break # Exit the loop when i equals 4
}
}
Output:
2
4
Here, the loop skips the odd numbers, prints 2, skips 3, prints 4, and then terminates the loop due to the break statement.
These jump statements give you control over how loops behave and can help make your code more efficient by avoiding unnecessary iterations or by terminating loops early when certain conditions are met.
Looping Over Different Data Structures
In R, you can use loops to iterate over different data structures such as vectors, lists, matrices,
and data frames. Depending on the data structure, the loop’s behavior may change slightly, but the general concepts of looping remain the same.
1. Looping Over Vectors
Vectors are the most basic data structure in R, and looping through them is straightforward using a for loop.
Example: Looping Over a Numeric Vector
# Numeric vector
numbers <- c(10, 20, 30, 40, 50)
# Looping through the vector
for (num in numbers) {
print(num)
}
Output:
10
20
30
40
50
Example: Looping Over a Character Vector
# Character vector
fruits <- c(“apple”, “banana”, “cherry”)
# Looping through the vector
for (fruit in fruits) {
print(paste(“I like”, fruit))
}
Output:
“I like apple”
“I like banana” “I like cherry”
2. Looping Over Lists
Lists can hold different types of data (including vectors, matrices, other lists, etc.), and you can loop through each element in a list.
Example: Looping Over a List
# List with different data types
my_list <- list(name = “Aaditya”, age = 22, marks = c(95, 88, 92))
# Looping through the list
for (item in my_list) { print(item)
}
Output:
“Aaditya” 22
95 88 92
Example: Accessing List Elements by Name
# Looping through the list by element names
for (name in names(my_list)) {
print(paste(name, “:”, my_list[[name]]))
}
Output:
“name : Aaditya” “age : 22”
“marks : 95 88 92”
3. Looping Over Matrices
Matrices are two-dimensional arrays where each element is of the same data type. You can loop over them by rows, columns, or elements.
Example: Looping Over Elements in a Matrix
# 3×3 Matrix
matrix_data <- matrix(1:9, nrow = 3, ncol = 3)
# Looping over elements in the matrix
for (i in 1:nrow(matrix_data)) {
for (j in 1:ncol(matrix_data)) {
print(paste(“Element at [“, i, “,”, j, “] is”, matrix_data[i, j]))
}
Output:
“Element at [ 1 , 1 ] is 1”
“Element at [ 1 , 2 ] is 4”
“Element at [ 1 , 3 ] is 7”
“Element at [ 2 , 1 ] is 2”
“Element at [ 2 , 2 ] is 5”
“Element at [ 2 , 3 ] is 8”
“Element at [ 3 , 1 ] is 3”
“Element at [ 3 , 2 ] is 6”
“Element at [ 3 , 3 ] is 9”
4. Looping Over Data Frames
Data frames are tabular structures similar to matrices, but each column can contain different types of data. You can loop through rows, columns, or elements of a data frame.
Example: Looping Over Rows in a Data Frame
# Data frame
df <- data.frame(Name = c(“Aaditya”, “Raj”, “Pandey”),
Age = c(22, 24, 23),
Score = c(90, 85, 88))
# Looping over rows
for (i in 1:nrow(df)) {
print(paste(df$Name[i], “is”, df$Age[i], “years old with score”, df$Score[i]))
}
Output:
“Aaditya is 22 years old with score 90” “Raj is 24 years old with score 85”
“Pandey is 23 years old with score 88”
Example: Looping Over Columns in a Data Frame
# Looping over columns
for (col in names(df)) {
print(paste(“Column:”, col))
print(df[[col]])
}
Output:
“Column: Name”
“Aaditya” “Raj” “Pandey” “Column: Age”
22 24 23
“Column: Score” 90 85 88
5. Looping Over a List of Data Frames
You may have multiple data frames stored in a list, and you can loop through each one for processing.
Example: Looping Through a List of Data Frames
# Creating a list of data frames
df1 <- data.frame(ID = 1:3, Score = c(88, 92, 95))
df2 <- data.frame(ID = 4:6, Score = c(81, 85, 89))
df_list <- list(df1, df2)
# Looping through each data frame in the list
for (df in df_list) {
print(df)
}
Output:
ID Score
1 1 88
2 2 92
3 3 95
ID Score
1 4 81
2 5 85
3 6 89
These examples show how to effectively loop over different data structures in R, allowing you to automate and process complex data manipulations.
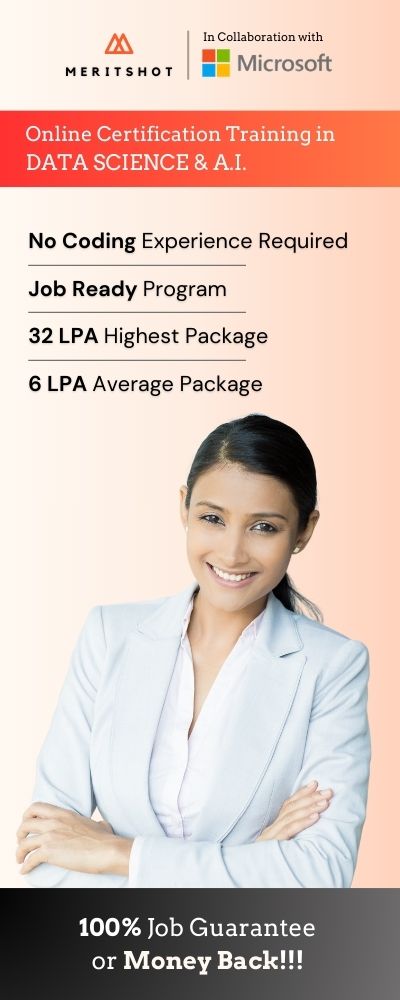