Meritshot Tutorials
- Home
- »
- Arguments in R programming
R Tutorial
-
R-OverviewR-Overview
-
R Basic SyntaxR Basic Syntax
-
R Data TypesR Data Types
-
R-Data StructuresR-Data Structures
-
R-VariablesR-Variables
-
R-OperatorsR-Operators
-
R-StringsR-Strings
-
R-FunctionR-Function
-
R-ParametersR-Parameters
-
Arguments in R programmingArguments in R programming
-
R String MethodsR String Methods
-
R-Regular ExpressionsR-Regular Expressions
-
Loops in R-programmingLoops in R-programming
Arguments in R programming
In R, arguments are values that you pass to a function to specify how it should perform its task. A function may take zero or more arguments depending on its definition. These
arguments help control the behavior of the function and determine what kind of output is produced.
Arguments are placed inside the parentheses following the function name when calling a function. The simplest way to understand this is through an
Example:
# Defining a function with arguments
greet <- function(name) {
paste(“Hello,”, name)
}
# Calling the function with an argument
greet(“Aaditya”) # Output: “Hello, Aaditya”
Here, name is an argument of the function greet. When you call greet(“Aaditya”), the argument “Aaditya” is passed to the function and used inside its body.
2. Adding Arguments in R
When defining a function in R, you can add arguments by specifying them inside the parentheses after the function name. These arguments can have default values or can be mandatory.
Example 1: Simple Function with Arguments
# Defining a function with two arguments
add_numbers <- function(x, y) {
return(x + y)
}
# Calling the function with arguments
add_numbers(3, 5) # Output: 8
In this example, the function add_numbers() takes two arguments, x and y. When you call add_numbers(3, 5), it adds the values and returns the result.
Example 2: Function with Default Arguments
# Function with a default argument
add_numbers <- function(x, y = 10) {
return(x + y)
}
# Calling the function with and without the second argument
add_numbers(5) # Output: 15 (uses the default value of 10 for ‘y’)
add_numbers(5, 20) # Output: 25 (overrides the default value of ‘y’)
Here, the argument y has a default value of 10. If you don’t provide a value for y, the function uses the default value.
2. Adding Multiple Arguments in R (Dots … Argument)
In R, you can use the ellipsis (…) to allow a function to accept an arbitrary number of arguments. This is useful when you don’t know in advance how many arguments will be passed to the function.
Example: Using ... to Accept Multiple Arguments
# Function with ellipsis to accept multiple arguments
summarize_numbers <- function(…, digits = 2) {
numbers <- c(…)
return(round(mean(numbers), digits))
}
# Calling the function with varying numbers of arguments
summarize_numbers(1, 2, 3, 4, 5) # Output: 3.00 (mean of 1, 2, 3, 4, 5)
summarize_numbers(10, 20, 30) # Output: 20.00 (mean of 10, 20, 30)
In this example, the function summarize_numbers() can take any number of numeric
arguments using the … (dots) argument. Inside the function, c(…) combines all arguments into a vector, and the mean of the numbers is computed.
Key Points about the Dots (…) Argument:
- It allows you to pass an arbitrary number of
- Useful for functions that wrap around other functions, or when the number of arguments is flexible.
Example: Forwarding Extra Arguments
The … argument is also useful for passing extra arguments to other functions.
# Function that forwards extra arguments to another function
plot_with_style <- function(x, y, …) {
plot(x, y, col = “blue”, pch = 19, …)
}
# Passing additional graphical parameters through `…`
plot_with_style(1:10, 1:10, main = “Plot Title”, xlab = “X-Axis”, ylab = “Y-Axis”)
In this example, the … argument allows you to pass additional parameters (like main, xlab, and ylab) to the plot() function without explicitly defining them in
the plot_with_style() function.
2. Using Functions as Arguments in R
In R, functions can be treated as first-class objects, meaning that you can pass functions as arguments to other functions. This enables you to apply one function inside another, or to create more flexible and reusable functions.
Example: Passing a Function as an Argument
# Defining a function that takes another function as an argument
apply_function <- function(x, func) {
return(func(x))
}
# Defining some functions to use as arguments
square <- function(x) { x^2 }
cube <- function(x) { x^3 }
# Passing functions as arguments
apply_function(4, square) # Output: 16 (4^2)
apply_function(3, cube) # Output: 27 (3^3)
Here, the function apply_function() takes two arguments: x (a number) and func (a function). When you pass square or cube as the second argument, it applies that function to x.
Example: Using Built-in Functions as Arguments
You can also pass built-in R functions like sum(), mean(), or custom-defined functions as arguments to other functions.
# Passing built-in functions as arguments
apply_function(c(1, 2, 3, 4, 5), sum) # Output: 15 (sum of the vector)
apply_function(c(1, 2, 3, 4, 5), mean) # Output: 3 (mean of the vector)
Example: Using Anonymous Functions as Arguments
Sometimes, you may not want to define a full function separately. Instead, you can use anonymous functions (functions without a name) as arguments.
# Using an anonymous function as an argument
apply_function(5, function(x) { x + 10 }) # Output: 15 (adds 10 to 5)
In this example, the function function(x) { x + 10 } is passed directly as an argument without giving it a name.
Summary of Key Concepts:
- Arguments in R are the inputs you pass to functions to control their
- You can add multiple arguments to functions by specifying them in parentheses or using the … (dots) argument to accept a variable number of
- Functions as arguments: Functions in R can take other functions as arguments, allowing for flexible and reusable code structures.
- Default arguments can simplify function usage by providing fallback values if certain arguments are not
By using these techniques, We can create more dynamic, flexible, and reusable functions in R.
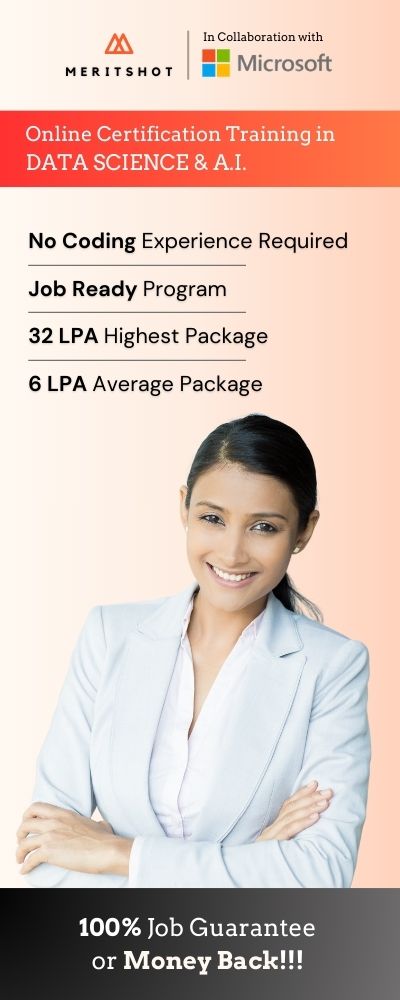