Meritshot Tutorials
- Home
- »
- R Basic Syntax
R Tutorial
-
R-OverviewR-Overview
-
R Basic SyntaxR Basic Syntax
-
R Data TypesR Data Types
-
R-Data StructuresR-Data Structures
-
R-VariablesR-Variables
-
R-OperatorsR-Operators
-
R-StringsR-Strings
-
R-FunctionR-Function
-
R-ParametersR-Parameters
-
Arguments in R programmingArguments in R programming
-
R String MethodsR String Methods
-
R-Regular ExpressionsR-Regular Expressions
-
Loops in R-programmingLoops in R-programming
-
R-CSV FILESR-CSV FILES
-
Statistics in-RStatistics in-R
-
Probability in RProbability in R
-
Confidence Interval in RConfidence Interval in R
-
Hypothesis Testing in RHypothesis Testing in R
-
Correlation and Covariance in RCorrelation and Covariance in R
-
Probability Plots and Diagnostics in RProbability Plots and Diagnostics in R
-
Error Matrices in RError Matrices in R
-
Curves in R-Programming LanguageCurves in R-Programming Language
R Basic Syntax
As a convention, we will start learning R programming by writing a “Hello, World!” program. We will be writing programs of R on the command prompt or you can use an R script file to write your program. Let’s check , How the Syntax of R works ?
The basic syntax in R involves expressions, variables, functions, and commands, comments that are executed in the R environment or at the command prompt.
R Command Prompt
Once you have R environment setup, then it’s easy to start your R command prompt by just typing the following command at your command prompt −
$ R
This will launch R interpreter and you will get a prompt > where you can start typing your program as follows −
$ R
This will launch R interpreter and you will get a prompt > where you can start typing your program as follows −
myString <- “Hello, Meritshot!” print (myString)
OUTPUT:
“Hello,Meritshot!”
OR
#My First program in R programming string <-“Hello Meritshot!” print(string)
Here first statement defines a string variable myString, where we assign a string “Hello, Meritshot!” and then next statement print() is being used to print the value stored in variable myString.
Usually, you will do your programming by writing your programs in script files and then you execute those scripts at your command prompt with the help of R interpreter called Rscript.
# Printing the sum of 3 and 4
print(3 + 4)
#Output: 7
# Assigning value 10 to variable x
x <- 10
Print(x) 10
Comments
Comments can be used to explain R code, and to make it more readable. It can also be used to prevent execution when testing alternative code.
Comments starts with a #. When executing code, R will ignore anything that starts with #.
Single Line Comments
This example uses a comment before a line of code:
1. Example
# This is a comment
“Hello Meritshot!”
This example uses a comment at the end of a line of code:
2. Example
“Hello Meritshot!” # This is a comment
Comments does not have to be text to explain the code, it can also be used to prevent R from executing the code:
Example
# “Good morning!”
“Good night!”
Multiline Comments
Unlike other programming languages, such as Java, there are no syntax in R for multiline comments. However, we can just insert a # for each line to create multiline comments
Example
# We are in a Team
# R Tutorials
# “Hello Roshan Sharma”
# More than just one line
Here Are Some Examples of Basic R-Syntax and Comments, How to Use these,
Example 1: Assigning a Value to a Variable
# Allocating the number of apples to the variable ‘num_apples’
num_apples <- 5
print(num_apples)
# Output: 5
In this example, we assign the value 5 to the variable num_apples, representing the number of apples you have. This is a relatable scenario for anyone keeping track of their fruit inventory!
Example 2: Basic Arithmetic Operation
# Assigning the number of apples and bananas
num_apples <- 5
num_bananas <- 4
#Adding the number of apples and bananas
total_fruits <- num_apples + num_bananas
print(total_fruits) # Output: 9
In this example, we assign values to the number of apples and bananas, then calculate the total number of fruits by adding them together. This is a relatable scenario for anyone keeping track of their fruit collection!
Example 3: Creating a Vector
# Creating a vector with different types of fruits
fruits <- c(“Apple”, “Banana”, “Cherry”)
print(fruits) # Output: “Apple” “Banana” “Cherry”
In this example, we create a vector called fruits that contains the names of three different fruits. This is a common scenario when listing your favorite fruits!
Example 4: Accessing an Element in a Vector
We get syntax error because Python does not allow kebab case
# Creating a vector of fruits
fruits <- c(“Apple”, “Banana”, “Cherry”)
# Accessing the second fruit in the vector second_fruit <- fruits[2]
print(second_fruit) # Output: “Banana”
# Creating a vector of fruits
fruits <- c(“Apple”, “Banana”, “Cherry”)
# Accessing the second fruit in the vector second_fruit <- fruits[2]
print(second_fruit) # Output: “Banana”
Example 5: Simple Conditional Statement
# Assigning the number of fruits you have
num_fruits <- 8
# Checking if you have more than 5
fruits if (num_fruits > 5) {
print(“You have enough fruits!”) # Output: You have enough fruits!
}
Example 6: Simple Function Definition
# Defining a function to count total fruits
count_fruits <- function(apples, bananas) {
return(apples + bananas)
}
# Using the function to count total fruits
total_fruits <- count_fruits(5, 3)
print(total_fruits) # Output: 8
In this example, we define a function called count_fruits that takes the number of apples and bananas as inputs and returns their total. We then use this function to calculate the total number of fruits, which is a practical scenario for anyone managing their fruit inventory!
Example 7: Data Manipulation
# Creating a vector of fruit quantities
fruit_quantities <- c(2, 5, 3, 7, 1)
# Subsetting the vector to get the first three quantities
print(fruit_quantities[1:3]) # Output: 2 5 3
# Filtering the vector to get quantities greater than 3
filtered_quantities <- fruit_quantities[fruit_quantities > 3]
print(filtered_quantities) # Output: 5 7
In this example, we create a vector called fruit_quantities representing the quantities of different fruits. We then subset the vector to get the first three quantities and filter it to find quantities greater than 3. This is a relatable scenario for anyone managing their fruit supplies!
Example 7: Plotting And Visualizations
# Creating a vector of fruit types and their quantities
fruit_types <- c(“Apples”, “Bananas”, “Cherries”, “Dates”)
quantities <- c(5, 3, 8, 2)
# Basic bar plot to visualize the quantities of fruits
barplot(quantities, names.arg = fruit_types, main = “Fruit Quantities”, xlab = “Fruit
Types”, ylab = “Quantity”)
In this example, we create a bar plot to visualize the quantities of different types of fruits. The barplot function displays the quantities of apples, bananas, cherries, and dates, making it easy to compare them visually. This is a practical scenario for anyone interested in tracking and presenting their fruit inventory!
Example 8: Advanced Visualization
# Load the ggplot2 library
library(ggplot2)
# Creating a data frame with fruit types and their prices
fruit_data <- data.frame(
fruit = c(“Apples”, “Bananas”, “Cherries”, “Dates”),
price = c(1.5, 0.5, 2.0, 3.0)
)
# Creating a scatter plot to visualize fruit prices
ggplot(fruit_data, aes(x = fruit, y = price)) +
geom_point(size = 3, color = “blue”)
+ ggtitle(“Fruit Prices”) +
xlab(“Fruit Types”) +
ylab(“Price ($)”)
In this example, we create a scatter plot to visualize the prices of different fruits using the ggplot2 library. Each point represents a fruit type and its corresponding price, making it easy to compare the costs visually. This is a relatable scenario for anyone interested in understanding fruit pricing
These examples cover the examples from basics of R to the advanced level, including variable assignment, simple operations, vectors, conditional statements, and functions, Data Manipulation, Plotting And Visualizations, Advanced Visualization. We will use these concepts of Syntax in further modules
So in Nutshell , R programming makes it easy to carry out simple arithmetic operations and assign values to variables using script files. With the R interpreter, you can quickly perform calculations and handle your data, which really boosts your efficiency as a programmer. This straightforward approach not only helps you get started but also sets the stage for diving into more advanced data analysis and visualization tasks in R. Whether you’re just beginning or looking to expand your skills, R provides a solid foundation for your data journey..
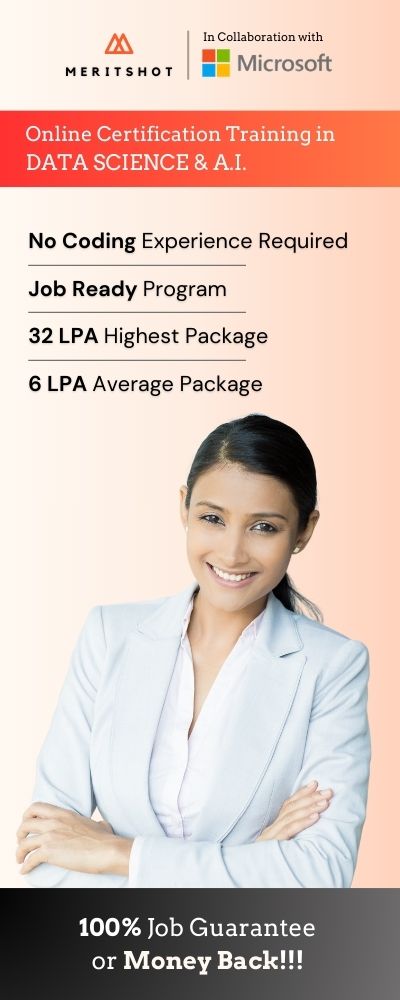