Meritshot Tutorials
- Home
- »
- SQL Comments
SQL Tutorial
-
SQL SyntaxSQL Syntax
-
SQL ORDER BY ClauseSQL ORDER BY Clause
-
Introduction to SQLIntroduction to SQL
-
SQL SELECT DISTINCTSQL SELECT DISTINCT
-
SQL Logical OperatorsSQL Logical Operators
-
SQL WHERE ClauseSQL WHERE Clause
-
SQL SELECT StatementSQL SELECT Statement
-
SQL DELETE StatementSQL DELETE Statement
-
SQL INSERT INTOSQL INSERT INTO
-
SQL Null ValuesSQL Null Values
-
SQL Update StatementSQL Update Statement
-
SQL Select TopSQL Select Top
-
SQL Aggregate FunctionsSQL Aggregate Functions
-
SQL LIKE and WildcardsSQL LIKE and Wildcards
-
SQL IN and SQL BETWEENSQL IN and SQL BETWEEN
-
SQL JOINSSQL JOINS
-
SQL Group BySQL Group By
-
SQL HavingSQL Having
-
SQL EXISTSSQL EXISTS
-
SQL SELECT INTOSQL SELECT INTO
-
SQL INSERT INTO SELECTSQL INSERT INTO SELECT
-
SQL CASE STATEMENTSQL CASE STATEMENT
-
SQL NULL FunctionsSQL NULL Functions
-
SQL Stored ProceduresSQL Stored Procedures
-
SQL User-Defined FunctionsSQL User-Defined Functions
-
SQL CommentsSQL Comments
-
SQL OperatorsSQL Operators
-
SQL Database Creation and ManagementSQL Database Creation and Management
-
SQL CREATE DATABASE StatementSQL CREATE DATABASE Statement
-
SQL CREATE TABLE StatementSQL CREATE TABLE Statement
-
SQL DROP DATABASE StatementSQL DROP DATABASE Statement
-
SQL DROP TABLE StatementSQL DROP TABLE Statement
-
SQL ALTER TABLE StatementSQL ALTER TABLE Statement
-
SQL NOT NULL ConstraintSQL NOT NULL Constraint
-
SQL UNIQUE ConstraintSQL UNIQUE Constraint
-
SQL PRIMARY KEY ConstraintSQL PRIMARY KEY Constraint
-
SQL FOREIGN KEY ConstraintSQL FOREIGN KEY Constraint
-
SQL CHECK ConstraintSQL CHECK Constraint
-
SQL DEFAULT ConstraintSQL DEFAULT Constraint
-
SQL IndexesSQL Indexes
-
SQL Date FunctionsSQL Date Functions
-
SQL ViewsSQL Views
-
SQL InjectionSQL Injection
-
SQL Data Types OverviewSQL Data Types Overview
-
SQL AUTO_INCREMENTSQL AUTO_INCREMENT
-
SQL Keywords ReferenceSQL Keywords Reference
SQL Comments
SQL comments are annotations in SQL code that are ignored during execution but can provide valuable information for developers. They are used to explain and document SQL queries, stored procedures, and other SQL objects, making the code easier to understand and maintain.
Types of SQL Comments
- Single-Line Comments
- Multi-Line Comments
Syntax:
Single-Line Comments:
- Using —
Single-line comments start with — and continue to the end of the line. They are commonly used for brief explanations or notes.
— This is a single-line comment
SELECT * FROM employees ; — Select all columns from employees table
Multi-Line Comments:
- Using /* */
Multi-line comments are enclosed between /* and */. They can span multiple lines and are useful for longer explanations or disabling blocks of code.
/*
This is a multi-line comment.
It can span multiple lines and is used for more extensive explanations.
*/
SELECT * FROM employees;
Example:
1. Single-Line Comment Example
— Retrieve all employee details
SELECT employee_id, first_name, last_name, salary
FROM employees
2. Multi-Line Comment Example
/*
The following query retrieves employee details including their salaries.
It selects the employee_id, first_name, last_name, and salary columns.
*/
SELECT employee_id, first_name, last_name, salary
FROM employees;
3. Combining Single-Line and Multi-Line Comments
— Start of the query
SELECT employee_id, first_name, last_name, salary
FROM employees
/* Filtering employees with a salary greater than 50000 */
WHERE salary > 50000;
4. Disabling Code Using Comments
/*
The following query is temporarily disabled for testing purposes.
Uncomment it when ready to execute.
*/
— SELECT * FROM orders;
Tips to Remember
- Use Comments Wisely: Ensure comments are clear and relevant. Avoid stating the obvious; instead, explain why certain decisions were made or how the code works.
- Keep Comments Up-to-Date: Update comments when modifying code to reflect the current state of the logic and avoid misleading information.
- Avoid Excessive Comments: Over-commenting can clutter the code. Comment only where necessary and where the code’s intent or functionality isn’t immediately obvious.
- Use Consistent Style: Stick to a consistent commenting style throughout your codebase to maintain readability and clarity.
Frequently Asked Questions
Q1: Can comments be nested in SQL?
A1: No, SQL does not support nested comments. Be cautious when using multi-line comments to avoid confusion.
Q2: Are comments visible in SQL query results?
A2: No, comments are ignored during execution and do not appear in query results.
Q3: Can comments be used within SQL stored procedures?
A3: Yes, comments can be used within stored procedures to document and explain the logic.
Example:
sql
Copy code
CREATE PROCEDURE GetEmployeeDetails
AS
BEGIN
— Select employee details for a specific employee
SELECT employee_id, first_name, last_name, salary
FROM employees
WHERE employee_id = 1;
END;
Q4: What is the purpose of commenting out code?
A4: Commenting out code is useful for temporarily disabling code during development or testing without deleting it.
Q5: Can comments be used in SQL scripts executed by tools or applications?
A5: Yes, comments are ignored by SQL tools and applications during execution, making them safe for documentation within scripts.
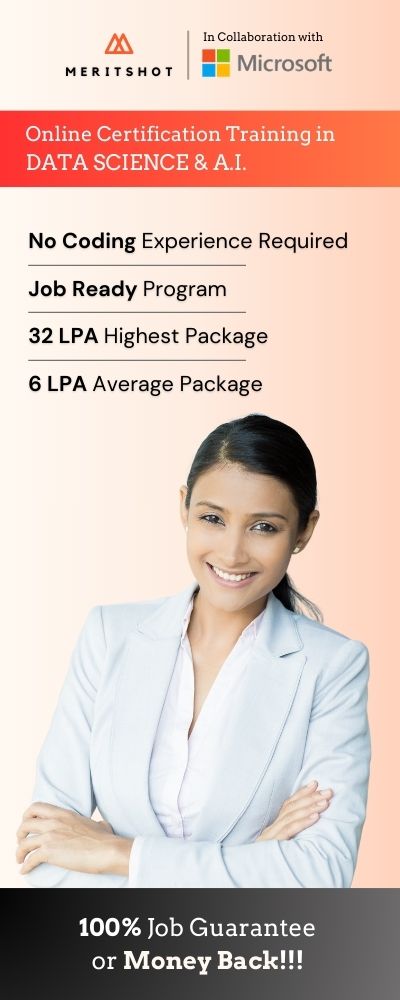