Meritshot Tutorials
- Home
- »
- SQL EXISTS
SQL Tutorial
-
SQL SyntaxSQL Syntax
-
SQL ORDER BY ClauseSQL ORDER BY Clause
-
Introduction to SQLIntroduction to SQL
-
SQL SELECT DISTINCTSQL SELECT DISTINCT
-
SQL Logical OperatorsSQL Logical Operators
-
SQL WHERE ClauseSQL WHERE Clause
-
SQL SELECT StatementSQL SELECT Statement
-
SQL DELETE StatementSQL DELETE Statement
-
SQL INSERT INTOSQL INSERT INTO
-
SQL Null ValuesSQL Null Values
-
SQL Update StatementSQL Update Statement
-
SQL Select TopSQL Select Top
-
SQL Aggregate FunctionsSQL Aggregate Functions
-
SQL LIKE and WildcardsSQL LIKE and Wildcards
-
SQL IN and SQL BETWEENSQL IN and SQL BETWEEN
-
SQL JOINSSQL JOINS
-
SQL Group BySQL Group By
-
SQL HavingSQL Having
-
SQL EXISTSSQL EXISTS
-
SQL SELECT INTOSQL SELECT INTO
-
SQL INSERT INTO SELECTSQL INSERT INTO SELECT
SQL EXISTS
The EXISTS operator in SQL is used to check if a subquery returns any rows. It is a logical operator that returns TRUE if the subquery returns one or more rows and FALSE if it returns no rows. It is often used in conjunction with a subquery to test for the presence of rows in another table.
Purpose
- Check Row Existence: Determines if a subquery returns any results.
- Conditional Filtering: Used to filter rows based on the existence of related data in another table.
Syntax
SELECT column_name(s)
FROM table_name
WHERE EXISTS (subquery);
Example:
1. Find Departments with Employees
This query retrieves departments that have at least one employee.
SELECT department_id, department_name
FROM departments
WHERE EXISTS (
SELECT 1
FROM employees
WHERE employees.department_id = departments.department_id
);
Output:
department_id | department_name |
1 | Sales |
2 | HR |
3 | IT |
2. Get Customers with Orders
This query retrieves customers who have placed at least one order.
SELECT customer_id, customer_name
FROM customers
WHERE EXISTS (
SELECT 1
FROM orders
WHERE orders.customer_id = customers.customer_id
);
Output:
customer_id | customer_name |
1 | Rajesh |
3 | Priya |
3. Find Products with Sales
This query selects products that have been sold at least once.
SELECT product_id, product_name
FROM products
WHERE EXISTS (
SELECT 1
FROM sales
WHERE sales.product_id = products.product_id
);
Output:
product_id | product_name |
101 | Sports Shoe |
102 | Running Shoes |
4. Get Employees with Specific Skills
This query retrieves employees who have skills listed in the skills table.
SELECT employee_id, first_name
FROM employees
WHERE EXISTS (
SELECT 1
FROM skills
WHERE skills.employee_id = employees.employee_id
AND skills.skill_name = ‘SQL’
);
Output:
employee_id | first_name |
1 | Rajesh |
3 | Ravi |
Tips to Remember
- Subquery Returns: EXISTS does not depend on the data returned by the subquery, only whether or not any rows are returned.
- Use with NOT EXISTS: NOT EXISTS can be used to check for the absence of rows returned by a subquery.
- Efficient Checks: EXISTS is often used for its efficiency in checking row existence without returning actual data from the subquery.
- Optimized Queries: EXISTS can help optimize queries, especially when dealing with large datasets or complex subqueries.
Power of EXISTS
- Efficient Filtering: Allows efficient checking for the existence of related data, which can optimize query performance.
- Complex Conditions: Useful in scenarios where you need to filter based on conditions applied to related tables or subqueries.
Frequently Asked Questions
Q1: How does EXISTS differ from IN?
A1: EXISTS checks for the existence of rows returned by a subquery, while IN checks if a value is present in a set of values or the result of a subquery.
Example with IN:
SELECT employee_id
FROM employees
WHERE department_id IN (SELECT department_id FROM departments WHERE department_name = ‘IT’);
Example with EXISTS:
SELECT employee_id
FROM employees e
WHERE EXISTS (
SELECT 1
FROM departments d
WHERE d.department_id = e.department_id
AND d.department_name = ‘IT’
);
Q2: Can EXISTS be used with JOIN?
A2: Yes, EXISTS can be used in combination with JOIN to check for the existence of related rows.
Example:
SELECT e.employee_id, e.first_name
FROM employees e
WHERE EXISTS (
SELECT 1
FROM departments d
WHERE d.department_id = e.department_id
AND d.department_name = ‘Sales’
);
Q3: Can EXISTS be used with multiple subqueries?
A3: Yes, you can use multiple subqueries with EXISTS to check for various conditions.
Example:
SELECT employee_id, first_name
FROM employees e
WHERE EXISTS (
SELECT 1
FROM departments d
WHERE d.department_id = e.department_id
AND EXISTS (
SELECT 1
FROM skills s
WHERE s.employee_id = e.employee_id
AND s.skill_name = ‘Project Management’
)
);
Q4: How does EXISTS handle NULL values?
A4: EXISTS does not return results based on the actual data in the subquery, but simply whether the subquery returns any rows. It handles NULL values naturally within the subquery’s logic.
Q5: Can EXISTS be used with aggregate functions?
A5: Yes, EXISTS can be used with subqueries that include aggregate functions to check for the existence of rows satisfying aggregate conditions.
Example:
SELECT department_id
FROM departments d
WHERE EXISTS (
SELECT 1
FROM employees e
WHERE e.department_id = d.department_id
GROUP BY e.department_id
HAVING COUNT(e.employee_id) > 5
);
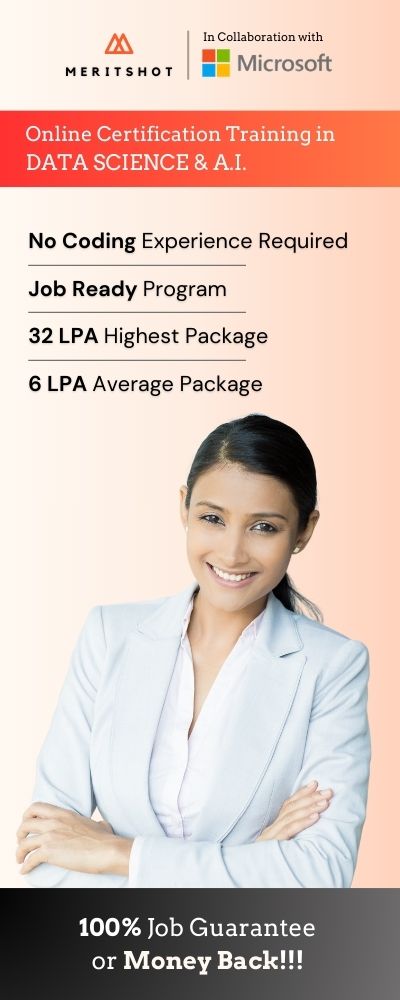