Meritshot Tutorials
- Home
- »
- SQL ORDER BY Clause
SQL Tutorial
-
SQL SyntaxSQL Syntax
-
SQL ORDER BY ClauseSQL ORDER BY Clause
-
Introduction to SQLIntroduction to SQL
-
SQL SELECT DISTINCTSQL SELECT DISTINCT
-
SQL Logical OperatorsSQL Logical Operators
-
SQL WHERE ClauseSQL WHERE Clause
-
SQL SELECT StatementSQL SELECT Statement
-
SQL DELETE StatementSQL DELETE Statement
-
SQL INSERT INTOSQL INSERT INTO
-
SQL Null ValuesSQL Null Values
-
SQL Update StatementSQL Update Statement
-
SQL Select TopSQL Select Top
-
SQL Aggregate FunctionsSQL Aggregate Functions
-
SQL LIKE and WildcardsSQL LIKE and Wildcards
-
SQL IN and SQL BETWEENSQL IN and SQL BETWEEN
-
SQL JOINSSQL JOINS
-
SQL Group BySQL Group By
-
SQL HavingSQL Having
-
SQL EXISTSSQL EXISTS
-
SQL SELECT INTOSQL SELECT INTO
-
SQL INSERT INTO SELECTSQL INSERT INTO SELECT
-
SQL CASE STATEMENTSQL CASE STATEMENT
-
SQL NULL FunctionsSQL NULL Functions
-
SQL Stored ProceduresSQL Stored Procedures
-
SQL User-Defined FunctionsSQL User-Defined Functions
-
SQL CommentsSQL Comments
-
SQL OperatorsSQL Operators
-
SQL Database Creation and ManagementSQL Database Creation and Management
-
SQL CREATE DATABASE StatementSQL CREATE DATABASE Statement
-
SQL CREATE TABLE StatementSQL CREATE TABLE Statement
-
SQL DROP DATABASE StatementSQL DROP DATABASE Statement
-
SQL DROP TABLE StatementSQL DROP TABLE Statement
-
SQL ALTER TABLE StatementSQL ALTER TABLE Statement
-
SQL NOT NULL ConstraintSQL NOT NULL Constraint
-
SQL UNIQUE ConstraintSQL UNIQUE Constraint
-
SQL PRIMARY KEY ConstraintSQL PRIMARY KEY Constraint
-
SQL FOREIGN KEY ConstraintSQL FOREIGN KEY Constraint
-
SQL CHECK ConstraintSQL CHECK Constraint
-
SQL DEFAULT ConstraintSQL DEFAULT Constraint
-
SQL IndexesSQL Indexes
-
SQL Date FunctionsSQL Date Functions
-
SQL ViewsSQL Views
-
SQL InjectionSQL Injection
-
SQL Data Types OverviewSQL Data Types Overview
-
SQL AUTO_INCREMENTSQL AUTO_INCREMENT
-
SQL Keywords ReferenceSQL Keywords Reference
Understanding SQL Syntax
The ORDER BY clause is used in SQL to sort the result set of a query based on one or more columns. Sorting data is essential for organizing your results in a meaningful way, making it easier to analyze and interpret.
Basic Syntax of the ORDER BY Clause
The basic syntax for the ORDER BY clause is:
SELECT column1, column2, …
FROM table_name
ORDER BY column1 [ASC|DESC], column2 [ASC|DESC], …;
- SELECT: Specifies the columns to retrieve.
- FROM: Specifies the table from which to retrieve data.
- ORDER BY: Specifies the columns by which to sort the result set.
- ASC: Sorts the results in ascending order (default).
- DESC: Sorts the results in descending order.
Example:
Of Ordering by a Single Column
Consider a table named employees with columns: employee_id, first_name, last_name, age, and salary. To retrieve a list of employees ordered by their age in ascending order:
SELECT employee_id, first_name, last_name, age
FROM employees
ORDER BY age ASC;
Output:
employee_id | first_name | last_name | age |
4 | Ravi | Kumar | 25 |
1 | Rajesh | Sharma | 30 |
3 | Priya | Patel | 35 |
2 | Aarti | Mehta | 40 |
Example:
Of Ordering by Multiple Columns
To sort employees by department in ascending order and then by salary in descending order:
SELECT employee_id, first_name, last_name, department, salary
FROM employees
ORDER BY department ASC, salary DESC;
Output:
employee_id | first_name | last_name | department | salary |
2 | Aarti | Mehta | Marketing | 60000 |
3 | Priya | Patel | Marketing | 50000 |
1 | Rajesh | Sharma | Sales | 45000 |
4 | Ravi | Kumar | HR | 70000 |
Using ORDER BY with Aggregate Functions
You can use the ORDER BY clause to sort results that involve aggregate functions, such as SUM, AVG, etc. For example, to order departments by the average salary of their employees:
SELECT department, AVG(salary) AS average_salary
FROM employees
GROUP BY department
ORDER BY average_salary DESC;
Output:
department | average_salary |
HR | 70000 |
Marketing | 55000 |
Sales | 45000 |
Sorting with NULL Values
By default, NULL values are sorted as the lowest values in ascending order and as the highest values in descending order. To control the sorting of NULL values explicitly, you can use the NULLS FIRST or NULLS LAST options (supported in some databases like PostgreSQL).
SELECT employee_id, first_name, last_name, salary
FROM employees
ORDER BY salary ASC NULLS LAST;
Tips to Remember
- Default Sorting: If no sort direction is specified, the default is ascending order (ASC).
- Multiple Columns: When sorting by multiple columns, SQL sorts by the first column first, then by the second column, and so on.
- Performance Considerations: Sorting large datasets can be resource-intensive. Ensure that columns used in ORDER BY are indexed if you frequently sort on them.
- Test Sorting: Always check your queries to ensure that sorting is performed as expected, especially when using multiple columns.
Power of Data Organization
The ORDER BY clause is a powerful feature in SQL that helps you:
- Organize Results: Present data in a sorted and structured format.
- Prioritize Information: Highlight the most important or relevant records by sorting them.
- Improve Analysis: Make data analysis easier by arranging data in a logical order.
Frequently Asked Questions
Q1: Can I use ORDER BY with GROUP BY?
A1: Yes, you can use ORDER BY to sort the results after grouping data with GROUP BY. For example, you can sort aggregated results by the aggregated values.
Q2: What if I want to sort in descending order by default?
A2: You can specify DESC after the column name to sort in descending order. For example:
SELECT first_name, last_name
FROM employees
ORDER BY salary DESC;
Q3: Can I use ORDER BY with columns that are calculated in the query?
A3: Yes, you can sort by calculated columns. For instance:
SELECT first_name, salary * 1.1 AS adjusted_salary
FROM employees
ORDER BY adjusted_salary DESC;
Q4: How does sorting affect performance?
A4: Sorting can impact performance, especially with large datasets. Indexes on the sorted columns can help improve performance.
Q5: Can I sort by a column that does not appear in the result set?
A5: Yes, you can sort by columns that are not included in the result set. For example:
SELECT first_name
FROM employees
ORDER BY salary DESC;
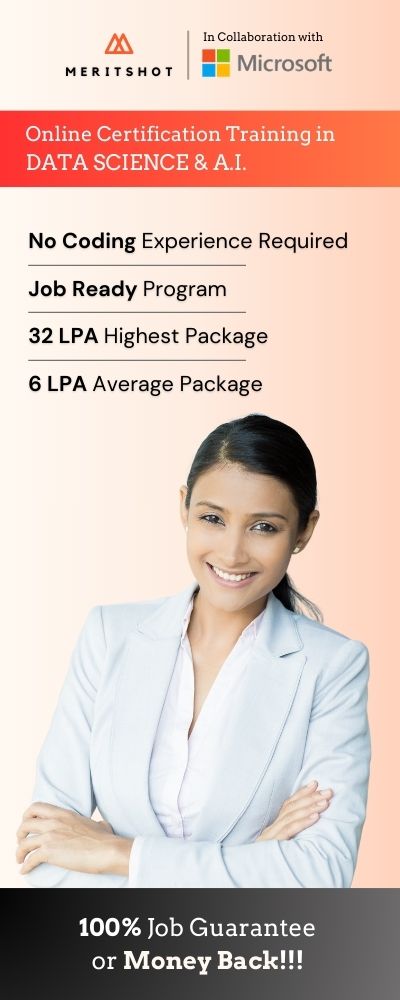